Write the PrintItem() function for the base class. Sample output for below program: Last name: Smith First and last name: Bill Jones Hint: Use the keyword const to make PrintItem() a virtual function. C++ program below, only lines 13-15 can be editted, the rest must stay the same. #include #include #include using namespace std; class BaseItem { public: void SetLastName(string providedName) { lastName = providedName; }; // FIXME: Define PrintItem() member function /* Your solution goes here */ protected: string lastName; }; class DerivedItem : public BaseItem { public: void SetFirstName(string providedName) { firstName = providedName; }; void PrintItem() const override { cout << "First and last name: "; cout << firstName << " " << lastName << endl; }; private: string firstName; }; int main() { BaseItem* baseItemPtr = nullptr; DerivedItem* derivedItemPtr = nullptr; vector itemList; unsigned int i; baseItemPtr = new BaseItem(); baseItemPtr->SetLastName("Smith"); derivedItemPtr = new DerivedItem(); derivedItemPtr->SetLastName("Jones"); derivedItemPtr->SetFirstName("Bill"); itemList.push_back(baseItemPtr); itemList.push_back(derivedItemPtr); for (i = 0; i < itemList.size(); ++i) { itemList.at(i)->PrintItem(); } return 0; }
Write the PrintItem() function for the base class. Sample output for below program:
Last name: Smith
First and last name: Bill Jones
Hint: Use the keyword const to make PrintItem() a virtual function.
C++ program below, only lines 13-15 can be editted, the rest must stay the same.
#include <iostream>
#include <string>
#include <
using namespace std;
class BaseItem {
public:
void SetLastName(string providedName) {
lastName = providedName;
};
// FIXME: Define PrintItem() member function
/* Your solution goes here */
protected:
string lastName;
};
class DerivedItem : public BaseItem {
public:
void SetFirstName(string providedName) {
firstName = providedName;
};
void PrintItem() const override {
cout << "First and last name: ";
cout << firstName << " " << lastName << endl;
};
private:
string firstName;
};
int main() {
BaseItem* baseItemPtr = nullptr;
DerivedItem* derivedItemPtr = nullptr;
vector<BaseItem*> itemList;
unsigned int i;
baseItemPtr = new BaseItem();
baseItemPtr->SetLastName("Smith");
derivedItemPtr = new DerivedItem();
derivedItemPtr->SetLastName("Jones");
derivedItemPtr->SetFirstName("Bill");
itemList.push_back(baseItemPtr);
itemList.push_back(derivedItemPtr);
for (i = 0; i < itemList.size(); ++i) {
itemList.at(i)->PrintItem();
}
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

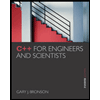
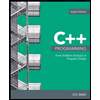
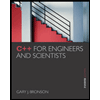
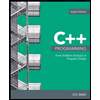