Programming language: C++ Implement a main function that profiles the performance of insert (See below the code) and outputs a table showing the average time per insert as the length of the array increases. Make sure your source code is well-commented, consistently formatted, uses no magic numbers/values,
Programming language: C++ Implement a main function that profiles the performance of insert (See below the code) and outputs a table showing the average time per insert as the length of the array increases. Make sure your source code is well-commented, consistently formatted, uses no magic numbers/values,
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter7: Arrays
Section7.4: Arrays As Arguments
Problem 6E: (Electrical eng.) Write a program that declares three one-dimensional arrays named volts, current,...
Related questions
Question
Implement a main function that profiles the performance of insert (See below the code) and outputs a table showing the average time per insert as the length of the array increases. Make sure your source code is well-commented, consistently formatted, uses no magic numbers/values, follows programming best-practices, and is ANSI-compliant. It is expected to have the program output
![1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
Bint* insert(int* array, int length, int index, int value)
{
8
18
#include <iostream>
using namespace std;
8
if (length == 0)
{
int* newArray = new int [1];
newArray [0] = value;
return newArray;
}
else
{
int* newArray = new int[length + 1];
//Copying the array from 0 to index
for (int i = 0; i < index; i++)
{
newArray[i]
array[i];
=
newArray[index] = value;
//Copying the array from index to length
int j = index + 1;
for (int i = index; i < length; i++)
{
newArray[j] = array[i];
delete[] array;
return newArray;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Facc2152e-e161-4281-84a8-08d1f99f5c73%2Fb190b20d-110f-4000-a959-d1ae8c76796b%2Fl9l8mo4_processed.png&w=3840&q=75)
Transcribed Image Text:1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
Bint* insert(int* array, int length, int index, int value)
{
8
18
#include <iostream>
using namespace std;
8
if (length == 0)
{
int* newArray = new int [1];
newArray [0] = value;
return newArray;
}
else
{
int* newArray = new int[length + 1];
//Copying the array from 0 to index
for (int i = 0; i < index; i++)
{
newArray[i]
array[i];
=
newArray[index] = value;
//Copying the array from index to length
int j = index + 1;
for (int i = index; i < length; i++)
{
newArray[j] = array[i];
delete[] array;
return newArray;
![Pseudocode:
main ()
/* Setting to allow fine-tuning the granularity of the readings */
Let INSERTS_PER_READING = 1000
/* Start with an empty array */
Let array = empty array (i.e. NULL)
Let length = 0
/* Take 60 readings */
Loop 60 times
/* Each reading will be taken after INSERTS_PER_READING inserts */
Let startTime = current time
Loop INSERTS_PER_READING times.
Let index = random integer in range [0, length]
Let value = random integer value
Let array = insert (array, length, index, value)
Let length = length + 1
End Loop
Let stopTime = current time
Let time PerInsert
=
(stopTime startTime) / INSERTS_PER_READING
/* Output reading in tabular format */
Output array length and timePerInsert
End Loop
/* Free the old array */
Free array](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Facc2152e-e161-4281-84a8-08d1f99f5c73%2Fb190b20d-110f-4000-a959-d1ae8c76796b%2Ftc7cjhq_processed.png&w=3840&q=75)
Transcribed Image Text:Pseudocode:
main ()
/* Setting to allow fine-tuning the granularity of the readings */
Let INSERTS_PER_READING = 1000
/* Start with an empty array */
Let array = empty array (i.e. NULL)
Let length = 0
/* Take 60 readings */
Loop 60 times
/* Each reading will be taken after INSERTS_PER_READING inserts */
Let startTime = current time
Loop INSERTS_PER_READING times.
Let index = random integer in range [0, length]
Let value = random integer value
Let array = insert (array, length, index, value)
Let length = length + 1
End Loop
Let stopTime = current time
Let time PerInsert
=
(stopTime startTime) / INSERTS_PER_READING
/* Output reading in tabular format */
Output array length and timePerInsert
End Loop
/* Free the old array */
Free array
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
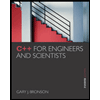
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
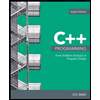
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
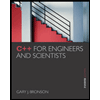
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
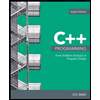
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
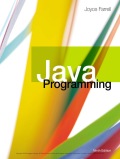
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
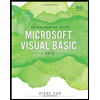
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
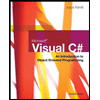
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,