Write the complete C-function void smallest(int data[], int size, int *small); finds the smallest value in d[] and return it via reference parameter. Show a call to this function in a calling function.
Write the complete C-function void smallest(int data[], int size, int *small); finds the smallest value in d[] and return it via reference parameter. Show a call to this function in a calling function.

Below is the C program with explanation: -
Explanation: -
- Including the essential header file.
- Defining the function void smallest(int data[], int size, int *small). This function will find the smallest number in the array by using the reference variable.
- Using the for-loop to iterate over the array.
- Inside the loop, the if-statement will check whether the value stored in the array is small then the value stored in the reference variable. The loop will check for each element and update the value of the reference variable. Finally, it will store the smallest value in the reference variable.
- The function will return the null value as it is a void function.
- Defining the main function.
- Declaring the variables as per the requirement.
- Filling the array by using the for-loop.
- Storing the first value of array in the reference variable.
- Calling the function void smallest() and pass the parameters.
- Using the reference variable displaying the smallest value present in the array.
C program: -
//header file
#include <stdio.h>
//defining the void function with parameters
void smallest(int data[], int size, int *small)
{
//using the for-loop to iterate over the array
for(int i =1; i<size; i++)
{
//to check whether the value present in the array is smaller than the value
//stored in the reference variable
if(*(data + i) < *small)
{
//storing the small value into the reference variable
*small = *(data+i);
}
}
//return statement
return ;
}
//main method
int main(void)
{
//declaring the variables
int data[100], *small, size;
//prompts the user to enter the number of elements he wants to store
printf("Enter the number of elements in array\n");
//storing the number in size variable
scanf("%d", &size);
//prompts the user to enter the integers
printf("Enter %d integers\n", size);
//using a for-loop to fill the array elements
for (int i = 0; i < size; i++)
{
//filling the array
scanf("%d", &data[i]);
}
//storing the first element of array in the small variable
small = data;
*small = *data;
//calling the function and passing the parameter
smallest(data, size,small);
//print the smallest number in the array by using reference variable
printf("The smallest number in the array is: %d",*small);
}
Step by step
Solved in 3 steps with 1 images

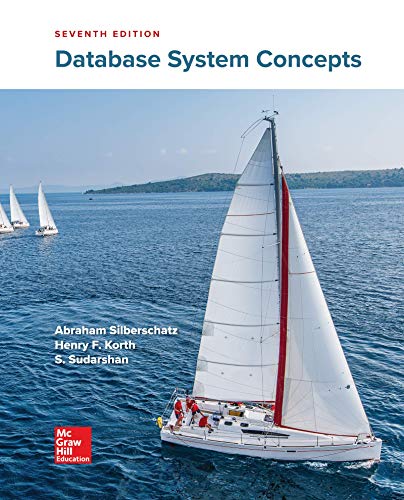
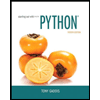
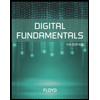
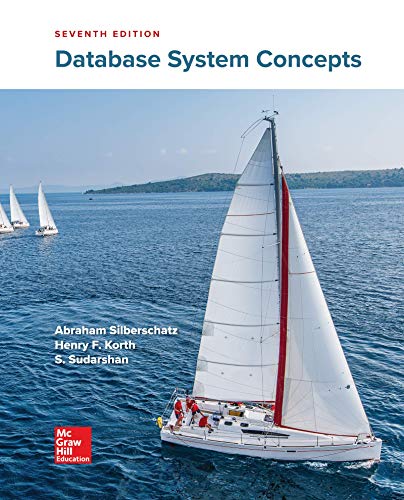
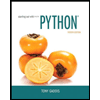
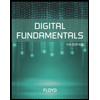
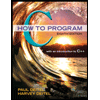
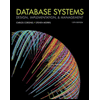
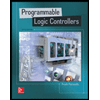