You are working for a university to maintain a list of grades and some related statistics for a student. Class and Data members: Create a class called Student that stores a student’s grades (integers) in a vector (do not use an array). The class should have data members that store a student’s name and the course for which the grades are earned. Constructor(s): The class should have a 2-argument constructor that receives the student’s name and course as parameters and sets the appropriate data members to these values. Member Functions: The class should have functions as follows: Member functions to set and get the student’s name and course variables. A member function that adds a single grade to the vector. Only positive grades are allowed. Call this function AddGrade. A member function to sort the vector in ascending order. A member function to compute the average (x̄) of the grades in the vector. The formula for calculating an average is x̄ = ∑xi / n where xi is the value of each grade and n is the total number of grades in the vector. When using a vector, you can manipulate it just like an array. 5. A member function to determine the lowest grade in the vector and a separate member function to determine the highest grade in the vector. [Note that to receive credit for these functions, they must contain an algorithm to search through the vector to determine the minimum or maximum value. You cannot simply sort the vector and return the first or last data member or use the *min_element or *max_element functions.] 6. A member function (e.g. getNumGrades) to return the number of grades that are stored in the vector. Remember that a vector knows its own size. Therefore, you don’t need to keep track of the number of items stored in a vector in a separate data member in the class. You also don’t want to make your vector public – it should be private. Therefore, you can create a public getNumGrades function that will simply return the size of the vector. 7. A member function to display the student’s name, course, and vector of sorted grades. Write a program (client) that uses the class by creating a Student object and prompting the user for a file name. Appropriate error checking is required to ensure that the file exists and can be opened successfully. Also, the name of the file may contain a space; therefore, be sure to use getline instead of cin when you prompt the user to enter the name of the file. The client should read in the file contents and store them in the object. The file will be formatted such that the first line contains the student’s name, the second line contains the course name, and each successive line contains a grade. A typical input file might contain: John Smith CSIS 112 90 85 97 91 87 86 88 82 83 Note that the file may contain any number of grades for a given course. Therefore, you need to read in and store each grade until you reach the end of the file. The client (i.e. main()) should read in the contents of the file. After each grade is read in, it should call the addGrade member function in the Student class to add the new grade (i.e. one grade at a time) to the vector. [Do not create a vector in main and pass the entire vector in to the addGrade function in the Student class. Pass in only one grade at a time and allow the addGrade function to add it to the vector of grades in the class.] Main() should then produce a report that displays the student’s name and course, the total number of grades in the file, the lowest grade, the highest grade, the average of all the grades, and finally, a listing of all of the grades that were read in. The listing of all of the grades must be displayed in sorted order (ascending – from lowest to highest). All output should be labeled appropriately, and validity checking should be done on input of the filename and also the grades that are read in. If a non-numeric or negative value is encountered in the file when reading in the grades, the program should output an error message indicating that a non-numeric or negative value was found in the file, and the entire program should then terminate. If a non-numeric or negative value is found, consider the entire file to be corrupted and don’t try to produce any calculations nor display the contents of the vector – just end the program with an appropriate error message. [Make sure the error message is displayed long enough for the user to read it before ending the program. – you may use the system(“pause”) command for this. Note that there are several security and performance issues with using system commands, and you’ll learn more about these later, but for now, feel free to use the system(“pause”) command. ]
You are working for a university to maintain a list of grades and some related statistics for a student.
Class and Data members:
Create a class called Student that stores a student’s grades (integers) in a
Constructor(s):
The class should have a 2-argument constructor that receives the student’s name and course as parameters and sets the appropriate data members to these values.
Member Functions:
The class should have functions as follows:
- Member functions to set and get the student’s name and course variables.
- A member function that adds a single grade to the vector. Only positive grades are allowed. Call this function AddGrade.
- A member function to sort the vector in ascending order.
- A member function to compute the average (x̄) of the grades in the vector. The formula for calculating an average is
x̄ = ∑xi / n
where xi is the value of each grade and n is the total number of grades in the vector. When using a vector, you can manipulate it just like an array.
5. A member function to determine the lowest grade in the vector and a separate member function to determine the highest grade in the vector. [Note that to receive credit for these functions, they must contain an
6. A member function (e.g. getNumGrades) to return the number of grades that are stored in the vector. Remember that a vector knows its own size. Therefore, you don’t need to keep track of the number of items stored in a vector in a separate data member in the class. You also don’t want to make your vector public – it should be private. Therefore, you can create a public getNumGrades function that will simply return the size of the vector.
7. A member function to display the student’s name, course, and vector of sorted grades.
Write a
The client should read in the file contents and store them in the object. The file will be formatted such that the first line contains the student’s name, the second line contains the course name, and each successive line contains a grade. A typical input file might contain:
John Smith
CSIS 112
90
85
97
91
87
86
88
82
83
Note that the file may contain any number of grades for a given course. Therefore, you need to read in and store each grade until you reach the end of the file.
The client (i.e. main()) should read in the contents of the file. After each grade is read in, it should call the addGrade member function in the Student class to add the new grade (i.e. one grade at a time) to the vector. [Do not create a vector in main and pass the entire vector in to the addGrade function in the Student class. Pass in only one grade at a time and allow the addGrade function to add it to the vector of grades in the class.]
Main() should then produce a report that displays the student’s name and course, the total number of grades in the file, the lowest grade, the highest grade, the average of all the grades, and finally, a listing of all of the grades that were read in. The listing of all of the grades must be displayed in sorted order (ascending – from lowest to highest).
All output should be labeled appropriately, and validity checking should be done on input of the filename and also the grades that are read in.
If a non-numeric or negative value is encountered in the file when reading in the grades, the program should output an error message indicating that a non-numeric or negative value was found in the file, and the entire program should then terminate. If a non-numeric or negative value is found, consider the entire file to be corrupted and don’t try to produce any calculations nor display the contents of the vector – just end the program with an appropriate error message. [Make sure the error message is displayed long enough for the user to read it before ending the program. – you may use the system(“pause”) command for this. Note that there are several security and performance issues with using system commands, and you’ll learn more about these later, but for now, feel free to use the system(“pause”) command. ]
The executing program should look something like this:
visual studio c++ code
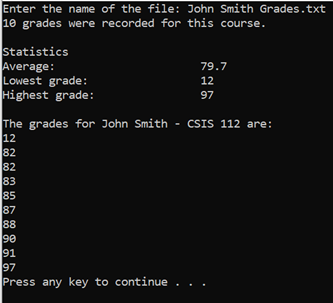


Trending now
This is a popular solution!
Step by step
Solved in 9 steps with 10 images

i keep getting an error for the getline(infile,studentName);
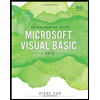
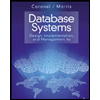
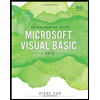
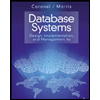
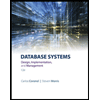
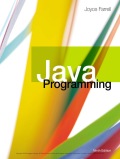