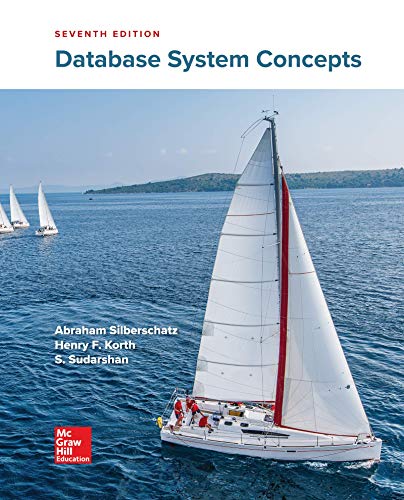
Concept explainers
Implement in C Programming
9.7.1: LAB: Course Grade
Write a program that reads the student information from a tab separated values (tsv) file. The program then creates a text file that records the course grades of the students. Each row of the tsv file contains the Last Name, First Name, Midterm1 score, Midterm2 score, and the Final score of a student. A sample of the student information is provided in StudentInfo.tsv. Assume the number of students is at least 1 and at most 20.
The program performs the following tasks:
- Read the file name of the tsv file from the user. Assume the file name has a maximum of 25 characters.
- Open the tsv file and read the student information. Assume each last name or first name has a maximum of 25 characters.
- Compute the average exam score of each student.
- Assign a letter grade to each student based on the average exam score in the following scale:
- A: 90 =< x
- B: 80 =< x < 90
- C: 70 =< x < 80
- D: 60 =< x < 70
- F: x < 60
- Compute the average of each exam.
- Output the last names, first names, exam scores, and letter grades of the students into a text file named report.txt. Output one student per row and separate the values with a tab character.
- Output the average of each exam, with two digits after the decimal point, at the end of report.txt. Hint: Use the precision sub-specifier to format the output.
Ex: If the input of the program is:
StudentInfo.tsv
and the contents of StudentInfo.tsv are:
Barrett Edan 70 45 59 Bradshaw Reagan 96 97 88 Charlton Caius 73 94 80 Mayo Tyrese 88 61 36 Stern Brenda 90 86 45
the file report.txt should contain:
Barrett Edan 70 45 59 F Bradshaw Reagan 96 97 88 A Charlton Caius 73 94 80 B Mayo Tyrese 88 61 36 D Stern Brenda 90 86 45 C Averages: midterm1 83.40, midterm2 76.60, final 61.60
#include <stdio.h>
int main(void) {
/* TODO: Declare any necessary variables here. */
/* TODO: Read a file name from the user and read the tsv file here. */
/* TODO: Compute student grades and exam averages, then output results to a text file here. */
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 4 images

- First programming homework Create a class of records for a gradebook called Rec. The data should be private, and should include a firstname, lastname, array of three grades, and a field for average grade. Build two constructors: a default constructor and a constructor that takes the first and last name. Build a function to read the data, either from a file or from cin. The read function reads the two names and three grades, but does not read the average grade. Build a write function that writes the data either to a file or to cout. The write function prints all of the data on one line with spaces between fields. Build a function to calculate the average grade field. Build an overloaded operator == to compare two records. This should be implemented as a friend function. Declare the constructors and functions in the body of the class, but implement them outside the class. I will provide you with a driver called hw1.cpp in ~cthorpe/public/142 and with a test file HW1.txt in the same…arrow_forwardPlease help with my C++Specifications • For the view and delete commands, display an error message if the user enters an invalid contact number. • Define a structure to store the data for each contact. • When you start the program, it should read the contacts from the tab-delimited text file and store them in a vector of contact objects. •When reading data from the text file, you can read all text up to the next tab by adding a tab character ('\t') as the third argument of the getline() function. •When you add or delete a contact, the change should be saved to the text file immediately. That way, no changes are lost, even if the program crashes laterarrow_forwardJava : Create a .txt file with 3 rows of various movies data of your choice in the following table format: Movie ID number of viewers rating release year Movie name 0000012211 174 8.4 2017 "Star Wars: The Last Jedi" 0000122110 369 7.9 2017 "Thor: Ragnarok" Create a class Movie which instantiates variables corresponds to the table columns. Implement getters, setters, constructors and toString. Implement 2 readData() methods : the first one will return an array of Movies and the second one will return a List of movies . Test your methods.arrow_forward
- createDatabaseOfProfiles(String filename) This method creates and populates the database array with the profiles from the input file (profile.txt) filename parameter. Each profile includes a persons' name and two DNA sequences. 1. Reads the number of profiles from the input file AND create the database array to hold that number profiles. 2. Reads the profiles from the input file. 3. For each person in the file 1. creates a Profile object with the information from file (see input file format below). 2. insert the newly created profile into the next position in the database array (instance variable).arrow_forwardComputer Science csv file "/dsa/data/all_datasets/texas.csv" Task 6: Write a function "county_locator" that allows a user to enter in a name of a spatial data frame and a county name and have a map generated that shows the location of that county with respect to other counties (by using different colors and/or symbols). Hint: function(); $county ==; plot(); add=TRUE.arrow_forwardDescriptionWrite a program to compute average grades for a course. The course records are in a single file and are organized according to the following format: each line contains a student's first name, then one space, then the student's last name, then one space, then some number of quiz scores that, if they exist, are separated by one space. Each student will have zero to ten scores, and each score is an integer not greater than 100. Your program will read data from this file and write its output to a second file. The date in the output file will be nearly the same as the data in the input file except that you will print the names as last-name, first-name; each quiz score, and there will be one additional number at the end of each line:the average of the student's ten quiz scores.Both files are parameters. You can access the name of the input file with argv[1]. and the name of the output file with argv[2].The output file must be formatted as described below: 1. First and last names…arrow_forward
- Write a program that uses a structure to store the following information on a company division: Division name (such as East, West, North, or South) Quarter (1, 2, 3, or 4)Quarterly salesThe user should be asked for the four quarters’ sales figures for the East, West, North, and South divisions. The information for each quarter for each division should be written to a file.arrow_forwardJAVA 8.13 LAB: Movie show time display Write a program that reads movie data from a csv (comma separated values) file and output the data in a formatted table. The program first reads the name of the CSV file from the user. The program then reads the csv file and outputs the contents according to the following requirements: Each row contains the title, rating, and all showtimes of a unique movie. A space is placed before and after each vertical separator (|) in each row. Column 1 displays the movie titles and is left justified with a minimum of 44 characters. If the movie title has more than 44 characters, output the first 44 characters only. Column 2 displays the movie ratings and is right justified with a minimum of 5 characters. Column 3 displays all the showtimes of the same movie, separated by a space. Each row of the csv file contains the showtime, title, and rating of a movie. Assume data of the same movie are grouped in consecutive rows. Ex: If the input of the program…arrow_forwardyou have been asked by a computer gaming company to create a role-playing game, commonly known as an rpg. the theme of this game will be of the user trying to get a treasure that is being guarded the user will choose a character from a list you create create a character class, in a file named character.h, which will: have the following variables: name race (chosen from a list that you create, like knight, wizard, elf, etc.) weapon (chosen from a list that you create) spells (true meaning has the power to cast spells on others) anything else you want to add the treasure will be hidden in one of the rooms in a castle create a castle class, in a file named castle.h, that will: have these variables: at least four rooms named room1, room2, etc. moat (which is a lagoon surrounding a castle) which boolean (not all castles have them) anything else you want to add create a default constructor for each class that initializes each argument to a blank or false value create a second…arrow_forward
- WAP to read 4 student objects and write them into a file, then print total number of student number in file. After that read an integer and print details of student stored at this number in the file using random access and file manipulators.arrow_forward1. Create a file that contains 20 integers or download the attached file twenty-integers.txttwenty-integers.txt reads:12 20 13 45 67 90 100 34 56 89 33 44 66 77 88 99 20 69 45 20 Create a program that: 2. Declares a c-style string array that will store a filename. 3. Prompts the user to enter a filename. Store the file name declared in the c-string. 4. Opens the file. Write code to check the file state. If the file fails to open, display a message and exit the program. 5. Declare an array that holds 20 integers. Read the 20 integers from the file into the array. 6. Write a function that accepts the filled array as a parameter and determines the MAXIMUM value in the array. Return the maximum value from the function (the function will be of type int). 7. Print ALL the array values AND print the maximum value in the array using a range-based for loop. Use informational messages. Ensure the output is readable.arrow_forwardC++ formatting please Write a program (not a function) that reads from a file named "data.csv". This file consists of a list of countries and gold medals they have won in various Olympic events. Write to standard out, the top 5 countries with the most gold medals. You can assume there will be no ties. Expected Output: 1: United States with 1022 gold medals 2: Soviet Union with 440 gold medals 3: Germany with 275 gold medals 4: Great Britain with 263 gold medals 5: China with 227 gold medalsarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
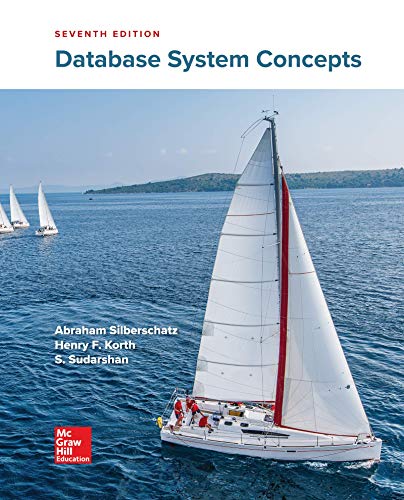
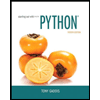
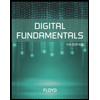
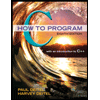
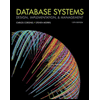
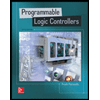