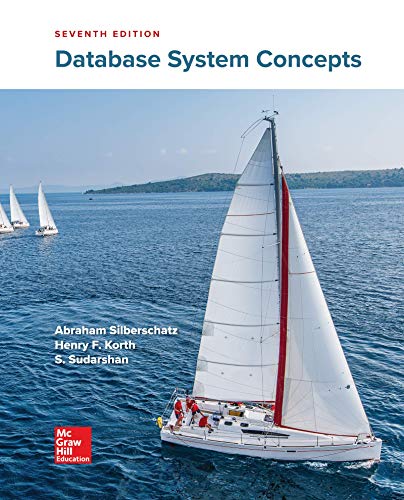
Write a Java or C++ program that will display the given menu:
A Simple Library System
1. Add a Student
2. Add a Book
3. Display All Students
4. Display All Books
5. Search a Student
6. Search a Book
7. Borrow a Book
8. Display Borrowed Books
9. Return a Book (optional)
10. Exit
➢ When 1 is chosen, ask the user to enter the student’s information(5-digit ID number,
surname, first name, age, and sex)
➢ When 2 is chosen, ask the user to enter the book’s information(5-digit book number,
Title, Author’s name(Surname, first name), date of purchase, and status (available/not
available))
➢ When 3 is chosen, display list of all students(5-digit ID number, surname, first name,
age, and sex)
➢ When 4 is chosen, display list of all books(5-digit book number, Title, Author’s
name(Surname, first name), date of purchase, and status (available/not available)
➢ When 5 is chosen, ask the user if he wants to search the list by Surname or ID
number. If record is found, display the corresponding record, else display an
appropriate prompt.
➢ When 6 is chosen, ask the user if he wants to search the list by Title, Author or Book
number. If record is found, display the corresponding record, else display an
appropriate prompt.
➢ When 7 is chosen is chosen, display the list of all available books, then ask the user
the ID number of the student who wants to borrow a book, the book that he wants to
borrow, and the current date.
➢ When 8 is chosen, display the list of borrowed books (display Book Number, Title,
borrower’s name(Surname, first name), and date Borrowed)
➢ When 9 is chosen, ask the user the book number and the student ID number then
update the corresponding record (you will get an additional 10 points(maximum) if
you include this in your program)
➢ When 10 is chosen , end the program
Other Requirements/limitations
➢ The data(student and book records must be stored in a file for permanent storage
(use Java file handling)
➢ Include exception handling (data validation)
➢ Use of Java Swing is not allowed(GUI is not required)
Submission Requirements
➢
➢ *.java, *.class, executable files and data/text files(containing the initial records)

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps

- Congrats! You work for Zillow now. Your first task is to write an algorithm that recommends apartments based on a user’s preferences. Zillow offers the following three properties for rent: Apartment A – 600 square feet, pets allowed, $1400/month Apartment B – 800 square feet, no pets, $1600/month Apartment C – 1000 square feet, pets allowed, $1800/month Write an algorithm for a program that does the following: Prompt the user for the minimum square footage they will accept, how many pets they have, and the maximum price they’d pay per month, and then calculate and output the apartments that match their preferences. If there is no apartment that matches their preferences, print out "No matches, sorry!". Make sure the inputs are valid--negative square footages, prices, and pet numbers don’t make sense!arrow_forwardpython multiple choice Code Example 4-1 def get_username(first, last): s = first + "." + last return s.lower() def main(): first_name = input("Enter your first name: ") last_name = input("Enter your last name: ") username = get_username(first_name, last_name) print("Your username is: " + username) main() 5. Refer to Code Example 4-1: What is the scope of the variable named s ?a. globalb. localc. global in main() but local in get_username()d. local in main() but global in get_username()arrow_forwardNumber to Words: Write a Java program to convert a given number to its equivalent words representation. For example, 123 -> "one hundred twenty-three".arrow_forward
- A fish-finder is a device used by anglers to find fish in a lake. If the fish-finder finds a fish, it will sound an alarm. It uses depth readings to determine whether to sound an alarm. For our purposes, the fish-finder will decide that a fish is swimming past if:there are four consecutive depth readings which form a strictly increasing sequence (such as 3 4 7 9) (which we will call "Fish Rising"), orthere are four consecutive depth readings which form a strictly decreasing sequence (such as 9 6 5 2) (which we will call "Fish Diving"), orthere are four consecutive depth readings which are identical (which we will call "Constant Depth").All other readings will be considered random noise or debris, which we will call "No Fish."Create a Python program called "fishfinder_firstname_lastname" that takes 1 input of string of 4 numbers separated with comma. Your program must display "Fish Rising", "Fish Diving.", "Fish At Constant Depth" or "No Fish".Example:30,10,20,20 Must display No…arrow_forwardJava Programming: The program will figure out a number chosen by a user. Ask user to think of a number from 1 to 100. The program will make guesses and the user will tell the program to guess higher (h) or lower (l). Sample run of the program might look like below : Guess a number from 1 to 100. (here, the user is thinking of 81) Is it 50? (h/l/c): h Is it 75? (h/l/c): h Is it 88? (h/l/c): l Is it 81? (h/l/c): c Great! Do you want to play again? (y/n): y Guess a number from 1 to 100. (here the user is thinking 37) Is it 50? (h/l/c): l Is it 25? (h/l/c): h Is it 37? (h/l/c): c Great! Do you want to play again? (y/n): n our program should implement the binary search algorithm. Every time the program makes a guess it should guess the midpoint of the remaining possible values. Consider the first example above, in which the user has chosen the number 81: On the first guess, the possible values are 1 to 100. The midpoint is 50. The user responds by saying “higher” On the second…arrow_forwardComputer programmin. Java.arrow_forward
- Write a python program which displays a simple menu as follows:Python ISBN Conversion Menu1. Verify the check digit of an ISBN-102. Verify the check digit of an ISBN-133. Convert an ISBN-10 to an ISBN-134. Convert an ISBN-13 to an ISBN-105. Exit Please remember to use what you have learned during the semester such as:• Functions and/or Value Returning Functions• If structures or logic structures IF/Elif/ELSE• Data structures i.e. File input/output• String manipulations i.e. string slicing and lists, etc.• Modules• Loops i.e. WHILE loops or FOR loops I already created the program by using Python, but here is what I need help with (in bold):• Reading and writing data to a file(s). In other words, have the program to read ISBNs from a file and store the correct results in a file as well.Can you include codes within my current program (included below) so that my program will read ISBNs from a file and store the correct results in a file as well? * Please make sure to use the Python program…arrow_forwardUsing introduction to Python You have been asked to write an application that automatically grades a quiz. The quiz has 15 multiple-choice questions. The correct answers are: 1.A, 2.C, 3.A, 4.B, 5.B, 6.D, 7.D, 8.A, 9.C, 10.A, 11.B, 12.C, 13.D, 14.C, 15.B The program will start by creating a list holding the quiz answers key shown above. It will then prompt the user for the student name and their answers file and read them into a separate list. It will finally compare the key vs. answers lists to calculate & display: Number of correct answersNumber of incorrect answersQuestions incorrectly answered.Whether the student has passed or failed the quizA student must correctly answer 11 out of 15 questions to pass.The user can grade as many students as they wish. Notes The file containing the student’s answers is organized as 15 lines. Each line contains the answer to the corresponding question. The responses are single character strings, from A to D, uppercase. Must use functional…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
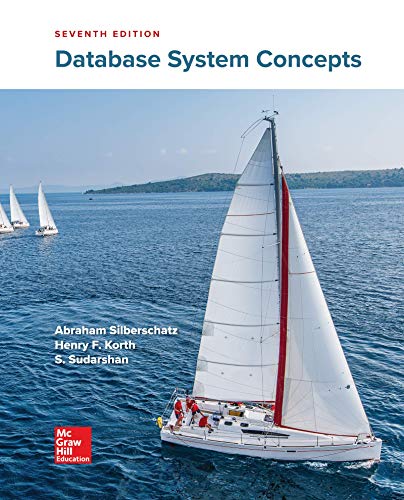
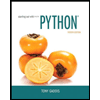
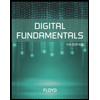
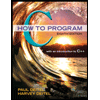
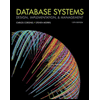
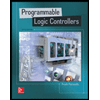