Four in a row is a game in which players take turns adding tokens to the columns on the game board. Tokens fall to the lowest position in the chosen column that does not already have a token in it. Once one of the players has placed four of their tokens in a straight line (either vertically, horizontally, or diagonally), they win the game If the board is full and no player has won, then the game ends in a draw. TASK Using the following class descriptions, create a UML diagram and a version of Four in a row game
Control structures
Control structures are block of statements that analyze the value of variables and determine the flow of execution based on those values. When a program is running, the CPU executes the code line by line. After sometime, the program reaches the point where it has to make a decision on whether it has to go to another part of the code or repeat execution of certain part of the code. These results affect the flow of the program's code and these are called control structures.
Switch Statement
The switch statement is a key feature that is used by the programmers a lot in the world of programming and coding, as well as in information technology in general. The switch statement is a selection control mechanism that allows the variable value to change the order of the individual statements in the software execution via search.
Four in a row is a game in which players take turns adding tokens to the columns on the game board.
Tokens fall to the lowest position in the chosen column that does not already have a token in it. Once one of the players has placed four of their tokens in a straight line (either vertically, horizontally, or diagonally), they win the game
If the board is full and no player has won, then the game ends in a draw.
TASK
Using the following class descriptions, create a UML diagram and a version of Four in a row game
-
The game must allow for a minimum of two and maximum of four players
-
The game must allow each player to enter their name(duplicate names should not be accepted)
-
The game should give the players the ability to choose how many rows (between four and ten), and how many columns (between four and ten) the game board should have.
-
The code uses several classes, including "Player", "Board","Game" and exceptions for handling errors such as invalid moves and full columns.
Overview
-
Create the Player class, which will handle the players in the game.
-
Create the constructor, which will take in the player's name and player number as arguments and set them to instance variables.
-
Create the getName and getPlayerNumber methods, which will return the player's name and player number, respectively.
-
Create the makeMove method, which will prompt the user for the column where they want to drop their token and return that value.
-
Create a UML class diagram to visualize the relationships between classes and their methods and attributes. You can use a UML tool like Lucidchart, Visual Paradigm, or draw.io to create a class diagram.
![# Board Class
Attribute/Method
board
boardSetUp()
printBoard()
columnFull()
boardFull()
Checks if a given column is full by checking if the top spot of the column is empty.
Checks if the entire board is full by checking if all columns are full.
Adds a token to the board for a given column and player. It checks if the column is full and if it is, it throws
an exception. It also checks if the column number provided is valid and if it is not, it throws an exception.
checklfPlayerls The Winner() Checks if the current player has won the game by getting four of their tokens in a row either horizontally,
vertically, or diagonally by calling other methods.
addToken()
checkVertical()
Description
Keeps track of which player's token (if any) is stored at each location on the game board
Prompts the user for the number of rows and columns for the board and initializes the board with empty
spaces represented by "-"
prints the current state of the board to the console.
checkHorizontal()
Checks if a player has won vertically by iterating through the board array and comparing four consecutive
rows in a column.
Checks if a player has won horizontally by iterating through the board array and comparing four consecutive
columns in a row.
Player Class
Attribute/Method Description
name
playerNumber
Player()
getName()
getNumber()
makeMove()
toString()
Specifies this player's name
Specifies the number of the player's token
Constructor takes a name and playerNumber as parameters and assigns them to the corresponding instance
variables.
Accessor for name
Accessor for playerNumber
Prompts the player for which column they want to place their token in, and returns the column number.
Returns a string representation of the player in the format "Player [player number] is [name]".](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa3cab9c1-3149-4232-8d34-9f213defe2a3%2F17a73a56-0ca4-463c-91c7-38efd140fd5d%2Fnmyg9pr_processed.png&w=3840&q=75)
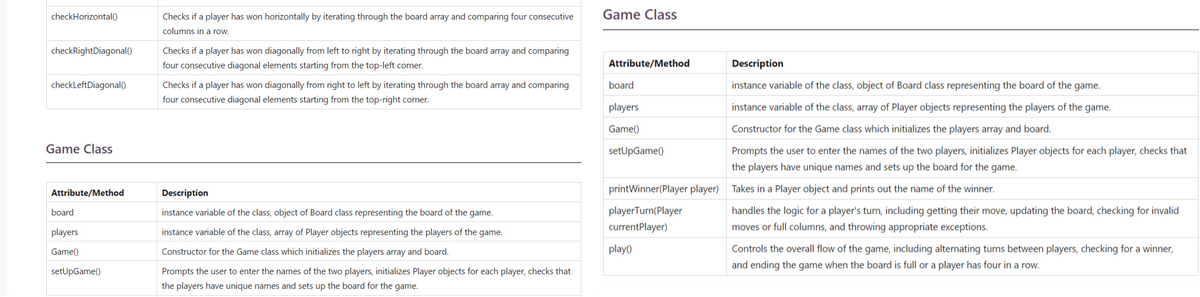

Step by step
Solved in 4 steps

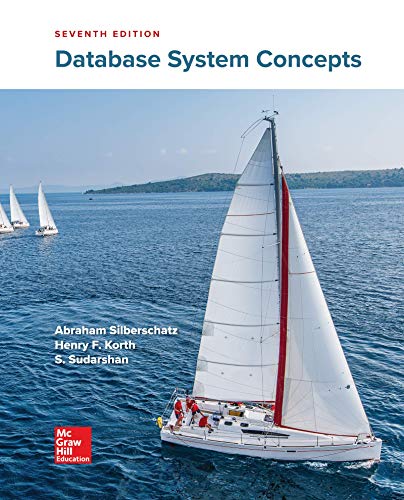
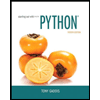
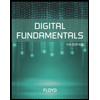
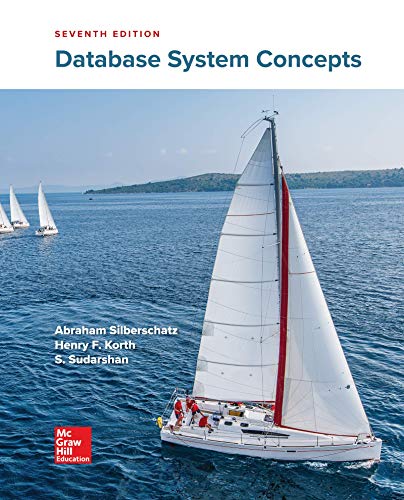
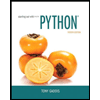
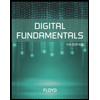
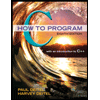
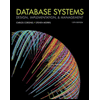
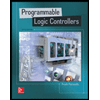