In this homework, you will implement an employee class which extends abstract Employee class (you can find and download this on the Labwork 5 page), you can add or modify existing functions but you cannot delete. You are asked to implement two classes, one should be a specific type of employee class, what type of employee it is going to be is totally up to you. You can implement a Software Engineer, Data Analyst, Art Director, Medical Doctor, Youtuber or Astronaut class, the limit is your imagination. You should also implement a Company class which is going to be your test class. In this class you will implement a main function that is going to present a company which your type of employees are working (e.g. a hospital if you have implemented a Medical Doctor class) and showcase their attributes and functions. You can implement a menu or give a hard-coded main function. You should also upload the Employee class even if you have not changed anything, hence you are supposed to upload 3 files. Requirements: Implement the work() function so that it prints out what the employee is currently doing and also whether the work is successful You should have different possible outputs for work() function and your function should print a random one, also randomizing the success of the work There are generated names inside the Employee you should make use of those when creating employees IDs of the employees should not be randomized, IDs should be assigned in the order of the creation of employees ( first created employee should have ID:1 the next ID:2 and so on) 5. You should add at least 2 additional non-trivial functions (meaning not getter setters or any other useless functions) and 2 attributes to your own employee class Your Company class should have at least 2 reasonable non-trivial functions and 2 attributes 7. Your main function in Company class should showcase all the functions and attributes you have implemented and should give an output that makes sense of the program you have written The more creative and intricate you are about the details of this labwork, the greater your grade is going to be! An example can be; This is a Graphic Design company. Our company name is Star Dust. There are currently 2 employees. Leon Mcdonald is working on a banner design and s/he is doing good. Frankie Johnson is working on a video editing project but s/he is not very successful. We are currently working on 5 projects. We have completed 32 projects in the past. We are currently looking for a Social Media Manager, who has at least 3 years of experience. Do not replicate this example, and please make your class more intricate than this one. public abstract class Employee { protected final String name; protected final int id; public Employee(String empName, int empId) { name = empName; id = empId; } public String getName() { return name; } public int getId() { return id; } //returns true if work was successful. otherwise returns false also prints out what the employee is doing and whether s/he is successful public abstract boolean work(); public String toString() { return name + " ID: " + id; } public boolean equals(Object other) { if (other instanceof Employee) { return equals((Employee)other); } return false; } public boolean equals(Employee other) { return other != null && name.equals(other.name) && id == other.id; } private static int lastGeneratedId = 0; private static String[] generatedNames = { "Leon Mcdonald", "Frankie Johnson", "Todd Rosenthal", "Mauricio Curran", "Randy Feinstein", "Donald Munoz", "Bonnie Barnhardt", "Gary Foley", "Brittney Wilson", "Lyndsay Loomis", "Madge Cartwright", "Stella Coan", "David Lafave", "Kimberly Matthews", "Dwayne Heckler", "Laura Payne", "Mary Luevano", "Walter Sizemore", "James Lawson", "Pat Nelson", "Sherry Leighton", "Anthony Tieman", "Lona Davis", "Ana Flores", "Richard Mcdonald", "Joseph Standley", "Nancy Eddy", "Joyce Shaw", "Philip Collings", "James Reay", "Barbara Acker", "Violet Cleary", "Maria Crawley", "Erin Hilton", "Evelyn Morales", "Leo Rose", "Dorothy Johnson", "Geoffrey Fogarty", "Jane Marin", "Daniel Tran", "Nancy Lee", "Peter Johnson", "Glenn Browning", "Mark Jaramillo", "Latina Gross", "Theresa Stutes", "George Thiel", "Robert Carney", "Janet Watts", "Michael English", "James Scott", "Elmer Honaker", "Brian Upton", "Dawne Miller", "Gretchen Bender", "John Carr", "Faith Gavin", "Traci Hill", "Joseph Miller", "Don Montoya", "Brandy Pritts", "Sandra Sheppard", "Charles Whitmer", "Ana Williams", "Elizabeth Murphy", "Michael Hollingsworth", "Claudine Dalton", "Harold Coleman", "Young Ayala", "Shelba Kirschbaum", "Tom Perez", "Amee Martin", "Maryanne Foote", "Sylvia Harrell", "Alexander Weibel", "Bruce Bailey", "Vincent Fidler", "Jack Wilbur", "Charles Pond", "Danielle Yocom", "John Kemp", "Jamie Casey", "Everett Frederick", "Emma Hazley", "Justin Lynch", "Tyler Miller", "Albert Reyes", "Wilbur Price", "Kimberly Halton", "Mary Underwood", "Raymond Garrett", "William Olive", "Joanne Smith", "Wilbert Howerton", "Selene Gross", "Jennie Andrews", "Jasper Barrows", "Robert Verdin", "Mark Mcallister", "Teri Morrissey" };
In this homework, you will implement an employee class which extends abstract Employee class (you can find and download this on the Labwork 5 page), you can add or modify existing functions but you cannot delete. You are asked to implement two classes, one should be a specific type of employee class, what type of employee it is going to be is totally up to you. You can implement a Software Engineer, Data Analyst, Art Director, Medical Doctor, Youtuber or Astronaut class, the limit is your imagination. You should also implement a Company class which is going to be your test class. In this class you will implement a main function that is going to present a company which your type of employees are working (e.g. a hospital if you have implemented a Medical Doctor class) and showcase their attributes and functions. You can implement a menu or give a hard-coded main function. You should also upload the Employee class even if you have not changed anything, hence you are supposed to upload 3 files.
Requirements:
- Implement the work() function so that it prints out what the employee is currently doing and also whether the work is successful
- You should have different possible outputs for work() function and your function should print a random one, also randomizing the success of the work
- There are generated names inside the Employee you should make use of those when creating employees
- IDs of the employees should not be randomized, IDs should be assigned in the order of the creation of employees ( first created employee should have ID:1 the next ID:2 and so on) 5. You should add at least 2 additional non-trivial functions (meaning not getter setters or any other useless functions) and 2 attributes to your own employee class
- Your Company class should have at least 2 reasonable non-trivial functions and 2 attributes 7. Your main function in Company class should showcase all the functions and attributes you have implemented and should give an output that makes sense of the program you have written
The more creative and intricate you are about the details of this labwork, the greater your grade is going to be!
An example can be;
This is a Graphic Design company.
Our company name is Star Dust.
There are currently 2 employees.
Leon Mcdonald is working on a banner design and s/he is doing good.
Frankie Johnson is working on a video editing project but s/he is not very successful.
We are currently working on 5 projects.
We have completed 32 projects in the past.
We are currently looking for a Social Media Manager, who has at least 3 years of experience.
Do not replicate this example, and please make your class more intricate than this one.
public abstract class Employee {
protected final String name;
protected final int id;
public Employee(String empName, int empId) {
name = empName;
id = empId;
}
public String getName() {
return name;
}
public int getId() {
return id;
}
//returns true if work was successful. otherwise returns false also prints out what the employee is doing and whether s/he is successful
public abstract boolean work();
public String toString() {
return name + " ID: " + id;
}
public boolean equals(Object other) {
if (other instanceof Employee) {
return equals((Employee)other);
}
return false;
}
public boolean equals(Employee other) {
return other != null && name.equals(other.name) && id == other.id;
}
private static int lastGeneratedId = 0;
private static String[] generatedNames = {
"Leon Mcdonald",
"Frankie Johnson",
"Todd Rosenthal",
"Mauricio Curran",
"Randy Feinstein",
"Donald Munoz",
"Bonnie Barnhardt",
"Gary Foley",
"Brittney Wilson",
"Lyndsay Loomis",
"Madge Cartwright",
"Stella Coan",
"David Lafave",
"Kimberly Matthews",
"Dwayne Heckler",
"Laura Payne",
"Mary Luevano",
"Walter Sizemore",
"James Lawson",
"Pat Nelson",
"Sherry Leighton",
"Anthony Tieman",
"Lona Davis",
"Ana Flores",
"Richard Mcdonald",
"Joseph Standley",
"Nancy Eddy",
"Joyce Shaw",
"Philip Collings",
"James Reay",
"Barbara Acker",
"Violet Cleary",
"Maria Crawley",
"Erin Hilton",
"Evelyn Morales",
"Leo Rose",
"Dorothy Johnson",
"Geoffrey Fogarty",
"Jane Marin",
"Daniel Tran",
"Nancy Lee",
"Peter Johnson",
"Glenn Browning",
"Mark Jaramillo",
"Latina Gross",
"Theresa Stutes",
"George Thiel",
"Robert Carney",
"Janet Watts",
"Michael English",
"James Scott",
"Elmer Honaker",
"Brian Upton",
"Dawne Miller",
"Gretchen Bender",
"John Carr",
"Faith Gavin",
"Traci Hill",
"Joseph Miller",
"Don Montoya",
"Brandy Pritts",
"Sandra Sheppard",
"Charles Whitmer",
"Ana Williams",
"Elizabeth Murphy",
"Michael Hollingsworth",
"Claudine Dalton",
"Harold Coleman",
"Young Ayala",
"Shelba Kirschbaum",
"Tom Perez",
"Amee Martin",
"Maryanne Foote",
"Sylvia Harrell",
"Alexander Weibel",
"Bruce Bailey",
"Vincent Fidler",
"Jack Wilbur",
"Charles Pond",
"Danielle Yocom",
"John Kemp",
"Jamie Casey",
"Everett Frederick",
"Emma Hazley",
"Justin Lynch",
"Tyler Miller",
"Albert Reyes",
"Wilbur Price",
"Kimberly Halton",
"Mary Underwood",
"Raymond Garrett",
"William Olive",
"Joanne Smith",
"Wilbert Howerton",
"Selene Gross",
"Jennie Andrews",
"Jasper Barrows",
"Robert Verdin",
"Mark Mcallister",
"Teri Morrissey"
};

Step by step
Solved in 2 steps

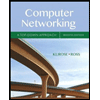
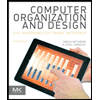
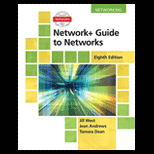
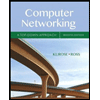
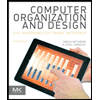
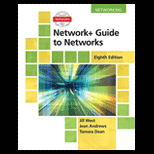
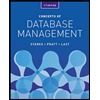
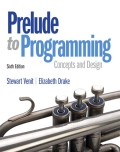
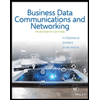