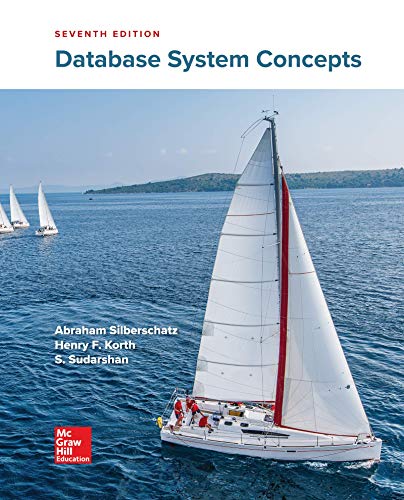
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
![Lom/pluginfile.php/1076589/mod resource/content/1/HW4.pdf
9 AAA
Invalid (AAA is not a valid letter combination)
Table A Example valid/invalid sequences.
Table B below shows example input file plates.txt and the corresponding expected content in
the output files.
plates.txt
11 A
valid.txt
invalid.txt
11 A
OOB
OOB
12 YY
222
12 YY
87652 M
500111 FA
87652 M
34091 D
D 999
222
„le)&0123456
34091 D
500111 FA
D 999
l©)&0123456
Table B Example input file and expected output files.
Exception Handling. Your program must catch and handle exceptions where appropriate, for
instance, when reading and writing files in addition to any other python runtime errors such as
ValueError and IOError, including any other potential errors that may emerge from your code.
/pluginfile.php/1076589/mod_resource/content/1/HW4.pdf
1) When opening file for reading an IOError may occur due to a number of reasons. Your
code should display the custom message File Read Error: to indicate attempt to open
the file for reading failed followed by the python error message. For example, if the input
file plates.txt cannot be opened for reading then the following message may be shown:
File Read Error: [Errno 2] No such file or directory: 'plates.txt
Note the first message File Read Error: is the custom message and the rest is python
specific error message. If the file cannot be opened for reading, then you must end the
program gracefully and no further processing is required at this point.
2) When reading the lines from the input file and parsing the line to find digits and letters
sequences; if the input line cannot be parsed (in other words the input line is not strictly
following the required format which is two sequences separated only by white spaces)
then your code should display the message Parse Error @ line number and indicate the
line number which contain the line from input that does not follow the required format
including the sequence found in that line. In addition to that, include any python error
messages. Your code should include this line among the output to be written to
invalid.txt file and proceed to processing next line in the input file. For example, when
the input file plates.txt as shown in Table B above is processed, the following messages
should be printed:
Parse Error @ line number 2: need more than 1 value to unpack: 00B
Parse Error @ line number 5: need more than 1 value to unpack: 222
Parse Error @ line number 9: need more than 1 value to unpack: l0)&0123456
Note that the above sequences are the only lines from input file in Table B that do not](https://content.bartleby.com/qna-images/question/3bda9717-13ba-410f-bdea-8631e1dda074/a99c7249-4f52-450f-a063-e472b171ff8a/e17upxh_thumbnail.jpeg)
Transcribed Image Text:Lom/pluginfile.php/1076589/mod resource/content/1/HW4.pdf
9 AAA
Invalid (AAA is not a valid letter combination)
Table A Example valid/invalid sequences.
Table B below shows example input file plates.txt and the corresponding expected content in
the output files.
plates.txt
11 A
valid.txt
invalid.txt
11 A
OOB
OOB
12 YY
222
12 YY
87652 M
500111 FA
87652 M
34091 D
D 999
222
„le)&0123456
34091 D
500111 FA
D 999
l©)&0123456
Table B Example input file and expected output files.
Exception Handling. Your program must catch and handle exceptions where appropriate, for
instance, when reading and writing files in addition to any other python runtime errors such as
ValueError and IOError, including any other potential errors that may emerge from your code.
/pluginfile.php/1076589/mod_resource/content/1/HW4.pdf
1) When opening file for reading an IOError may occur due to a number of reasons. Your
code should display the custom message File Read Error: to indicate attempt to open
the file for reading failed followed by the python error message. For example, if the input
file plates.txt cannot be opened for reading then the following message may be shown:
File Read Error: [Errno 2] No such file or directory: 'plates.txt
Note the first message File Read Error: is the custom message and the rest is python
specific error message. If the file cannot be opened for reading, then you must end the
program gracefully and no further processing is required at this point.
2) When reading the lines from the input file and parsing the line to find digits and letters
sequences; if the input line cannot be parsed (in other words the input line is not strictly
following the required format which is two sequences separated only by white spaces)
then your code should display the message Parse Error @ line number and indicate the
line number which contain the line from input that does not follow the required format
including the sequence found in that line. In addition to that, include any python error
messages. Your code should include this line among the output to be written to
invalid.txt file and proceed to processing next line in the input file. For example, when
the input file plates.txt as shown in Table B above is processed, the following messages
should be printed:
Parse Error @ line number 2: need more than 1 value to unpack: 00B
Parse Error @ line number 5: need more than 1 value to unpack: 222
Parse Error @ line number 9: need more than 1 value to unpack: l0)&0123456
Note that the above sequences are the only lines from input file in Table B that do not
![Task. Your task is to develop a python program that reads input from text file and finds if the
alphanumeric sequence in each line of the provided input file is valid for Omani car license plate.
The rules for valid sequences for car plates in Oman are as follows:
Each sequence is composed of 1 to 5 digits followed by one or two letters.
Digits cannot start with 0, for instance, 00, 011, or 09 are not valid digit sequences.
The following list are the only valid letter combinations:
['A','AA','AB','AD','AR','AM','AW','AY',
'B','BA','BB','BD','BR','BM','BW','BY',
'D',DA','DD','DR','DW','DY',
"R',RA','RR','RM','RW','RY',
'S','SS',
'M', 'MA','MB','MM','MW','MY',
W',WA','WB','ww,
Y,YA','YB','YD','YR','YW','YY']
Program Input/Output. Your program should read input from a file named plates.txt and write
lines with valid sequences to a file named valid.txt. Any line from the input file containing invalid
sequence should be written to a file named invalid.txt. Each line from the input file must be
written to a separate line to the respective output files.
Each line in the input file will be composed of exactly two sequences separated by any number
of white spaces. Valid lines will be composed of 1 to 5 digits followed by one or more spaces
followed by one or two letters without any spaces between digits or letters. Any line that does
not follow this requirement may be considered invalid and included as output to file invalid.txt.
after shown appropriate message as described below in the exception handling section. Table
A below shows example sequences and their validity for car plates.
Example Sequence
Validity
12 A
Valid
123 BB
Valid
Invalid (BZ is not a valid letter combination)
Invalid (cannot start with zero digit)
10 BZ
00 R
Invalid (missing digits)
Invalid (more than 5 digits)
MM
123456 B
1110 Y
Valid
Invalid (AAA is not a valid letter combination)
Table A Example valid/invalid sequences.
9 AAA](https://content.bartleby.com/qna-images/question/3bda9717-13ba-410f-bdea-8631e1dda074/a99c7249-4f52-450f-a063-e472b171ff8a/aim8y8n_thumbnail.jpeg)
Transcribed Image Text:Task. Your task is to develop a python program that reads input from text file and finds if the
alphanumeric sequence in each line of the provided input file is valid for Omani car license plate.
The rules for valid sequences for car plates in Oman are as follows:
Each sequence is composed of 1 to 5 digits followed by one or two letters.
Digits cannot start with 0, for instance, 00, 011, or 09 are not valid digit sequences.
The following list are the only valid letter combinations:
['A','AA','AB','AD','AR','AM','AW','AY',
'B','BA','BB','BD','BR','BM','BW','BY',
'D',DA','DD','DR','DW','DY',
"R',RA','RR','RM','RW','RY',
'S','SS',
'M', 'MA','MB','MM','MW','MY',
W',WA','WB','ww,
Y,YA','YB','YD','YR','YW','YY']
Program Input/Output. Your program should read input from a file named plates.txt and write
lines with valid sequences to a file named valid.txt. Any line from the input file containing invalid
sequence should be written to a file named invalid.txt. Each line from the input file must be
written to a separate line to the respective output files.
Each line in the input file will be composed of exactly two sequences separated by any number
of white spaces. Valid lines will be composed of 1 to 5 digits followed by one or more spaces
followed by one or two letters without any spaces between digits or letters. Any line that does
not follow this requirement may be considered invalid and included as output to file invalid.txt.
after shown appropriate message as described below in the exception handling section. Table
A below shows example sequences and their validity for car plates.
Example Sequence
Validity
12 A
Valid
123 BB
Valid
Invalid (BZ is not a valid letter combination)
Invalid (cannot start with zero digit)
10 BZ
00 R
Invalid (missing digits)
Invalid (more than 5 digits)
MM
123456 B
1110 Y
Valid
Invalid (AAA is not a valid letter combination)
Table A Example valid/invalid sequences.
9 AAA
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- In this coding challenge, you will be retrieving email usernames from a string. You will write a function named get_usernames () that takes in a string as it's input parameter, and returns a list of email usernames in the string. The input strings will contain 0 or more emails in the format and the '@' symbol will only appear in the context of an email. The order of usernames should match the order in the input text. username@domainname, For example: EXAMPLE 1 text: "If you need help on an assignment, email help@ucsd.edu or support@gmail.com" return: ['help', 'support'] EXAMPLE 2 text: "Good morning! I hope you're having a great time with CSE 8A!" return: [] EXAMPLE 3 text: "I've been having a lot of trouble reaching you, can you please email me at cse@ucsd.edu? return: ['cse', 'cse'] Here is the problem description: Function Name: get_usernames Parameter: text - A string corresponding to a piece of text containing 0 or more emails. Return: A list of the email usernames in the input…arrow_forwardIn Python, Utilizing the following functions headers: Main() Create a program that will utilize a list of states and their capitals. The outcome will be two printed sorted lists, one of which is just the states and the other is the capitals. State_capitals = [‘Oregon’, ‘Salem’, ‘Michigan’, ‘Lansing’, ‘California’, ‘Sacramento’, ‘Texas’, ‘Austin’,‘Massachusetts’, ‘Boston’, ‘Georgia’, ‘Atlanta’, ‘Colorado’, ‘Denver’, ‘Hawaii’, ‘Honolulu’, ‘Arizona’, ‘Phoenix’, ‘Kentucky’, ‘Frankfurt’] Sample output: Capitals = [‘Salem’, ‘Lansing’, ‘Sacramento’, ‘Austin’, ‘Boston’, ‘Atlanta’, ‘Denver’, ‘Honolulu’, ‘Phoenix’ ‘Frankfurt’] States = [‘Oregon’, ‘Michigan’, ‘California’, ‘Texas’, ‘Massachusetts’, ‘Georgia‘, ‘Colorado’, ‘Hawaii’, ‘Arizona’, ‘Kentucky’] In Python, Utilizing the following functions headers: Main() Create a program that returns a new list that only has elements that are common between the lists (without duplicates). Please be positive that the program runs on two lists…arrow_forwardPlease help me with my code. Create a program in Python using RegEx that reads data from Breakfast Menu (https://www.w3schools.com/xml/simple.xml) and builds parallel arrays for the menu items, with each array containing the menu item name, description, calories, and price, respectively. After reading the data and building the arrays, display the menu items similar to the following: name - description - calories - priceAt the bottom, display the total number of items on the menu, the average number of calories per item, and the average price per item similar to: 0 items - 0 average calories - $0.00 average price You may either read the page using Internet processing methods, or you may download and save the page and then read the data from the saved file. You must process the data using string functions (no XML libraries). must use separate subroutines/functions/methods to implement each type of processing, and include error handling for missing or invalid data. Please use…arrow_forward
- In pythonWrite a function called compter_les_votes that accepts a list of ballots as input (of arbitrary length) and produces a report as a dictionary. The ballots have the form of a couple (valid, condidat) where candidate is the name of one of the candidates in the election, and valid is a boolean that indicates whether or not the ballot is valid. For example, for the following list of bulletins: [(True, 'Pierre'), (False, 'Jean'), (True, 'Pierre'), (True, 'Jacques')]Your function must return the following dictionary: { "number of ballots": 4, "invalid ballots": 1, "results": { "Stone": 2, "Jacques": 1 }}Note that you do not have to display the dictionary, only return it.arrow_forwardThe image is all, it is python codingarrow_forwardplease use python.arrow_forward
- PYTHON Complete the function below, which takes two arguments: data: a list of tweets search_words: a list of search phrases The function should, for each tweet in data, check whether that tweet uses any of the words in the list search_words. If it does, we keep the tweet. If it does not, we ignore the tweet. data = ['ZOOM earnings for Q1 are up 5%', 'Subscriptions at ZOOM have risen to all-time highs, boosting sales', "Got a new Mazda, ZOOM ZOOM Y'ALL!", 'I hate getting up at 8am FOR A STUPID ZOOM MEETING', 'ZOOM execs hint at a decline in earnings following a capital expansion program'] Hint: Consider the example_function below. It takes a list of numbers in numbers and keeps only those that appear in search_numbers. def example_function(numbers, search_numbers): keep = [] for number in numbers: if number in search_numbers(): keep.append(number) return keep def search_words(data, search_words):arrow_forwardWrite a program that read the total rainfall for each of the 12 months into a list. The program should calculate and display the total rainfall for the year, the average monthly rainfall, and the months with the highest and lowest amounts. The input data is available in Program9.txt on the I: drive.No input, processing, or output should happen in the main function. All work should be delegated to other functions. The program should have at least 5 functions (main and developerInfo included). Include the recommended minimum documentation for each function. See the program one template for more details. Do not use any global variable.arrow_forwardInstructions: I need Python help. The problem I am trying to figure out so that when we work a similar problem in class I can do my assignment. Tonights assignment is a participation thing, Friday I need to do a similar assignment in class for a grade. Write a program that reads movie data from a CSV (comma separated values) file and output the data in a formatted table. The program first reads the name of the CSV file from the user. The program then reads the CSV file and outputs the contents according to the following requirements: Each row contains the title, rating, and all showtimes of a unique movie. A space is placed before and after each vertical separator ('|') in each row. Column 1 displays the movie titles and is left justified with a minimum of 44 characters. If the movie title has more than 44…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
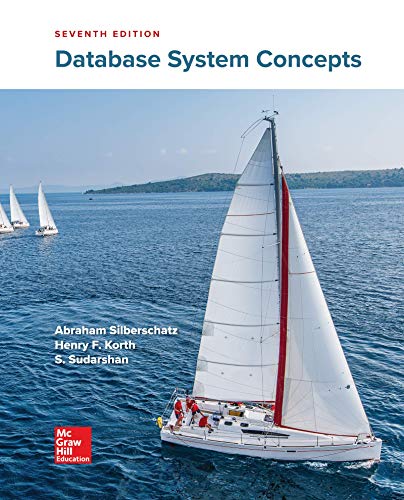
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
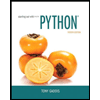
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
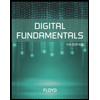
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
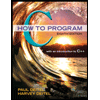
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
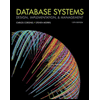
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
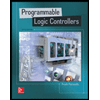
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education