You are first tasked with developing a search algorithm to search for a student by their ID in an array of integers. The read function in main.cpp reads the student IDs from students.txt in an array. Note that the entire array need not be updated. The index up to which the array is updated is returned by the function and stored in a variable called num_students. A research team has decided that a Ternary search algorithm works best. The algorithm is as follows: 1. Let low = 0, high = size-1; let mid1 low + (high-low)/3 and mid2 high - (high-low)/3 2. If A[mid1] is the element, return mid1; If A[mid2] is the element, return mid2 3. If element < A[mid1], perform ternary search from low to mid1 4. If element > A[mid1] and element < A[mid2], perform ternary search from mid1 to mid2 5. If element > A[mid2], perform ternary search from mid2 to high Implement the search algorithm by defining the function ternarySearch declared in main.cpp. Complete the rest of the main function to accept the student's ID as input and search for the student. Output the index of the student if found, else output a message that the student was not found. Task 2: Add one student Implement an insertion algorithm to insert a new student into the sorted array by defining the function addStudent declared in main.cpp.
You are first tasked with developing a search algorithm to search for a student by their ID in an array of integers. The read function in main.cpp reads the student IDs from students.txt in an array. Note that the entire array need not be updated. The index up to which the array is updated is returned by the function and stored in a variable called num_students. A research team has decided that a Ternary search algorithm works best. The algorithm is as follows: 1. Let low = 0, high = size-1; let mid1 low + (high-low)/3 and mid2 high - (high-low)/3 2. If A[mid1] is the element, return mid1; If A[mid2] is the element, return mid2 3. If element < A[mid1], perform ternary search from low to mid1 4. If element > A[mid1] and element < A[mid2], perform ternary search from mid1 to mid2 5. If element > A[mid2], perform ternary search from mid2 to high Implement the search algorithm by defining the function ternarySearch declared in main.cpp. Complete the rest of the main function to accept the student's ID as input and search for the student. Output the index of the student if found, else output a message that the student was not found. Task 2: Add one student Implement an insertion algorithm to insert a new student into the sorted array by defining the function addStudent declared in main.cpp.
Chapter3: Data Representation
Section: Chapter Questions
Problem 12VE
Related questions
Question
1300030051
1300308467
1300606334
1200906550
1201809169
1206505724
1301801478
1302109358
1202406962
1202704464
1203005705
1203308145
1302603281
1203903827
1304209961
1304500491
1304702995
1305101942
1306404827
1205705436
1206002391
1206304604
1206603902
1206900153
1207200292
1207502382
1207807421
1208108716
1208409718
1208709895
![You are first tasked with developing a search algorithm to search for a student by their ID in an array of
integers. The read function in main.cpp reads the student IDs from students.txt in an array. Note
that the entire array need not be updated. The index up to which the array is updated is returned by the
function and stored in a variable called num_students.
A research team has decided that a Ternary search algorithm works best. The algorithm is as follows:
1. Let low = 0, high = size-1; let mid1 + low + (high-low)/3 and mid2 + high - (high-low)/3
2. If A[mid1] is the element, return mid1; If A[mid2] is the element, return mid2
3. If element < A[mid1], perform ternary search from low to mid1
4. If element > A[mid1] and element < A[mid2], perform ternary search from mid1 to mid2
5. If element > A[mid2], perform ternary search from mid2 to high
Implement the search algorithm by defining the function ternarySearch declared in main.cpp.
Complete the rest of the main function to accept the student's ID as input and search for the student.
Output the index of the student if found, else output a message that the student was not found.
Task 2: Add one student
Implement an insertion algorithm to insert a new student into the sorted array by defining the function
addStudent declared in main.cpp.
Hint: You may reuse parts of the ternary search algorithm to find the location to insert the student.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fdb9f5d8b-0036-4232-a7c4-4e95eadce252%2Fe4ad62b0-d996-4e26-bc3b-d9ca5ecf995f%2Fc1h7tlm_processed.jpeg&w=3840&q=75)
Transcribed Image Text:You are first tasked with developing a search algorithm to search for a student by their ID in an array of
integers. The read function in main.cpp reads the student IDs from students.txt in an array. Note
that the entire array need not be updated. The index up to which the array is updated is returned by the
function and stored in a variable called num_students.
A research team has decided that a Ternary search algorithm works best. The algorithm is as follows:
1. Let low = 0, high = size-1; let mid1 + low + (high-low)/3 and mid2 + high - (high-low)/3
2. If A[mid1] is the element, return mid1; If A[mid2] is the element, return mid2
3. If element < A[mid1], perform ternary search from low to mid1
4. If element > A[mid1] and element < A[mid2], perform ternary search from mid1 to mid2
5. If element > A[mid2], perform ternary search from mid2 to high
Implement the search algorithm by defining the function ternarySearch declared in main.cpp.
Complete the rest of the main function to accept the student's ID as input and search for the student.
Output the index of the student if found, else output a message that the student was not found.
Task 2: Add one student
Implement an insertion algorithm to insert a new student into the sorted array by defining the function
addStudent declared in main.cpp.
Hint: You may reuse parts of the ternary search algorithm to find the location to insert the student.
![#include <iostream>
#include <fstream>
using namespace std;
#define MAXSTUDENTS 64
Fint read(string filename, int* students) {
ifstream fin(filename);
int index = 0;
while (!fin.eof() && index < MAXSTUDENTS) {
fin >> students[index++];
}
return index;
int ternarySearch(int* students, int element, int num_students);
void addStudent (int student, int* students);
// void addStudents(int* newstudents, int* students); // Uncomment to implement bonus
Fint main() {
int* students = new int[MAXSTUDENTS];
int num_students read("students.txt", students);
******/
* TODO: Implement your code here:
* 1. Take user input - student ID
* 2. Search for the student using ternarySearch
* 3. Take user input to add student to the list
* 4. Add the student using addStudent
**
/*本
delete[] students;
return e;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fdb9f5d8b-0036-4232-a7c4-4e95eadce252%2Fe4ad62b0-d996-4e26-bc3b-d9ca5ecf995f%2Fzidpwni_processed.jpeg&w=3840&q=75)
Transcribed Image Text:#include <iostream>
#include <fstream>
using namespace std;
#define MAXSTUDENTS 64
Fint read(string filename, int* students) {
ifstream fin(filename);
int index = 0;
while (!fin.eof() && index < MAXSTUDENTS) {
fin >> students[index++];
}
return index;
int ternarySearch(int* students, int element, int num_students);
void addStudent (int student, int* students);
// void addStudents(int* newstudents, int* students); // Uncomment to implement bonus
Fint main() {
int* students = new int[MAXSTUDENTS];
int num_students read("students.txt", students);
******/
* TODO: Implement your code here:
* 1. Take user input - student ID
* 2. Search for the student using ternarySearch
* 3. Take user input to add student to the list
* 4. Add the student using addStudent
**
/*本
delete[] students;
return e;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
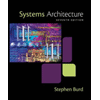
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning
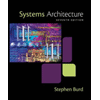
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning