Write C program language Department of computer science offers a set of courses each semester. These courses are listed in a file as follows: course name, course id, number of registered student, and max number of students. Number of registered students is initialized to zero always. The file that contains the courses’ information is formatted as follows (i.e. sample course file): Each semester, students are required to register a set of courses. The students are listed in a file along with the courses to be registered as follows: student name, student id, courses to be registered (COMP242, COMP336, COMP231...). The file that contains the students’ information is formatted as follows (i.e. sample student file): Course File Java#COMP231#0#27 Data Structures #COMP2321#0#30 Algorithms#COMP336#0#15 Computer and Programming#COMP142#0#27 Student File Ahmad Ali#1159999#COMP242#COMP336#COMP231#COMP338 Ali Mahmoud#1169999#COMP142#COMP336#COMP231 Ashraf Hasan #1139999#COMP142#COMP336#COMP231#COMP333#COMP 432#COMP338#COMP233 Programming Requirements: 1. Your program should use the concept of struct. Hint: create two structs, one for course and the second for student. typedef struct { char sName[30]; int stdNo; int NoRegCourses; } student; typedef struct { char cName[30]; char cNo[10]; int maxRegNo; int currentRegNo; student sList[50]; }course; 2. Your program should use some of string functions, e.g. strlen, strtok. 3. Hint: to convert a string to integer you may use the following function atoi(). Example: int val; char str[20]; strcpy(str, "116987"); val = atoi(str); printf("String value = %s value = %d\n", str, val); What to Do: 1. Read the offered courses from the course file into a linked list of type course. 2. Read the students from the student file into another linked list , after that add the student to the required courses in an array of linked lists .Before adding a student to any course, you have to make sure that: a. The course is offered by the department (i.e. is available in the course list). For example, Ashraf Hasan want to register COMP333, but it is not offered by the department. b. The student can register for at most 5 courses each semester. c. Number of registered students in each course should not exceed the maximum number of students. For example, if 23 students want to register COMP336, then just the first 15 students should be added to this course. d. Show relevant message in case the student can not register a course . e.g closed course, course not found , the student registered 5 courses . 3. After that, for each course print the list of students. Note the code in C not in c++
Write C program language
Department of computer science offers a set of courses each
semester. These courses are listed in a file as follows: course
name, course id, number of registered student, and max number
of students. Number of registered students is initialized to zero
always. The file that contains the courses’ information is formatted
as follows (i.e. sample course file): Each semester, students are
required to register a set of courses. The students are listed in a
file along with the courses to be registered as follows: student
name, student id, courses to be registered (COMP242,
COMP336, COMP231...). The file that contains the students’
information is formatted as follows (i.e. sample student file):
Course File
Java#COMP231#0#27 Data Structures #COMP2321#0#30
Student File
Ahmad
Ali#1159999#COMP242#COMP336#COMP231#COMP338 Ali
Mahmoud#1169999#COMP142#COMP336#COMP231
Ashraf Hasan
#1139999#COMP142#COMP336#COMP231#COMP333#COMP
432#COMP338#COMP233
Programming Requirements:
1. Your program should use the concept of struct. Hint: create two
structs, one for course and the second for student.
typedef struct { char sName[30]; int stdNo; int NoRegCourses; }
student;
typedef struct { char cName[30]; char cNo[10]; int maxRegNo; int
currentRegNo; student sList[50]; }course; 2. Your program should
use some of string functions, e.g. strlen, strtok. 3. Hint: to convert
a string to integer you may use the following function atoi().
Example: int val; char str[20]; strcpy(str, "116987"); val = atoi(str);
printf("String value = %s value = %d\n", str, val); What to Do: 1.
Read the offered courses from the course file into a linked list of
type course. 2. Read the students from the student file into
another linked list ,
after that add the student to the required courses in an array of
linked lists .Before adding a student to any course, you have to
make sure that:
a. The course is offered by the department (i.e. is available in the
course list). For example, Ashraf Hasan want to register
COMP333, but it is not offered by the department.
b. The student can register for at most 5 courses each semester.
c. Number of registered students in each course should not
exceed the maximum number of students. For example, if 23
students want to register COMP336, then just the first 15 students
should be added to this course.
d. Show relevant message in case the student can not register a
course . e.g closed course, course not found , the student
registered 5 courses .
3. After that, for each course print the list of students.
Note the
code in C
not in c++

Trending now
This is a popular solution!
Step by step
Solved in 5 steps

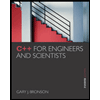
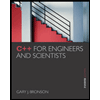