Write function is decompose. First you reduce the fraction. Then you return a Fraction that is one of three things: A) If we're an improper fraction (like 10/3), return a Fraction with the largest whole number in the fraction, in this case 3 (so you return {3,1}). B) If we're a proper fraction with a numerator of 1, return our values (i.e. return {num,den}) C) If we're a proper fraction with a numerator not 1, return the largest fraction with a numerator of 1 smaller than our value. (For example, if our numerator is 4 and denominator is 6, then we would return {1,2}, since 1/2 is the largest fraction with a numerator of 1 smaller than 4/6.) void reduce() { //YOU: Fix this code so it actually reduces the fraction //NOTE: The std::gcd() function might be useful // num /= 1; // den /= 1; for(int i = num * den; i > 1; i--) { if((den % i == 0) && (num % i) == 0) { den /= i; num /= i; } } } Fraction decompose() { reduce(); //Code continue solution fraction decompose } C++
Write function is decompose. First you reduce the fraction.
Then you return a Fraction that is one of three things:
A) If we're an improper fraction (like 10/3), return a Fraction with the
largest whole number in the fraction, in this case 3 (so you return {3,1}).
B) If we're a proper fraction with a numerator of 1, return our values (i.e.
return {num,den})
C) If we're a proper fraction with a numerator not 1, return the largest
fraction with a numerator of 1 smaller than our value. (For example, if our
numerator is 4 and denominator is 6, then we would return {1,2}, since 1/2 is
the largest fraction with a numerator of 1 smaller than 4/6.)
void reduce() {
//YOU: Fix this code so it actually reduces the fraction
//NOTE: The std::gcd() function might be useful
// num /= 1;
// den /= 1;
for(int i = num * den; i > 1; i--)
{
if((den % i == 0) && (num % i) == 0)
{
den /= i;
num /= i;
}
}
}
Fraction decompose() {
reduce();
//Code continue solution fraction decompose
}
C++

Step by step
Solved in 2 steps with 1 images

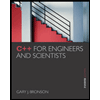
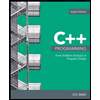
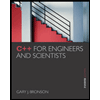
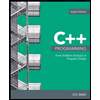