Write exactly these 3 functions: power(x,y), print(text, number) function and the main() function. The power(x,y) function returns an integer result that is calculated by raising a number x (integer) to a power y (integer). The second argument y (exponent) of the function can not exceed 100. If the second argument exceeds 100, the function should return -1. Your power(x,y) function must be able to take either 1 or 2 integer arguments using the concept of default argument in chapter 6. Look at the main() code to deduct what the default value should be. You should call C++ pow(x,y) function in the "cmath" library in the body of your power(x,y) function to avoid doing the power calculation yourself. The print(text, number) function is the only one that does output to the console using "cout". Other functions should not use "cout". This function does not return anything. Write the 2 function prototypes above main() and write the full function definitions after main().
Topic: Function with default argument value covered in Chapter 6
Write a C++
Do not use any topic not covered in lecture. There is no need for use of loop, and needs the use of only an "if/else" statement.
Write exactly these 3 functions: power(x,y), print(text, number) function and the main() function.
The power(x,y) function returns an integer result that is calculated by raising a number x (integer) to a power y (integer). The second argument y (exponent) of the function can not exceed 100.
If the second argument exceeds 100, the function should return -1.
Your power(x,y) function must be able to take either 1 or 2 integer arguments using the concept of default argument in chapter 6. Look at the main() code to deduct what the default value should be.
You should call C++ pow(x,y) function in the "cmath" library in the body of your power(x,y) function to avoid doing the power calculation yourself.
The print(text, number) function is the only one that does output to the console using "cout". Other functions should not use "cout". This function does not return anything.
Write the 2 function prototypes above main() and write the full function definitions after main().
Use the following given main() code without any change.
/* Your code description
………
*/
// Function Prototypes go here before main
// ……….
// Two Function definitions go here after main
// ……………...
Use my main code exactly AS IS.
int main()
{
// Variable declaration
int x = 3;
// Driver statements
x = power(x, 3);
print("Value of x after step 1 is ", x);
x = power(x, 2);
print("Value of x after step 2 is ", x);
x = power(x);
print("Value of x after step 3 is ", x);
x = power(x, 101);
print("Value of x after step 4 is ", x);
x = power(3, -5);
print("Value of x after step 5 is ", x);
x = 10;
x = power(x);
print("Value of x after step 6 is ", x);
return 0;
}
Program output must look exactly like this using my given main code:
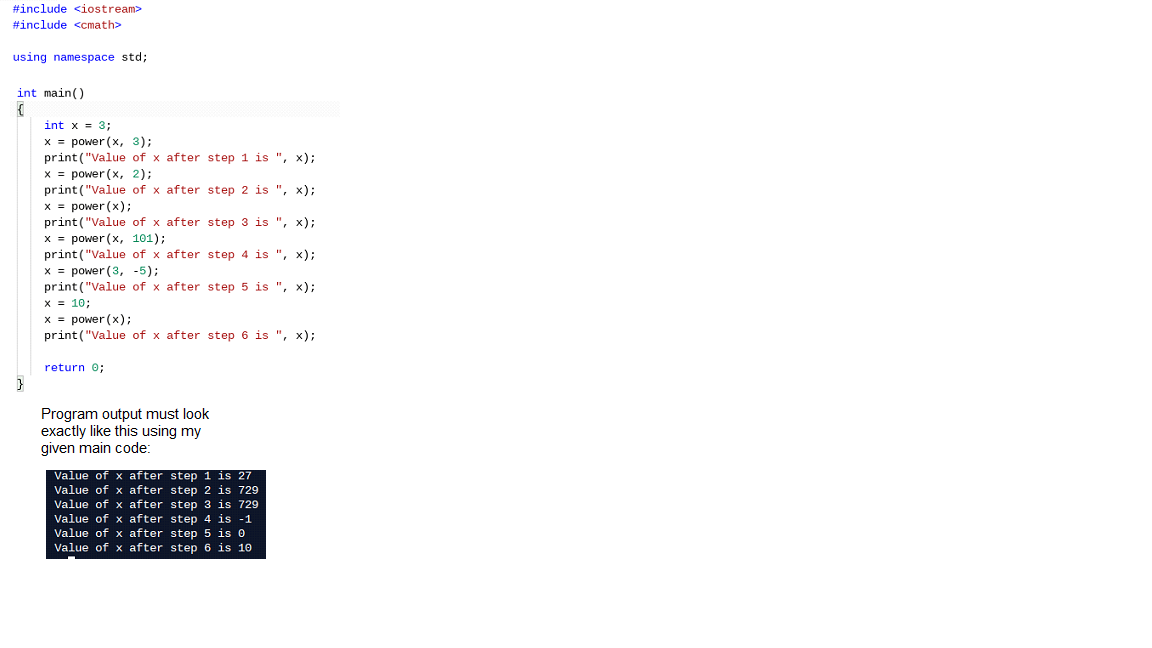

Step by step
Solved in 4 steps with 2 images

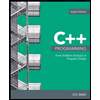
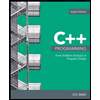