You are to work to develop a linked-list processing function IsolateTargetSoloAsTail that is to process a linked list as follows. ● If target cannot be found on the given list, a new node containing target is created and added to the end (made the new tail node) of the list. ► This includes the case where the given list is empty, in which case the new tail node added is also new head node. (This is so because the only node in a 1-node list is both the head and tail node of the list.) ● If target appears only once on the given list, the target-matching node is moved to the end (made the new tail node) of the list. ► In case the target-matching node is already the tail node (of the given li
You are to work to develop a linked-list processing function IsolateTargetSoloAsTail that is to process a linked list as follows. |
● | If target cannot be found on the given list, a new node containing target is created and added to the end (made the new tail node) of the list. |
► | This includes the case where the given list is empty, in which case the new tail node added is also new head node. (This is so because the only node in a 1-node list is both the head and tail node of the list.) |
● | If target appears only once on the given list, the target-matching node is moved to the end (made the new tail node) of the list. |
► | In case the target-matching node is already the tail node (of the given list), then nothing needs to be done. |
● | If target appears multiple times on the given list, the first target-matching node is moved to the end (made the new tail node) of the list, and all other target-matching nodes are to be deleted from the list. |
► | Note that although the target-matching node to be moved does not have to be the first (i.e., it can also be any of the other target-matching nodes) for the function to work as intended, you are to make it so for the purpose (and simplicity/uniformity) of this exercise. |
● | The order in which non-target-matching nodes originally appear in the given list must be preserved. |
|
The last 2 examples have nodes shown colored black to indicate which of the target-matching nodes in the given list has been moved to become the tail node in the processed list. |
● | Fill in the prototype for IsolateTargetSoloAsTail in the supplied header file (llcpInt.h). |
● | Fill in the definition for IsolateTargetSoloAsTail in the supplied implementation file (llcpImp.cpp). |
llcplnt.h
#ifndef ASSIGN05P1_H
#define ASSIGN05P1_H
#include <iostream>
struct Node
{
int data;
Node *link;
};
int FindListLength(Node* headPtr);
bool IsSortedUp(Node* headPtr);
void InsertAsHead(Node*& headPtr, int value);
void InsertAsTail(Node*& headPtr, int value);
void InsertSortedUp(Node*& headPtr, int value);
bool DelFirstTargetNode(Node*& headPtr, int target);
bool DelNodeBefore1stMatch(Node*& headPtr, int target);
void ShowAll(std::ostream& outs, Node* headPtr);
void FindMinMax(Node* headPtr, int& minValue, int& maxValue);
double FindAverage(Node* headPtr);
void ListClear(Node*& headPtr, int noMsg = 0);
// prototype of IsolateTargetSoloAsTail of Assignment 5 Part 1
#endif
llcpImp.cpp

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

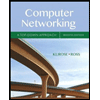
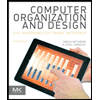
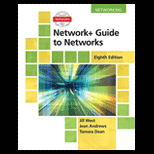
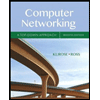
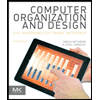
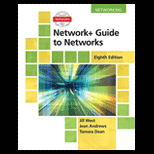
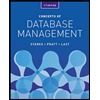
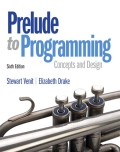
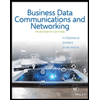