Write in c++ language: Your program will have a class called FlightController that is going to be derived from two base classes which are called LaunchController and LandingController. The FlightController class inherits all accessible data fields and methods from the LandingController and LaunchController classes. Inside the FlightController class, you will define an object of type FlightSchedule which will contain an array of 3 objects of type Flight. In your Flight objects, the constructor of these objects will ask from the user for the inputs of: flight name, launch hour, launch minutes, flight duration. The value of state of the Flight object will be assigned to “Idle” as a default value in the constructor also and you will call the member function within the Flight class which will calculate the landing time for your flight object by using the duration and the launch time. Definition of Flight class will also contain the necessary getters and setters that are given in the diagram and needed throughout your program. In the constructor of your FlightController you will need to define a pointer that will point to the adress of the FlightSchedule object you have created. You will send that pointer into the functions launchSchedule and landingSchedule in your base classes and these functions will perform a sorting operation between these three flight objects. The launchController will check the launch times as in hours and minutes of the array of objects that you have passed as a reference and will sort the array in ascending order. After the sorting you will output the launch order of these flights in order. Similar approach will be taken in the landingController and the landing order will be displayed as output after the sorting is complete. Outputs will consist of flight name, landing hour, landing minute, flight’s state and they will be printed from top down in order. Same thing will hold for both of the landing and launch controllers. Class definitions for both the landingController and the launchController must also contain all of the necessary members and variables in correct access specifiers and expected types. After successfully displaying the ordered ouputs for launches and landing , the program will end.
Write in c++ language:
Your program will have a class called FlightController that is going to be derived from two
base classes which are called LaunchController and LandingController. The FlightController
class inherits all accessible data fields and methods from the LandingController and
LaunchController classes.
Inside the FlightController class, you will define an object of type FlightSchedule which will
contain an array of 3 objects of type Flight. In your Flight objects, the constructor of these
objects will ask from the user for the inputs of: flight name, launch hour, launch minutes,
flight duration. The value of state of the Flight object will be assigned to “Idle” as a default
value in the constructor also and you will call the member function within the Flight class
which will calculate the landing time for your flight object by using the duration and the
launch time. Definition of Flight class will also contain the necessary getters and setters that
are given in the diagram and needed throughout your program.
In the constructor of your FlightController you will need to define a pointer that will point to
the adress of the FlightSchedule object you have created. You will send that pointer into the
functions launchSchedule and landingSchedule in your base classes and these functions will
perform a sorting operation between these three flight objects.
The launchController will check the launch times as in hours and minutes of the array of
objects that you have passed as a reference and will sort the array in ascending order. After
the sorting you will output the launch order of these flights in order. Similar approach will be
taken in the landingController and the landing order will be displayed as output after the
sorting is complete. Outputs will consist of flight name, landing hour, landing minute, flight’s
state and they will be printed from top down in order. Same thing will hold for both of the
landing and launch controllers.
Class definitions for both the landingController and the launchController must also contain all
of the necessary members and variables in correct access specifiers and expected types. After
successfully displaying the ordered ouputs for launches and landing , the program will end.
![LaunchController
LandingController
|- launchHour1: int
- launchHour2: int
- launchMin1: int
|- launchMin2: Int
- landHour1: int
- landHour2: int
- landMin1: int
|- landMin2: int
+ launchSchedule(FlightSchedule" ): void
+ landingSchedule(FlightSchedule" ): void
Extends
Extends
FlightController
# flight_schedule: FlightSchedule
FlightController()
+ flights: FlightSchedule"
Flight
- flightName: string
|- launchHour: int
- launchMin: int
|- landHour: int
|- landMin: int
|- duration: int
- state: string
FlightSchedule
+ flights]: Flight
+ method(type): type
+ getlaunchHour(): int
+ getlaunchMin((): int
+ getlandHour(): int
+ getlandMin(): int
+ getState(): string
+ setState(string): void
+ calculateLanding(): void](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ffe3adb89-d1ce-4f77-90a1-a91fd8b50f06%2F096963ea-c491-4c48-ac03-e5188c43e777%2Fbt57qwn_processed.png&w=3840&q=75)
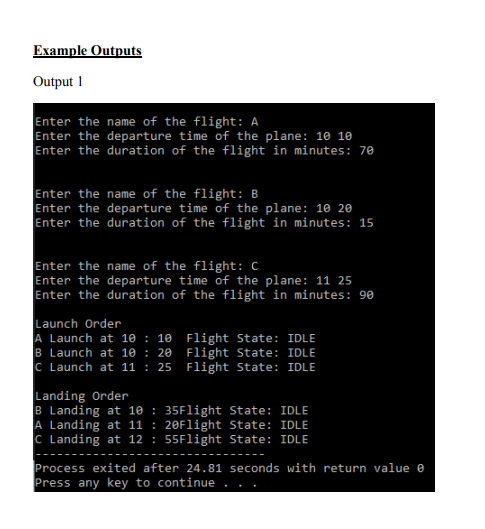

Step by step
Solved in 6 steps with 3 images

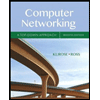
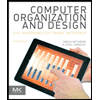
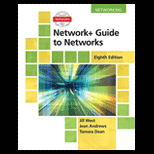
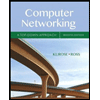
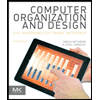
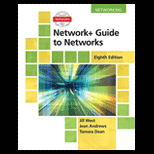
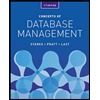
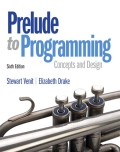
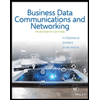