Write a python program which displays a simple menu as follows: Python ISBN Conversion Menu Verify the check digit of an ISBN-10 2. Verify the check digit of an ISBN-13 3. Convert an ISBN-10 to an ISBN-13 Convert an ISBN-13 to an ISBN-10 4. 1. 5. Exit Please remember to use what you have learned during the semester such as: Functions and/or Value Returning Functions If structures or logic structures IF/Elif/ELSE Data structures i.e. File input/output String manipulations i.e. string slicing and lists, etc. Modules Loops i.e. WHILE loops or FOR loops
I need some help fixing this code. I need it to let me enter the ISBN number witht the dashes and find the check number my code is below Ill like my teachers direction as a refernce.
def main():
while True:
print('1. Verify the check digit of an ISBN-10')
print("2. verify the check digit of an ISBN-13")
print("3. Convert an ISBN-10 to an ISBN-13")
print('4. Convert an ISBN-13 to an ISBN-10')
print('5. Exit')
command = input("Enter the Command (1-5): ")
if command == '5':
break
elif command == '1' or command == '3':
# get 10 digit input
ISBN = input("Please enter the ISBN-10 number: ")
ISBN = ISBN.replace('-', '')
while len(ISBN) != 10:
print("Please make sure you have entered a number which is exactly 10 characters long.")
ISBN = input("Please enter the ISBN number: ")
ISBN = ISBN.replace('-', '')
print(format(ISBN))
if command == '1':
print(check_10_digit(ISBN))
else:
print(convert_10_to_13(ISBN))
elif command == '2' or command == '4':
# get 13 digit input
ISBN = input("Please enter the 13 digit number: ")
ISBN = ISBN.replace('-', '')
while len(ISBN) != 13:
print("Please make sure you have entered a number which is exactly 13 characters long")
ISBN = input("Please enter the 13 digit number: ")
ISBN = ISBN.replace('-', '')
if command == '2':
print(check_13_digit(ISBN))
else:
print(convert_13_to_10(ISBN))
def check_10_digit(ISBN):
#This portion of code will pull the entered ISBN from my Menu() statement and add all the numbers.
#It will then find the remainder of those numbers, if th remainder is 0 it will say the ISBN is valid
#If it comes back with the formula having a remainder, it will say NOT Valid.
digit = 0
for i in range(len(ISBN)):
n = ISBN[i]
num = int(n)
digit += (i+1) * num
d10 = digit % 11
print("Your ISBN-10 is: ", ISBN, "with a remainder of", str(d10))
if d10 == 0:
print("\nYour ISBN-10 is valid")
else:
print("\nYour ISBN-10 is NOT valid")
def check_13_digit(ISBN):
#Calcualte the ISBN Check Digit for 13 digit numbers
n = str(ISBN)
digit = 0
for i in range(len(n)):
if i % 2 == 0:
digit += int(n[i])
else:
digit += int(n[i]) * 3
digit = digit % 10
if digit == 0:
print("\nYour remainder is: ", digit, "your ISBN-13 is Valid")
else:
print("\nYour remainder is: ", digit, "your ISBN-13 is NOT Valid")
def convert_10_to_13(ISBN):
#Convert an ISBN-10 number to a ISBN-13 number
ISBN_13 = '978' + ISBN[:-1]
n = str(ISBN_13)
digit = 0
for i in range(len(n)):
if i % 2 == 0:
digit += int(n[i])
else:
digit += int(n[i]) * 3
digit = digit % 10
ISBN_New = str(ISBN_13) + str(digit)
print("\nThe ISBN-10 number ", ISBN, "is converted to the ISBN-13 number ", ISBN_New)
def convert_13_to_10(ISBN):
#Convert an ISBN-13 number to a ISBN-10 number
if ISBN[:3] == '978':
n = str(ISBN[3:-1])
else :
raise Exception("\nISBN is not convertible.")
digit = 0
for i in range(len(n)):
if i % 2 == 0:
digit += int(n[i])
else:
digit += int(n[i]) * 3
digit = 10 - (digit % 10)
ISBN_New = str(n) + str(digit)
print("\nThe ISBN-13 number ", ISBN, "is converted to the ISBN-10 number ", ISBN_New)
main()
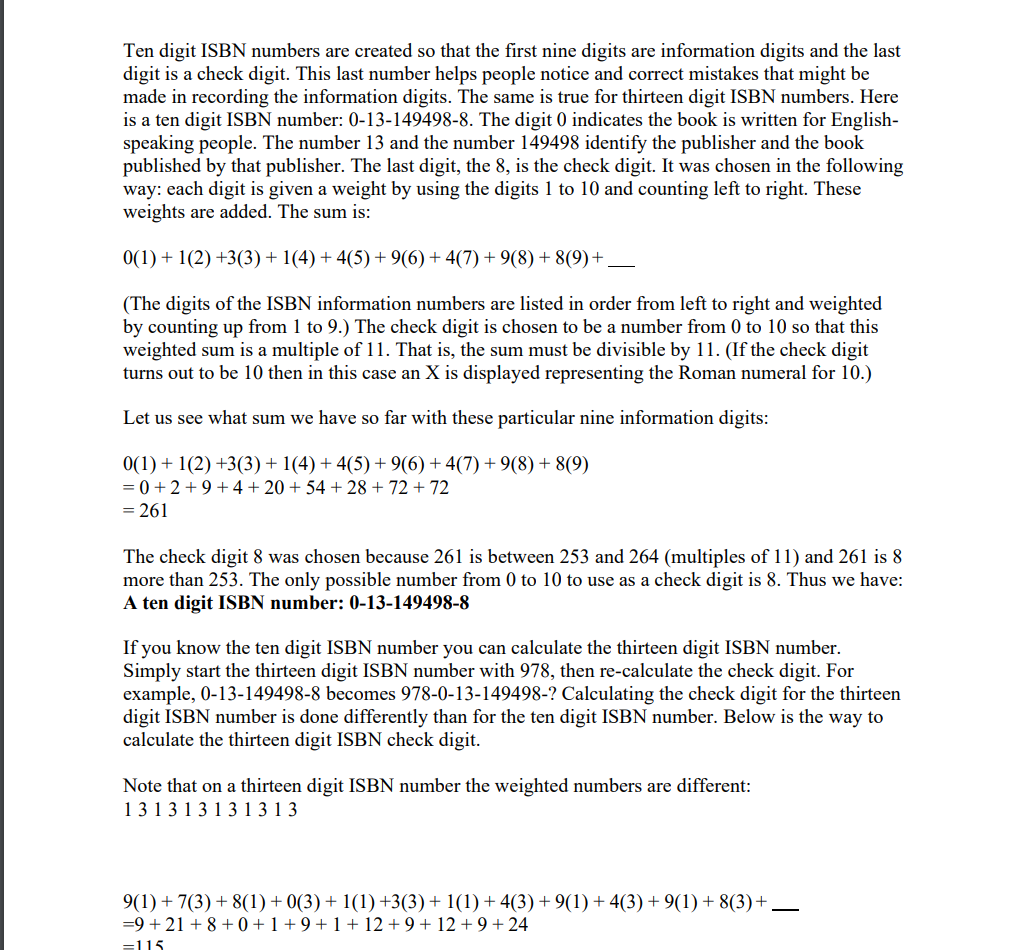
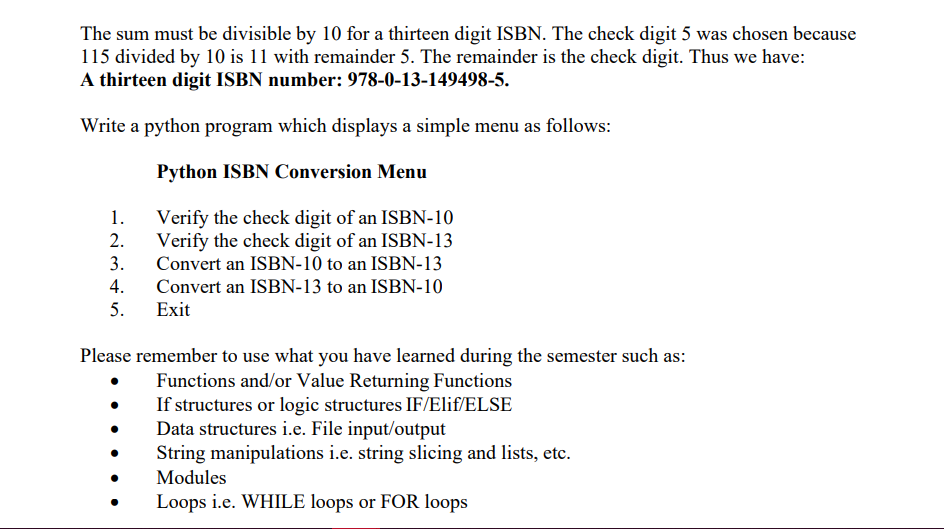

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

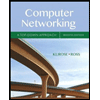
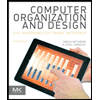
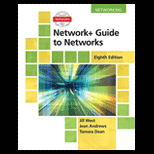
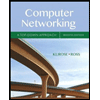
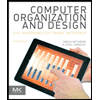
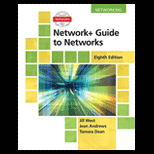
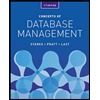
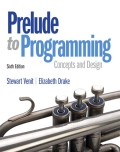
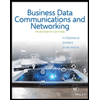