Write a static method named SmallestEvenStackQueue ToList that takes two parameters: an array-based unbounded queue of integers as its first parameter and an array-based bounded stack of integers as its second parameter. The method should find the smallest even integers from all corresponding values in the stack and queue and save them into an object of type ArrayUnsortedList and return this object from the method. If two corresponding elements in the stack and queue are odd integers, then add the value -1 to the unsorted list. Assume that stack and queue have the same size. Example1: if the queue has: 1 4 4 9. 8 10 If the stack has: 4 3 10 9. 5 20 Then the unsorted list has: 4 -1 4 4 -1 2 10 The stack and queue must become empty after calling the method
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package Linked_List;
import java.util.Scanner;
/**
*
* @author lab1257-15
*/
public class Demo {
public static boolean has42 (RefUnsortedList<Integer> t)
{
t.reset();
for (int i=1; i<=t.size();i++)
if (t.getNext()==42)
return true;
return false;
}
public static void RemoveAll(RefUnsortedList<Integer> t, int v)
{
while(t.contains(v))
{
t.remove(v);
}
}
public static void main(String[] args) {
}
}
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package Linked_List;
/**
*
* @author lab1257-15
*/
public class LLNode<T>
{
private LLNode<T> link;
private T info;
public LLNode(T info)
{
this.info = info;
link = null;
}
public void setInfo(T info)
// Sets info of this LLNode.
{
this.info = info;
}
public T getInfo()
// Returns info of this LLONode.
{
return info;
}
public void setLink(LLNode<T> link)
// Sets link of this LLNode.
{
this.link = link;
}
public LLNode<T> getLink()
// Returns link of this LLNode.
{
return link;
}
}
package Linkedqueue;
public class LinkedUnbndQueue<T> implements UnboundedQueueInterface<T>
{
protected LLNode<T> front; // reference to the front of this queue
protected LLNode<T> rear; // reference to the rear of this queue
public LinkedUnbndQueue()
{
front = null;
rear = null;
}
public void enqueue(T element)
// Adds element to the rear of this queue.
{
LLNode<T> newNode = new LLNode<T>(element);
if (rear == null)
front = newNode;
else
rear.setLink(newNode);
rear = newNode;
}
public T dequeue()
// Throws QueueUnderflowException if this queue is empty;
// otherwise, removes front element from this queue and returns it.
{
if (isEmpty())
throw new QueueUnderflowException("Dequeue attempted on empty queue.");
else
{
T element;
element = front.getInfo();
front = front.getLink();
if (front == null)
rear = null;
return element;
}
}
public boolean isEmpty()
// Returns true if this queue is empty; otherwise, returns false.
{
if (front == null)
return true;
else
return false;
}
}
package Linkeded;
public class LinkedStack<T> implements UnboundedStackInterface<T>
{
LLNode<T> top; // reference to the top of this stack
public LinkedStack()
{
top = null;
}
public void push(T element)
// Places element at the top of this stack.
{
LLNode<T> newNode = new LLNode<T>(element);
newNode.setLink(top);
top = newNode;
}
public void pop()
// Throws StackUnderflowException if this stack is empty,
// otherwise removes top element from this stack.
{
if (!isEmpty())
{
top = top.getLink();
}
else
throw new StackUnderflowException("Pop attempted on an empty stack.");
}
public T top()
// Throws StackUnderflowException if this stack is empty,
// otherwise returns top element from this stack.
{
if (!isEmpty())
return top.getInfo();
else
throw new StackUnderflowException("Top attempted on an empty stack.");
}
public boolean isEmpty()
// Returns true if this stack is empty, otherwise returns false.
{
if (top == null)
return true;
else
return false;
}
void push(LLNode<Integer> top) {
throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates.
}
public void print()
{
LLNode<T> temp;
temp=top;
while(temp!=null)
{
System.out.println(temp.getInfo());
temp=temp.getLink();
}
}
public int sumLS()
{
LLNode<T> temp;
temp=top;
int sum=0;
while(temp!=null)
{
int x= (Integer)temp.getInfo();
sum=sum+x;
temp=temp.getLink();
}
return sum;
}
}
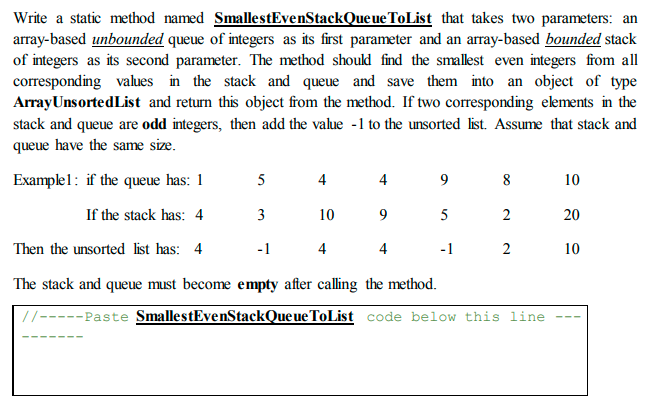

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

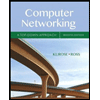
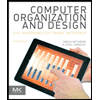
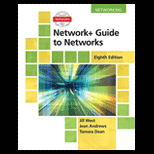
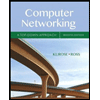
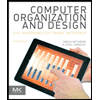
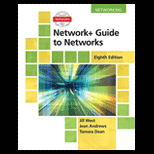
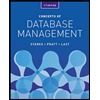
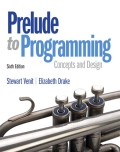
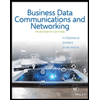