Write Java Classes please read what's asked and dont add unnecassary parts In this problem, you will create a shopping cart that will help a user keep track of bought items and their prices. To do so, you will first create an Item class, a ShoppingCart class and finally a driver class in which you will read items’ information from a text file and print the bill to the user. A. Item Class 1. The item class is defined by the following properties: a. Name. b. Price (Representing the unit price). c. Quantity (Representing the number of units). 2. Add a constructor that takes as argument the name, price and quantity of the food item. Initialize your variables accordingly. 3. You will need to retrieve all the properties of the class later on. Add the appropriate methods. 4. Add the following methods: a. A toString method that returns a properly formatted summary of the: name of the item, unit price (in U.S dollars) and the quantity as shown below: Bread 00.69$ * 3 b. A getTotalPrice method that returns the total price based on the items in the cart. B. Shopping Cart Class 1. The ShoppingCart class is defined by the following properties: a. An array of items to keep track of the items in the cart. b. Item count representing the number of items in the cart. c. Total Price representing the total price of cart based on the items. 2. Add a constructor that takes no arguments. Your constructor must initialize all your variables. Consider that the original capacity is 5 items. 3. Add the following method to your class: a. increaseSize: a method that increases the capacity of the cart by 3. The method must preserve the food items already stored. This method will only be called from within the ShoppingCart class. b. addToCart: a method that takes as argument an Item object and adds it to the cart. This method should also update all class variables accordingly upon the addition of an item. c. Since the item capacity is initialized to 5, you must make sure that the capacity is not full. If the cart is full, use the appropriate method to increase the size of the cart before adding the item. d. toString: the toString method should return a summary of the cart as shown in the sample below: Cart items: Bread 00.69 $ * 3 Cheese 01.24 $ * 2 Chicken 01.49 $ * 10 Total Price: 19.45 $
Write Java Classes please read what's asked and dont add unnecassary parts In this problem, you will create a shopping cart that will help a user keep track of bought items and their prices. To do so, you will first create an Item class, a ShoppingCart class and finally a driver class in which you will read items’ information from a text file and print the bill to the user. A. Item Class 1. The item class is defined by the following properties: a. Name. b. Price (Representing the unit price). c. Quantity (Representing the number of units). 2. Add a constructor that takes as argument the name, price and quantity of the food item. Initialize your variables accordingly. 3. You will need to retrieve all the properties of the class later on. Add the appropriate methods. 4. Add the following methods: a. A toString method that returns a properly formatted summary of the: name of the item, unit price (in U.S dollars) and the quantity as shown below: Bread 00.69$ * 3 b. A getTotalPrice method that returns the total price based on the items in the cart. B. Shopping Cart Class 1. The ShoppingCart class is defined by the following properties: a. An array of items to keep track of the items in the cart. b. Item count representing the number of items in the cart. c. Total Price representing the total price of cart based on the items. 2. Add a constructor that takes no arguments. Your constructor must initialize all your variables. Consider that the original capacity is 5 items. 3. Add the following method to your class: a. increaseSize: a method that increases the capacity of the cart by 3. The method must preserve the food items already stored. This method will only be called from within the ShoppingCart class. b. addToCart: a method that takes as argument an Item object and adds it to the cart. This method should also update all class variables accordingly upon the addition of an item. c. Since the item capacity is initialized to 5, you must make sure that the capacity is not full. If the cart is full, use the appropriate method to increase the size of the cart before adding the item. d. toString: the toString method should return a summary of the cart as shown in the sample below: Cart items: Bread 00.69 $ * 3 Cheese 01.24 $ * 2 Chicken 01.49 $ * 10 Total Price: 19.45 $
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Write Java Classes
please read what's asked and dont add unnecassary parts
In this problem, you will create a shopping cart that will help a user keep track of bought items and their prices. To do so, you will first create an Item class, a ShoppingCart class and finally a driver class in which you will read items’ information from a text file and print the bill to the user.
A. Item Class
1. The item class is defined by the following properties:
a. Name.
b. Price (Representing the unit price).
c. Quantity (Representing the number of units).
2. Add a constructor that takes as argument the name, price and quantity of the food item. Initialize your variables accordingly.
3. You will need to retrieve all the properties of the class later on. Add the appropriate methods.
4. Add the following methods:
a. A toString method that returns a properly formatted summary of the: name of the item, unit price (in U.S dollars) and the quantity as shown below:
Bread 00.69$ * 3
b. A getTotalPrice method that returns the total price based on the items in the cart.
B. Shopping Cart Class
1. The ShoppingCart class is defined by the following properties:
a. An array of items to keep track of the items in the cart.
b. Item count representing the number of items in the cart.
c. Total Price representing the total price of cart based on the items.
2. Add a constructor that takes no arguments. Your constructor must initialize all your variables. Consider that the original capacity is 5 items.
3. Add the following method to your class:
a. increaseSize: a method that increases the capacity of the cart by 3. The method must preserve the food items already stored. This method will only be called from within the ShoppingCart class.
b. addToCart: a method that takes as argument an Item object and adds it to the cart. This method should also update all class variables accordingly upon the addition of an item.
c. Since the item capacity is initialized to 5, you must make sure that the capacity is not full. If the cart is full, use the appropriate method to increase the size of the cart before adding the item.
d. toString: the toString method should return a summary of the cart as shown in the sample below:
Cart items:
Bread 00.69 $ * 3
Cheese 01.24 $ * 2
Chicken 01.49 $ * 10
Total Price: 19.45 $
C. Driver Class
1. Copy and paste the information below in a text file called “Items.txt”
Item Unit price ($) quantity (100 grams)
Bread 0.69 3
Cheese 1.24 2
Chicken 1.49 10
2. Using the information in the text file create a ShoppingCart of Items.
3. Print a summary of the cart for the user.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
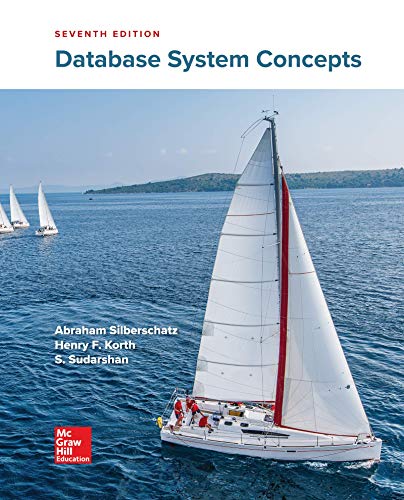
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
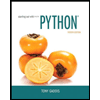
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
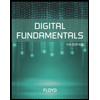
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
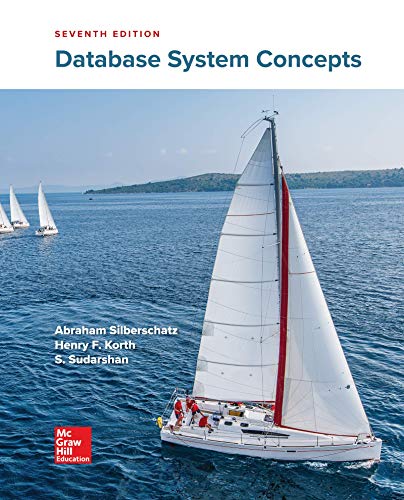
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
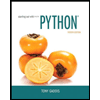
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
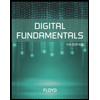
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
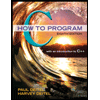
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
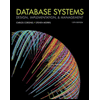
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
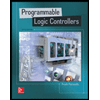
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education