Write a python program that does the following: Reads information from a text file into a list of sublists. Be sure to ask the user to enter the file name and end the program if the file doesn’t exist. Text file format will be as shown, where each item is separated by a comma and a space: ID, firstName, lastName, birthDate, hireDate, salary Store the information into a list of sublists called empRoster. EmpRoster will be a list of sublists, where each sublist contains the information for one employee. The birthDate and hireDate in file are strings, but should be stored as objects of type Date. Example of EmpRoster list with 2 employees: [ [111,”Joe”, “Jones”, “09-01-1980”,”10-19-1999”, 95000], \ [222, “Sam”, “Smith”, “07-10-1956”,, “01-01-2000”, 50000] ] Print a report that shows the following: Employee Roster ID First Name Last Name Birth Date Age Hire Date YrsWorked Salary WRITE the following information out to a text file – ask the user what the text file should be named! Employees over 60 years old Employees with salaries above 50000 Employees that have worked for the company more than 20 years Example input file: 111, Joe, Jones, 09-01-1980, 10-19-1999, 95000 222, Sam, Smith, 07-10-1956, 01-01-2000, 50000 333, Rick, Robins, 08-01-1960, 08-20-1998, 100000 Example report for the sample data: Employee Roster ID First Name Last Name Birth Date Age Hire Date Yrs Worked Salary 111 Joe Jones Sep 1, 1980 39 Oct 19, 1999 21 95000 222 Sam Smith Jul 10, 1956 63 Jan 1, 2000 20 50000 333 Rick Robins Aug 1, 1960 59 Aug 20, 1998 22 100000 Example output file: Employees over 60 years old: Sam Smith Employees with salaries above 50000: Joe Jones Rick Robins Employees that have worked for the company more than 20 years Joe Jones Rick Robins
Write a python program that does the following:
- Reads information from a text file into a list of sublists.
- Be sure to ask the user to enter the file name and end the program if the file doesn’t exist.
- Text file format will be as shown, where each item is separated by a comma and a space:
ID, firstName, lastName, birthDate, hireDate, salary
- Store the information into a list of sublists called empRoster.
EmpRoster will be a list of sublists, where each sublist contains the information for one employee.
The birthDate and hireDate in file are strings, but should be stored as objects of type Date.
Example of EmpRoster list with 2 employees:
[ [111,”Joe”, “Jones”, “09-01-1980”,”10-19-1999”, 95000], \
[222, “Sam”, “Smith”, “07-10-1956”,, “01-01-2000”, 50000] ] - Print a report that shows the following:
Employee Roster
ID First Name Last Name Birth Date Age Hire Date YrsWorked Salary - WRITE the following information out to a text file – ask the user what the text file should be named!
- Employees over 60 years old
- Employees with salaries above 50000
- Employees that have worked for the company more than 20 years
- Example input file:
111, Joe, Jones, 09-01-1980, 10-19-1999, 95000
222, Sam, Smith, 07-10-1956, 01-01-2000, 50000
333, Rick, Robins, 08-01-1960, 08-20-1998, 100000 - Example report for the sample data:
Employee Roster
ID First Name Last Name Birth Date Age Hire Date Yrs Worked Salary
111 Joe Jones Sep 1, 1980 39 Oct 19, 1999 21 95000
222 Sam Smith Jul 10, 1956 63 Jan 1, 2000 20 50000
333 Rick Robins Aug 1, 1960 59 Aug 20, 1998 22 100000
- Example output file:
Employees over 60 years old:
Sam Smith
Employees with salaries above 50000:
Joe Jones
Rick Robins
Employees that have worked for the company more than 20 years
Joe Jones
Rick Robins

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

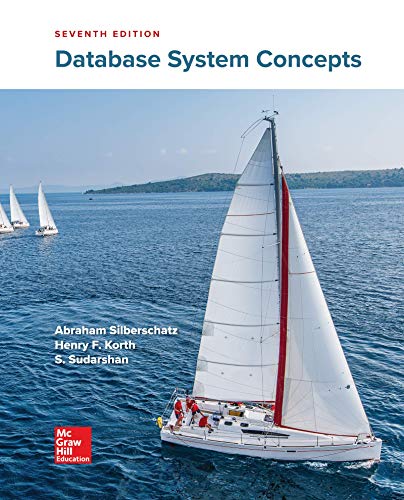
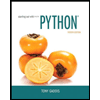
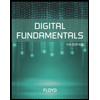
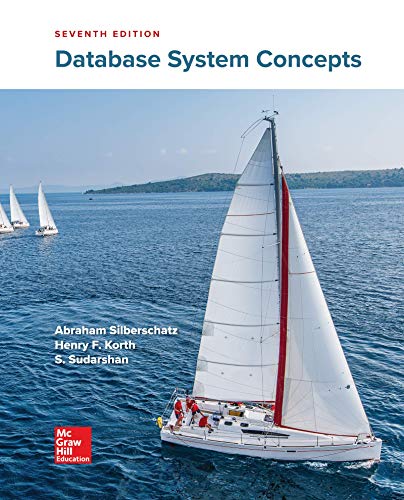
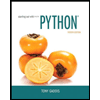
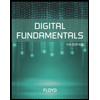
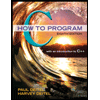
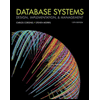
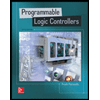