implement a LinkedList class that stores integers using dynamic memory and a proper main program to test it. The following member functions need to be properly implemented and tested: 1. Default Constructor. 2. Parametric Constructor (takes an integer as the argument). 3. Sum: Function to sum all the elements of a list and return an integer as an output. 4. Average: Function that returns the average of all numbers in the list as a float. 5. InsertAtHead: Function that takes in an integer as an argument and inserts it at the head of the linked list. 6. InsertAtTail: Function that takes an integer as an argument and inserts it at the end of the list. 7. Delete: Takes an integer and deletes all instances of that number in the linked list. 8. Pop: Deletes and returns the node at the head (note: return type should be node, not int). 9. Circular: Makes the list circular by having the last node in the list point to the header. 10. Display: Displays all the integers in the list, and displays the value at head twice, once in the start and once in the end (to test for circularity, think carefully on how you will end your display loop).
implement a LinkedList class that stores integers using dynamic memory and a proper main program to test it. The following member functions need to be properly implemented and tested: 1. Default Constructor. 2. Parametric Constructor (takes an integer as the argument). 3. Sum: Function to sum all the elements of a list and return an integer as an output. 4. Average: Function that returns the average of all numbers in the list as a float. 5. InsertAtHead: Function that takes in an integer as an argument and inserts it at the head of the linked list. 6. InsertAtTail: Function that takes an integer as an argument and inserts it at the end of the list. 7. Delete: Takes an integer and deletes all instances of that number in the linked list. 8. Pop: Deletes and returns the node at the head (note: return type should be node, not int). 9. Circular: Makes the list circular by having the last node in the list point to the header. 10. Display: Displays all the integers in the list, and displays the value at head twice, once in the start and once in the end (to test for circularity, think carefully on how you will end your display loop).
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter17: Linked Lists
Section: Chapter Questions
Problem 9PE
Related questions
Question
This one.
In c++
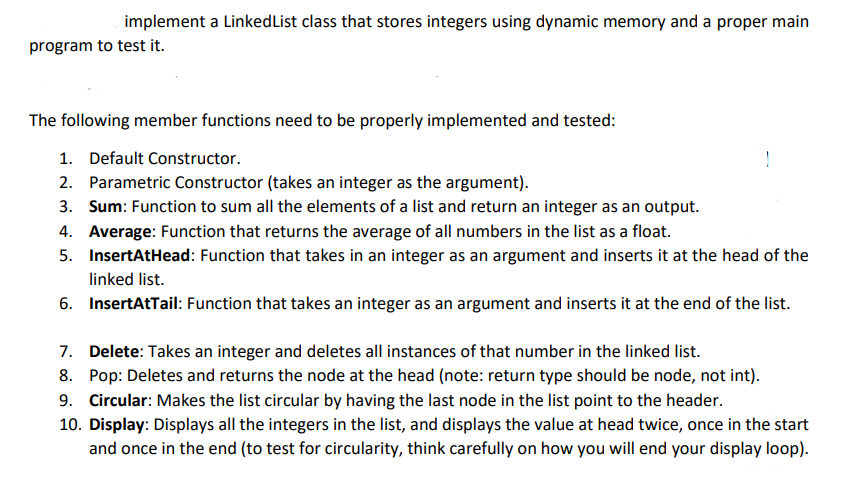
Transcribed Image Text:implement a LinkedList class that stores integers using dynamic memory and a proper main
program to test it.
The following member functions need to be properly implemented and tested:
1. Default Constructor.
!
2. Parametric Constructor (takes an integer as the argument).
3. Sum: Function to sum all the elements of a list and return an integer as an output.
4. Average: Function that returns the average of all numbers in the list as a float.
5. InsertAtHead: Function that takes in an integer as an argument and inserts it at the head of the
linked list.
6. InsertAtTail: Function that takes an integer as an argument and inserts it at the end of the list.
7. Delete: Takes an integer and deletes all instances of that number in the linked list.
8. Pop: Deletes and returns the node at the head (note: return type should be node, not int).
9. Circular: Makes the list circular by having the last node in the list point to the header.
10. Display: Displays all the integers in the list, and displays the value at head twice, once in the start
and once in the end (to test for circularity, think carefully on how you will end your display loop).
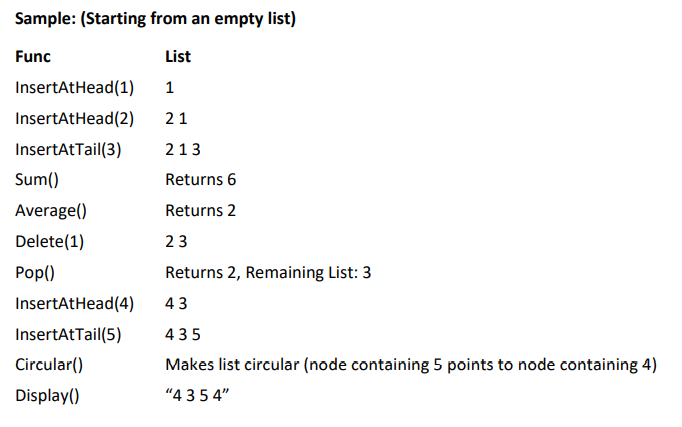
Transcribed Image Text:Sample: (Starting from an empty list)
Func
List
InsertAtHead(1)
InsertAtHead(2)
21
InsertAtTail(3)
213
Sum()
Returns 6
Average()
Returns 2
Delete(1)
23
Pop()
Returns 2, Remaining List: 3
InsertAtHead(4)
43
InsertAtTail(5)
435
Circular()
Makes list circular (node containing 5 points to node containing 4)
Display()
"4 35 4"
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Recommended textbooks for you
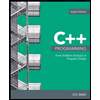
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
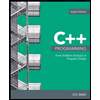
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning