Write this code in C# language: Scenario: A dog shelter would like a simple system to keep track of all the dogs that pass through their facility. You are required to make a command line-based dog shelter management application. Your application must allow a user to add, edit, search and view Contacts. The program must have a menu consisting of the following six (6) options: Add dog View all dogs View all available dogs View specific dog Update dog home status Exit Brief descriptions of each option in the menu Menu Item Description Add Dog 1) Asks the user for all the state value information for a dog. 2) Checks if there is space in the array to hold the new dog. 3) Check that the dogId is not already used in the system. 4) Creates a new Dog object and adds it to the array if there is space. If the array is full, print “Sorry… the Shelter is Full!”. Note: All new dogs have no home as yet (foundHome = false). View all dogs This menu option shows all dogs in the system. This includes dogs that have a home and those that do not. View all available dogs Shows all dogs in the system, which have no homes as yet. View a specific dog 1) Asks the user for a dogId. 2) Searches for a dog at the shelter with that dogId. 3) Displays the information of the dog associated with that dogId if found. If the dog is not found, “There is no dog with that id..” must be displayed. Update dog home status 1) Asks the user for a dogId. 2) Searches for a dog at the shelter with that dogId 3) If a dog with that id is found, the “foundHome” status is changed to true and the dog information is to be displayed. If the dog is not found, the message “There is no dog with that id..” should be displayed. Exit 1) Prints a message “Thank you for using the Dog Shelter Management System.” 2) Exits the program Dog objects must be created to store the information on each dog at the shelter. The UML for the Dog object is given below Dog - dogId: int - name: string - age: int - breed: string - sex: char m for male, f for female -foundHome: boolean + getId() :int + getName(): string + getAge():int + getBreed() :string + getSex():char + getHomeStatus(): boolean + setHomeStatus(status:boolean):void + getInfo() :string A constructor must be written that takes all the state values (fields) listed in the Dog class. A list of behaviours the Dog class must have is given in the following table: Method Name Scope Behaviour getId() Public Returns the dogId of the dog getName() public Returns the name of the dog getAge() public Returns age of the dog getBreed() public Returns the breed of the dog getSex() public Returns the sex of the dog getHomeStatus() public Returns the “foundHome” status of the dog setHomeStatus(status: boolean) Public Set the “foundHome” status of the dog to the status parameter. toInfo() public Returns a string containing all the information about the dog. This assignment specifically tests the concepts of arrays and Objects Oriented Design basics. Restrictions for this assignment: You must create and manage all arrays with your own code. You may NOT use other C# collection objects (for example arraylist or list etc) Please do NOT change the specifications. For example, do not add new contact data. You must create and manage all arrays with your own code. OOP design hint: Your main program should NOT directly be responsible for maintaining or managing the array of Dogs. You must create another object to manage the Dogs for you. Please write the code in C# language
Write this code in C# language:
Scenario:
A dog shelter would like a simple system to keep track of all the dogs that pass through their facility. You are required to make a command line-based dog shelter management application. Your application must allow a user to add, edit, search and view Contacts.
The program must have a menu consisting of the following six (6) options:
- Add dog
- View all dogs
- View all available dogs
- View specific dog
- Update dog home status
- Exit
Brief descriptions of each option in the menu
Menu Item |
Description |
Add Dog
|
1) Asks the user for all the state value information for a dog. 2) Checks if there is space in the array to hold the new dog. 3) Check that the dogId is not already used in the system. 4) Creates a new Dog object and adds it to the array if there is space. If the array is full, print “Sorry… the Shelter is Full!”. Note: All new dogs have no home as yet (foundHome = false).
|
View all dogs
|
This menu option shows all dogs in the system. This includes dogs that have a home and those that do not.
|
View all available dogs |
Shows all dogs in the system, which have no homes as yet. |
View a specific dog
|
1) Asks the user for a dogId. 2) Searches for a dog at the shelter with that dogId. 3) Displays the information of the dog associated with that dogId if found. If the dog is not found, “There is no dog with that id..” must be displayed.
|
Update dog home status
|
1) Asks the user for a dogId. 2) Searches for a dog at the shelter with that dogId 3) If a dog with that id is found, the “foundHome” status is changed to true and the dog information is to be displayed. If the dog is not found, the message “There is no dog with that id..” should be displayed. |
Exit |
1) Prints a message “Thank you for using the Dog Shelter Management System.” 2) Exits the program |
Dog objects must be created to store the information on each dog at the shelter.
The UML for the Dog object is given below
Dog |
- dogId: int - name: string - age: int - breed: string - sex: char m for male, f for female -foundHome: boolean |
+ getId() :int + getName(): string + getAge():int + getBreed() :string + getSex():char + getHomeStatus(): boolean + setHomeStatus(status:boolean):void + getInfo() :string |
A constructor must be written that takes all the state values (fields) listed in the Dog class.
A list of behaviours the Dog class must have is given in the following table:
Method Name |
Scope |
Behaviour |
getId() |
Public |
Returns the dogId of the dog |
getName() |
public |
Returns the name of the dog |
getAge() |
public |
Returns age of the dog |
getBreed() |
public |
Returns the breed of the dog |
getSex() |
public |
Returns the sex of the dog |
getHomeStatus() |
public |
Returns the “foundHome” status of the dog |
setHomeStatus(status: boolean) |
Public |
Set the “foundHome” status of the dog to the status parameter. |
toInfo() |
public |
Returns a string containing all the information about the dog. |
This assignment specifically tests the concepts of arrays and Objects Oriented Design basics.
Restrictions for this assignment:
You must create and manage all arrays with your own code.
- You may NOT use other C# collection objects (for example arraylist or list<type> etc)
- Please do NOT change the specifications. For example, do not add new contact data.
- You must create and manage all arrays with your own code.
OOP design hint:
Your main program should NOT directly be responsible for maintaining or managing the array of Dogs. You must create another object to manage the Dogs for you.
Please write the code in C# language

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

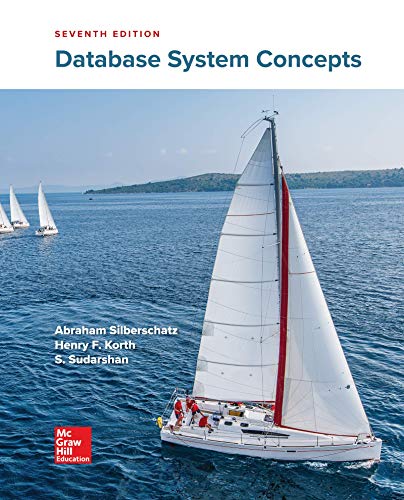
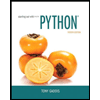
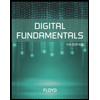
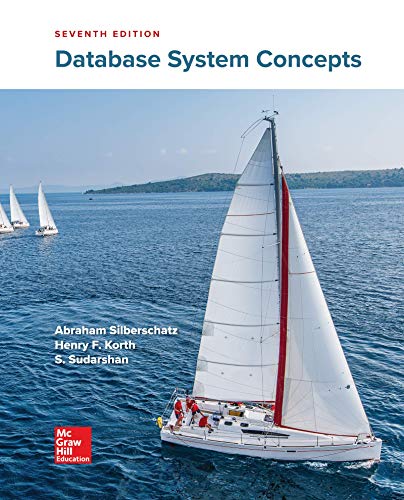
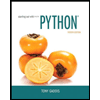
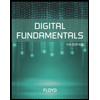
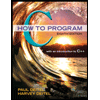
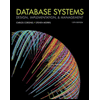
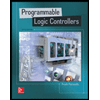