Write two local classes named Pump and Cylinder as described below. Then, write a main function that reads the flowSpeed and area of a Pump object (p) both as double values. main will also read the radius and height of a Cylinder object | (fuelTank) both as double values. Finally, the inbuiltPump of fuelTank will be the p Pump object. Finally, the program should calculate the time it takes the fuelTank to be completely filled with fuel and prints it to the screen as a double value. Pump Class A private double field named "flowSpeed" representing the flow speed of the pump. A private double field named "area" representing the cross-section area (i.e., kesit alan) of the pump. A constructor that gets two double values and initializes the object. An empty constructor. A public function named calculateflowRate that does not get any parameter, multiplies the flowspeed and area of the pump, and returns it as a double value. Cylinder Class A private double field named "radius" representing the radius of the cylinder. A private double field named "height" representing the height of the cylinder. A private Pump field named "inBuiltPump" representing the pump that provides fuel to this cylinder. A constructor that gets two double values, a Pump value, and initializes the object. A public function named calculateVolume that does not get any parameter, calculates the volume of the cylinder using the cylinder volume formula given below and returns it as a double value: Volume PI"radius"radius height A public function named calculateTimeToFill that does not get any parameter, calculates the time it takes for the cylinder to be filled with fuel from the pump by dividing the volume of the cylinder by the flow rate of the pump and returns it as a double value. The main function will, Instantiate one Pump object called p and instantiate a Cylinder object called fuelTank as explained above. Use the calculateTimeToFill method of the Cylinder class to print out the time it takes to fill the cylinder and print it out. NOTE: flowSpeed and area will always be given as positive values. NOTE: All the functions MUST only do the tasks described above, nothing else. NOTE: Both classes MUST be written as described. NOTE: To calculate PI, you MUST use the M_PI constant from the cmath library. Input 1564 2578 3 5 1 12 Output 90.4779 123.15 2.51327
Write two local classes named Pump and Cylinder as described below. Then, write a main function that reads the flowSpeed and area of a Pump object (p) both as double values. main will also read the radius and height of a Cylinder object | (fuelTank) both as double values. Finally, the inbuiltPump of fuelTank will be the p Pump object. Finally, the program should calculate the time it takes the fuelTank to be completely filled with fuel and prints it to the screen as a double value. Pump Class A private double field named "flowSpeed" representing the flow speed of the pump. A private double field named "area" representing the cross-section area (i.e., kesit alan) of the pump. A constructor that gets two double values and initializes the object. An empty constructor. A public function named calculateflowRate that does not get any parameter, multiplies the flowspeed and area of the pump, and returns it as a double value. Cylinder Class A private double field named "radius" representing the radius of the cylinder. A private double field named "height" representing the height of the cylinder. A private Pump field named "inBuiltPump" representing the pump that provides fuel to this cylinder. A constructor that gets two double values, a Pump value, and initializes the object. A public function named calculateVolume that does not get any parameter, calculates the volume of the cylinder using the cylinder volume formula given below and returns it as a double value: Volume PI"radius"radius height A public function named calculateTimeToFill that does not get any parameter, calculates the time it takes for the cylinder to be filled with fuel from the pump by dividing the volume of the cylinder by the flow rate of the pump and returns it as a double value. The main function will, Instantiate one Pump object called p and instantiate a Cylinder object called fuelTank as explained above. Use the calculateTimeToFill method of the Cylinder class to print out the time it takes to fill the cylinder and print it out. NOTE: flowSpeed and area will always be given as positive values. NOTE: All the functions MUST only do the tasks described above, nothing else. NOTE: Both classes MUST be written as described. NOTE: To calculate PI, you MUST use the M_PI constant from the cmath library. Input 1564 2578 3 5 1 12 Output 90.4779 123.15 2.51327
Programming Logic & Design Comprehensive
9th Edition
ISBN:9781337669405
Author:FARRELL
Publisher:FARRELL
Chapter11: More Object-oriented Programming Concepts
Section: Chapter Questions
Problem 1GZ
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question

Transcribed Image Text:Write two local classes named Pump and Cylinder as described below. Then, write a main function that reads the flowSpeed and area of a Pump object (p) both as double values. main will also read the radius and height of a Cylinder object
| (fuelTank) both as double values. Finally, the inBuiltPump of fuelTank will be the p Pump object. Finally, the program should calculate the time it takes the fuelTank to be completely filled with fuel and prints it to the screen as a double value.
Pump Class
A private double field named "flowSpeed" representing the flow speed of the pump.
A private double field named "area" representing the cross-section area (i.e., kesit alan) of the pump.
A constructor that gets two double values and initializes the object.
An empty constructor.
A public function named calculateFlowRate that does not get any parameter, multiplies the flowSpeed and area of the pump, and returns it as a double value.
Cylinder Class
A private double field named "radius" representing the radius of the cylinder.
A private double field named "height" representing the height of the cylinder.
A private Pump field named "inBuiltPump" representing the pump that provides fuel to this cylinder.
A constructor that gets two double values, a Pump value, and initializes the object.
A public function named calculateVolume that does not get any parameter, calculates the volume of the cylinder using the cylinder volume formula given below and returns it as a double value:
Volume = PI*radius*radius*height
A public function named calculateTimeToFill that does not get any parameter, calculates the time it takes for the cylinder to be filled with fuel from the pump by dividing the volume of the cylinder by the flow rate of the pump and returns it as a double value.
The main function will,
Instantiate one Pump object called p and instantiate a Cylinder object called fuelTank as explained above.
Use the calculateTimeToFill method of the Cylinder class to print out the time it takes to fill the cylinder and print it out.
NOTE: flowSpeed and area will always be given as positive values.
NOTE: All the functions MUST only do the tasks described above, nothing else.
Both classes MUST be written as described
NOTE: To calculate PI, you MUST use the M_PI constant from the cmath library.
Input
1564
2 5 7 8
3 5 1 12
Output
90.4779
123.15
2.51327
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
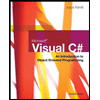
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
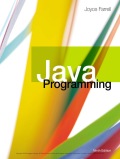
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
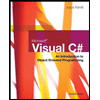
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
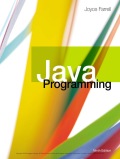
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
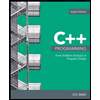
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning