You are tasked with creating a simple game where players can choose to be either a Wizard or a Warrior and engage in a battle. A player's object will store their name, health points, and damage points. A Wizard object can use magic spells to deal damage, while a Warrior object can use their weapon to deal damage. Create a class named Player which has the following data members and member functions: • Data Members: name (string) • health (int) • damage (int) • Member Functions: • Player (string name, int health, int damage) - constructor that sets the data members to the given values • void attack (Player* opponent) - deals damage to the opponent based on the player's damage points • void takeDamage (int damage) - reduces the player's health by the given amount Create two subclasses of Player named Wizard and Warrior which inherit from player. Both Wizard and Warrior have an additional data member: • Data Members: mana (int) - available only for Wizard • weapon (string) - available only for Warrior Wizard has the following additional member function: • Member Functions: • void castSpell (Player* opponent) - deals damage to the opponent based on the player's mana points Warrior has the following additional member function: • Member Functions: • void swingWeapon (Player* opponent) - deals damage to the opponent based on the player's weapon's damage points In addition to the above, create getters and setters for all the data members (get Health, setHealth,... etc) Implement the member functions of the Player, Wizard, and Warrior classes. In the attack function of the player class, you should output a message describing attack a damage dealt to the opponent. In the takeDamage function, output a message describing how much damage the player took and their remaining health points. Create a main function named main-1-1.cpp, which creates one instance of a Wizard and one instance of a Warrior. The program should then simulate a battle between the two players. The battle should consist of the players taking turns attacking each other until one of them runs out of health points. After each attack, output a message describing the attack and the resulting damage dealt. At the end of the battle, output a message declaring the winner.
You are tasked with creating a simple game where players can choose to be either a Wizard or a Warrior and engage in a battle. A player's object will store their name, health points, and damage points. A Wizard object can use magic spells to deal damage, while a Warrior object can use their weapon to deal damage. Create a class named Player which has the following data members and member functions: • Data Members: name (string) • health (int) • damage (int) • Member Functions: • Player (string name, int health, int damage) - constructor that sets the data members to the given values • void attack (Player* opponent) - deals damage to the opponent based on the player's damage points • void takeDamage (int damage) - reduces the player's health by the given amount Create two subclasses of Player named Wizard and Warrior which inherit from player. Both Wizard and Warrior have an additional data member: • Data Members: mana (int) - available only for Wizard • weapon (string) - available only for Warrior Wizard has the following additional member function: • Member Functions: • void castSpell (Player* opponent) - deals damage to the opponent based on the player's mana points Warrior has the following additional member function: • Member Functions: • void swingWeapon (Player* opponent) - deals damage to the opponent based on the player's weapon's damage points In addition to the above, create getters and setters for all the data members (get Health, setHealth,... etc) Implement the member functions of the Player, Wizard, and Warrior classes. In the attack function of the player class, you should output a message describing attack a damage dealt to the opponent. In the takeDamage function, output a message describing how much damage the player took and their remaining health points. Create a main function named main-1-1.cpp, which creates one instance of a Wizard and one instance of a Warrior. The program should then simulate a battle between the two players. The battle should consist of the players taking turns attacking each other until one of them runs out of health points. After each attack, output a message describing the attack and the resulting damage dealt. At the end of the battle, output a message declaring the winner.
Chapter10: Introduction To Inheritance
Section: Chapter Questions
Problem 1GZ
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
Please code in c++ without using namespace std and please add comments in the code and Thank you :)
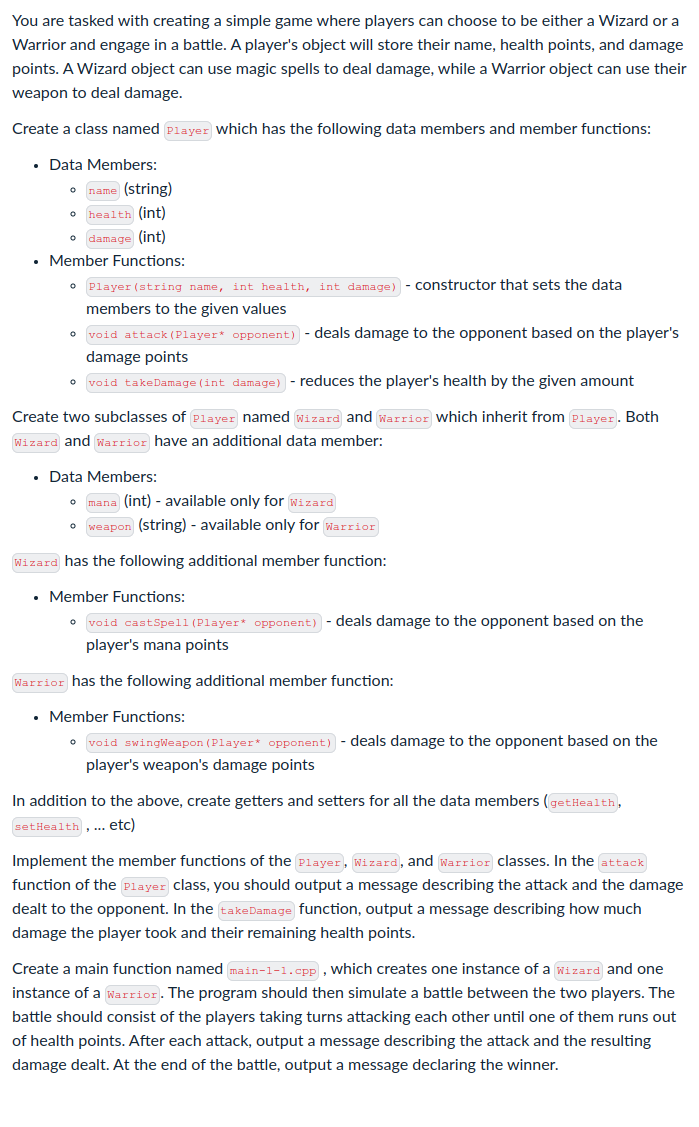
Transcribed Image Text:You are tasked with creating a simple game where players can choose to be either a Wizard or a
Warrior and engage in a battle. A player's object will store their name, health points, and damage
points. A Wizard object can use magic spells to deal damage, while a Warrior object can use their
weapon to deal damage.
Create a class named Player which has the following data members and member functions:
• Data Members:
o name (string)
o health (int)
• damage (int)
• Member Functions:
• Player (string name, int health, int damage) - constructor that sets the data
members to the given values
• void attack (Player* opponent) - deals damage to the opponent based on the player's
damage points
• void takeDamage (int damage) - reduces the player's health by the given amount
Create two subclasses of Player named Wizard and Warrior which inherit from Player. Both
Wizard and Warrior have an additional data member:
• Data Members:
omana (int) - available only for wizard
• weapon (string) - available only for Warrior
Wizard has the following additional member function:
• Member Functions:
void castSpell (Player* opponent) - deals damage to the opponent based on the
player's mana points
Warrior has the following additional member function:
• Member Functions:
• void swingWeapon (Player* opponent) - deals damage to the opponent based on the
player's weapon's damage points
In addition to the above, create getters and setters for all the data members (getHealth,
setHealth, ... etc)
Implement the member functions of the Player, Wizard, and Warrior classes. In the attack
function of the player class, you should output a message describing the attack and the damage
dealt to the opponent. In the takeDamage function, output a message describing how much
damage the player took and their remaining health points.
Create a main function named main-1-1.cpp, which creates one instance of a wizard and one
instance of a Warrior. The program should then simulate a battle between the two players. The
battle should consist of the players taking turns attacking each other until one of them runs out
of health points. After each attack, output a message describing the attack and the resulting
damage dealt. At the end of the battle, output a message declaring the winner.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 5 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
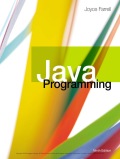
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
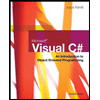
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
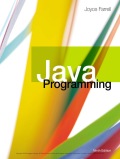
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
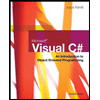
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage