Please add file handling to this given C++ code below so that it can store the input of the user:
Please add file handling to this given C++ code below so that it can store the input of the user:
#include<iostream>
#include<fstream>
#include<cctype>
#include<sstream>
#include<string>
using namespace std;
bool check = true;
struct node //structure of node //
{
int rollNo;
char name[20];
char address[40];
char gender[20];
char department[20];
int year;
char dob[20];
node *next;
}*head,*lastptr;
void add() //Adds record of student//
{
node *p;
p=new node;
cout<<"Enter ID number of student:"<<endl;
cin>>p->rollNo;
fflush(stdin);
cout<<"Enter name of student:"<<endl;
gets(p->name);
fflush(stdin);
cout<<"Enter address of student:"<<endl;
gets(p->address);
fflush(stdin);
cout<<"Enter birthday of student:"<<endl;
gets(p->dob);
fflush(stdin);
cout<<"Enter gender of student:"<<endl;
gets(p->gender);
fflush(stdin);
cout<<"Enter department of student:"<<endl;
gets(p->department);
fflush(stdin);
cout<<"Enter Training year of student:"<<endl;
cin>>p->year;
fflush(stdin);
p->next=NULL;
if(check)
{
head = p;
lastptr = p;
check = false;
}
else
{
lastptr->next=p;
lastptr=p;
}
cout<<endl<<"Recored Entered";
}
void modify() //modifies record of student//
{
node *ptr;
node *prev=NULL;
node *current=NULL;
int roll_no;
cout<<"Enter ID number to search:"<<endl;
cin>>roll_no;
prev=head;
current=head;
while(current->rollNo!=roll_no)
{
prev=current;
current=current->next;
}
ptr=new node;
fflush(stdin);
cout<<"Enter ID number of student:"<<endl;
cin>>ptr->rollNo;
fflush(stdin);
cout<<"Enter name of student:"<<endl;
gets(ptr->name);
fflush(stdin);
cout<<"Enter address of student:"<<endl;
gets(ptr->address);
fflush(stdin);
cout<<"Enter birthday of student:"<<endl;
gets(ptr->dob);
fflush(stdin);
cout<<"Enter gender of student:"<<endl;
gets(ptr->gender);
fflush(stdin);
cout<<"Enter department of student:"<<endl;
gets(ptr->department);
fflush(stdin);
cout<<"Enter Training year of student:"<<endl;
cin>>ptr->year;
fflush(stdin);
prev->next=ptr;
ptr->next=current->next;
current->next=NULL;
delete current;
cout<<endl<<"Recored Modified";
}
void search() //searches record of student//
{
node *prev=NULL;
node *current=NULL;
int roll_no;
cout<<"Enter ID Number to search:"<<endl;
cin>>roll_no;
prev=head;
current=head;
while(current->rollNo!=roll_no)
{
prev=current;
current=current->next;
}
cout<<"\nID No:";
cout<<current->rollNo;
cout<<"\nname: ";
puts(current->name);
cout<<"\nAddress: ";
puts(current->address);
cout<<"\nGender: ";
puts(current->gender);
cout<<"\ndepartment: ";
puts(current->department);
cout<<"\nYear: ";
cout<<current->year;
cout<<"\nDate of birth: ";
puts(current->dob);
}
void del()
{
node *ptr=NULL;
node *prev=NULL;
node *current=NULL;
int roll_no;
cout<<"Enter ID Number to Delete:"<<endl;
cin>>roll_no;
prev=head;
current=head;
while(current->rollNo!=roll_no)
{
prev=current;
current=current->next;
}
prev->next = current->next;
current->next=NULL;
delete current;
cout<<endl<<"Recored Deleted";
}
int main()
{
int x;
do
{
cout<<"1--->Press '1' to add New record:"<<endl;
cout<<"2--->Press '2' to search a record:"<<endl;
cout<<"3--->Press '3' to modify a record:"<<endl;
cout<<"4--->Press '4' to delete a record:"<<endl;
cout<<"5--->Press '5' to exit:"<<endl;
cin>>x;
switch(x){
case 1:
system("cls");
add();
break;
case 2:
system("cls");
search();
break;
case 3:
system("cls");
modify();
break;
case 4:
system("cls");
del();
break;
case 5:
exit(0);
break;
default:
cout<<"Enter a valid option. \n";
break;
}
}while(1);
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

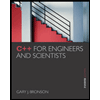
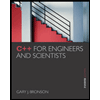