Write C++ Program to determine the max numbers between 2 arrays. For example: 6 3 1 34 2 011 20 Result 3 1 11 34 To resolve this problem, you should write 3 void functions: inputNumbers, testArrays and displayMaxArray. • inputNumbers: the aim of this function to read 10 numbers from the user and insert them in 2 different arrays (length of the array is 5). • testArrays: determine the max numbers between 2 arrays. • displayMaxArray: print the array containing the max numbers. Write C++ Program to check whether the Given String is a Palindrome. To resolve this problem, you should write 3 void functions: inputStrings, testPalindrome and displayNumbers. • inputStrings : the aim of this function to read 7 strings from the user and insert them in array of 7 elements. • testPalindrome: to check whether the given strings in the array are palindrome. • displayPalindrome: print the palindrome strings.
Write C++ Program to determine the max numbers between 2 arrays. For example: 6 3 1 34 2 011 20 Result 3 1 11 34 To resolve this problem, you should write 3 void functions: inputNumbers, testArrays and displayMaxArray. • inputNumbers: the aim of this function to read 10 numbers from the user and insert them in 2 different arrays (length of the array is 5). • testArrays: determine the max numbers between 2 arrays. • displayMaxArray: print the array containing the max numbers. Write C++ Program to check whether the Given String is a Palindrome. To resolve this problem, you should write 3 void functions: inputStrings, testPalindrome and displayNumbers. • inputStrings : the aim of this function to read 7 strings from the user and insert them in array of 7 elements. • testPalindrome: to check whether the given strings in the array are palindrome. • displayPalindrome: print the palindrome strings.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
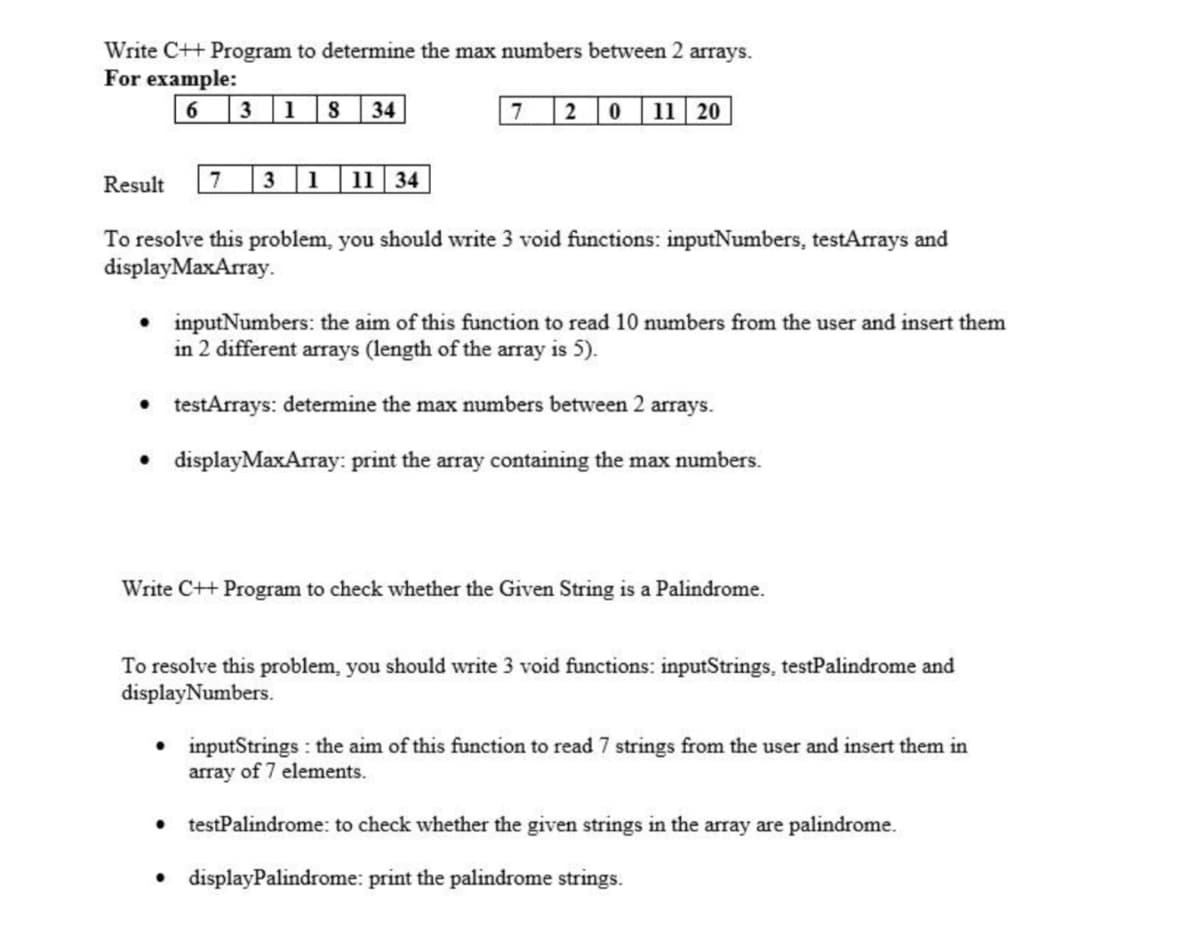
Transcribed Image Text:Write C++ Program to determine the max numbers between 2 arrays.
For example:
6.
1
34
7 2 0 11 20
Result
7
3 1
11 34
To resolve this problem, you should write 3 void functions: inputNumbers, testArrays and
displayMaxArray.
• inputNumbers: the aim of this function to read 10 numbers from the user and insert them
in 2 different arrays (length of the array is 5).
• testArrays: determine the max numbers between 2 arrays.
• displayMaxArray: print the array containing the max numbers.
Write C++ Program to check whether the Given String is a Palindrome.
To resolve this problem, you should write 3 void functions: inputStrings, testPalindrome and
displayNumbers.
• inputStrings : the aim of this function to read 7 strings from the user and insert them in
array of 7 elements.
• testPalindrome: to check whether the given strings in the array are palindrome.
displayPalindrome: print the palindrome strings.
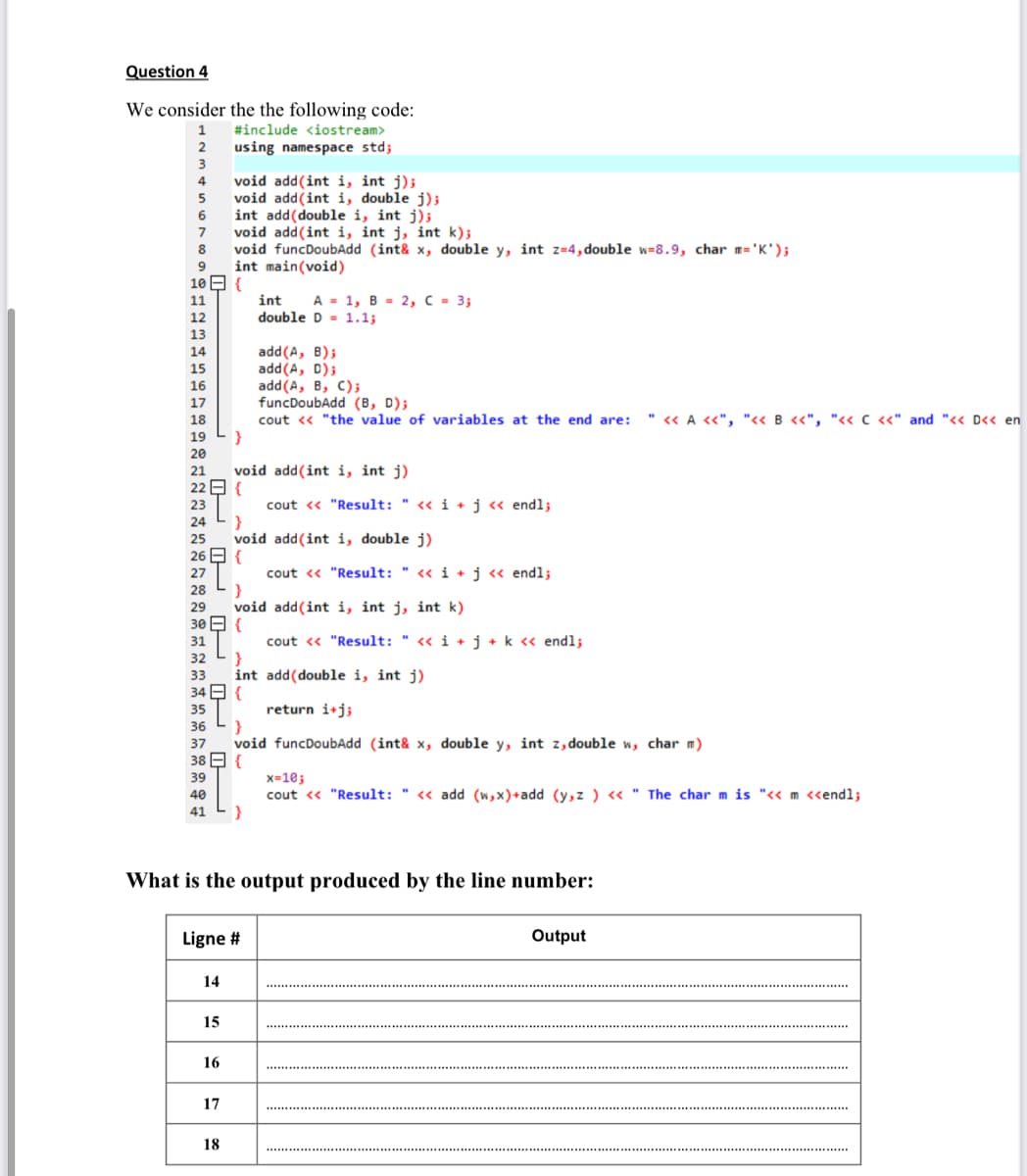
Transcribed Image Text:Question 4
We consider the the following code:
#include <iostream>
using namespace std;
void add(int i, int j);
void add(int i, double j);
int add(double i, int j);
void add(int i, int j, int k);
void funcDoubAdd (int& x, double y, int z=4, double w=8.9, char m='K');
int main(void)
10 E {
4
6.
7
11
int
А - 1, в - 2, с - 3;
12
double D = 1.1;
13
add(A, B);
add(A, D);
add(A, B, C);
funcDoubAdd (B, D);
14
15
16
17
18
cout <« "the value of variables at the end are:
" « A «", "« B «", "<« C «" and "« D<« en
19
}
20
void add(int i, int j)
22 E {
21
cout « "Result: " <« i +j« endl;
}
void add(int i, double j)
{
cout « "Result: " « i +j« endl;
23
24
25
26
27
28
void add(int i, int j, int k)
{
cout <« "Result: " <« i +j + k « endl;
}
int add(double i, int j)
34 E {
29
30
31
32
33
return i+j;
}
void funcDoubAdd (int& x, double y, int z, double w, char m)
{
x=10;
cout « "Result: " « add (w,x)+add (y,z ) « " The char m is "<< m «endl;
}
35
36
37
38
39
40
41
What is the output produced by the line number:
Ligne #
Output
14
15
16
17
18
O- O P P P
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Recommended textbooks for you
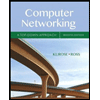
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
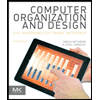
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
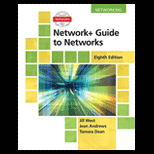
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
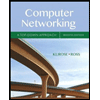
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
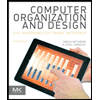
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
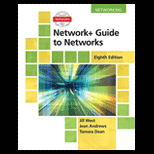
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
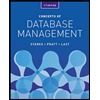
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
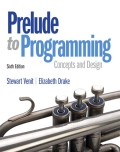
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
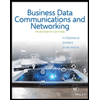
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY