Write a Python program, in a file called forest_map.py, to solve the following problem: Hansel and Gretel are abandoned in a forest with only a bag of breadcrumbs and must find their way home. The forest is laid out in a grid pattern. They can only take a step at a time through the forest, going either South or East. They cannot move diagonally, they cannot move North, and they cannot move West. As they leave a space, they will leave a breadcrumb (“*”) behind just in case -- if they have any breadcrumbs left. They do not leave a breadcrumb in the space where the path ends. If they don’t have enough breadcrumbs, they still move on to the next space, leaving their current space as it was. Given (as input) the number of breadcrumbs Hansel and Gretel have, and given the forest layout (as a fixed, but changeable, two-dimensional list in your code), determine whether Hansel and Gretel have just enough, more than enough, or not enough, breadcrumbs to leave in the path to get to the end. If they have more than enough, display how many breadcrumbs remain. An empty space (‘ ’) in the forest layout denotes a path and an ‘X’ in the forest layout denotes a tree in the forest. Hansel and Gretel cannot move where a tree is. The end of the path is denoted by an ‘E’ in the lower right-hand corner of the forest. Hansel and Gretel always start in the upper left-hand corner of the forest. There is always only one direction that they can move in, they will never have to choose between South and East. Your program must include the following: • function main that contains the forest layout as a list (you can just make it up here, it doesn’t have to be input, but the sizes of the dimensions should be flexible and the starting position always has to be in the top left corner of the list); it asks the user for the number of breadcrumbs as an integer; it should print the resulting forest map showing the breadcrumbs (just print the 2-D list, it doesn’t have to be formatted) and also make use of the following functions to compute and print the message to the user • function distance which takes, as parameters, the forest layout and the initial number of breadcrumbs, and returns the number of breadcrumbs they used to get to the end – this value will be negative if they run out before reaching the end; remember, unknown to Hansel and Gretel but known to you, the path only continues either East or South, and there is only one path and no dead-ends • function crumbsLeft which takes, as a parameter, the number of breadcrumbs left (which may be negative if the path is longer than the number of breadcrumbs) and prints that there were not enough breadcrumbs, just enough breadcrumbs, or the actual amount of breadcrumbs left For example, given the following forest map: forestPath = [[' ',' ',' ','X','X'],\ ['X','X',' ',' ','X'],\ ['X','X','X',' ','X'],\ [' ','X','X',' ',' '],\ [' ','X','X','X',' '],\ [' ',' ','X','X',' '],\ [' ',' ','X','X','E']] If the number of breadcrumbs given is 12, sample output would be: Enter number of breadcrumbs: 12 [['*', '*', '*', 'X', 'X'], ['X', 'X', '*', '*', 'X'], ['X', 'X', 'X', '*', 'X'], [' ', 'X', 'X', '*', '*'], [' ', 'X', 'X', 'X', '*'], [' ', ' ', 'X', 'X', '*'], [' ', ' ', 'X', 'X', 'E']] 2 breadcrumb(s) are left If the number of breadcrumbs given is 5, sample output would be: Enter number of breadcrumbs: 5 [['*', '*', '*', 'X', 'X'], ['X', 'X', '*', '*', 'X'], ['X', 'X', 'X', ' ', 'X'], [' ', 'X', 'X', ' ', ' '], [' ', 'X', 'X', 'X', ' '], [' ', ' ', 'X', 'X', ' '], [' ', ' ', 'X', 'X', 'E']] Not enough breadcrumbs for the trip. If the number of breadcrumbs given is 10, sample output would be: Enter number of breadcrumbs: 10 [['*', '*', '*', 'X', 'X'], ['X', 'X', '*', '*', 'X'], ['X', 'X', 'X', '*', 'X'], [' ', 'X', 'X', '*', '*'], [' ', 'X', 'X', 'X', '*'], [' ', ' ', 'X', 'X', '*'], [' ', ' ', 'X', 'X', 'E']] Phew, just enough breadcrumbs for the trip.
Write a Python program, in a file called forest_map.py, to solve the following problem: Hansel and Gretel are abandoned in a forest with only a bag of breadcrumbs and must find their way home. The forest is laid out in a grid pattern. They can only take a step at a time through the forest, going either South or East. They cannot move diagonally, they cannot move North, and they cannot move West. As they leave a space, they will leave a breadcrumb (“*”) behind just in case -- if they have any breadcrumbs left. They do not leave a breadcrumb in the space where the path ends. If they don’t have enough breadcrumbs, they still move on to the next space, leaving their current space as it was. Given (as input) the number of breadcrumbs Hansel and Gretel have, and given the forest layout (as a fixed, but changeable, two-dimensional list in your code), determine whether Hansel and Gretel have just enough, more than enough, or not enough, breadcrumbs to leave in the path to get to the end. If they have more than enough, display how many breadcrumbs remain. An empty space (‘ ’) in the forest layout denotes a path and an ‘X’ in the forest layout denotes a tree in the forest. Hansel and Gretel cannot move where a tree is. The end of the path is denoted by an ‘E’ in the lower right-hand corner of the forest. Hansel and Gretel always start in the upper left-hand corner of the forest. There is always only one direction that they can move in, they will never have to choose between South and East. Your program must include the following: • function main that contains the forest layout as a list (you can just make it up here, it doesn’t have to be input, but the sizes of the dimensions should be flexible and the starting position always has to be in the top left corner of the list); it asks the user for the number of breadcrumbs as an integer; it should print the resulting forest map showing the breadcrumbs (just print the 2-D list, it doesn’t have to be formatted) and also make use of the following functions to compute and print the message to the user • function distance which takes, as parameters, the forest layout and the initial number of breadcrumbs, and returns the number of breadcrumbs they used to get to the end – this value will be negative if they run out before reaching the end; remember, unknown to Hansel and Gretel but known to you, the path only continues either East or South, and there is only one path and no dead-ends • function crumbsLeft which takes, as a parameter, the number of breadcrumbs left (which may be negative if the path is longer than the number of breadcrumbs) and prints that there were not enough breadcrumbs, just enough breadcrumbs, or the actual amount of breadcrumbs left For example, given the following forest map: forestPath = [[' ',' ',' ','X','X'],\ ['X','X',' ',' ','X'],\ ['X','X','X',' ','X'],\ [' ','X','X',' ',' '],\ [' ','X','X','X',' '],\ [' ',' ','X','X',' '],\ [' ',' ','X','X','E']] If the number of breadcrumbs given is 12, sample output would be: Enter number of breadcrumbs: 12 [['*', '*', '*', 'X', 'X'], ['X', 'X', '*', '*', 'X'], ['X', 'X', 'X', '*', 'X'], [' ', 'X', 'X', '*', '*'], [' ', 'X', 'X', 'X', '*'], [' ', ' ', 'X', 'X', '*'], [' ', ' ', 'X', 'X', 'E']] 2 breadcrumb(s) are left If the number of breadcrumbs given is 5, sample output would be: Enter number of breadcrumbs: 5 [['*', '*', '*', 'X', 'X'], ['X', 'X', '*', '*', 'X'], ['X', 'X', 'X', ' ', 'X'], [' ', 'X', 'X', ' ', ' '], [' ', 'X', 'X', 'X', ' '], [' ', ' ', 'X', 'X', ' '], [' ', ' ', 'X', 'X', 'E']] Not enough breadcrumbs for the trip. If the number of breadcrumbs given is 10, sample output would be: Enter number of breadcrumbs: 10 [['*', '*', '*', 'X', 'X'], ['X', 'X', '*', '*', 'X'], ['X', 'X', 'X', '*', 'X'], [' ', 'X', 'X', '*', '*'], [' ', 'X', 'X', 'X', '*'], [' ', ' ', 'X', 'X', '*'], [' ', ' ', 'X', 'X', 'E']] Phew, just enough breadcrumbs for the trip.

Step by step
Solved in 4 steps with 3 images

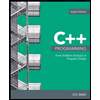
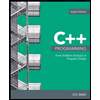