You will be writing a program for a local bank that allows them to create objects repres account. Create the following two classes: • Transaction: a class representing a customer's single transaction at the bank: • Member Variables: · amount: An int representing how much the transaction was for. • isWithdrawal: A bool: true if the transaction was a withdrawal and false if it • Member Functions
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Please do not copy and paste the answer that Chegg has for this question. I have asked this question already and the expert copied and pasted the answer from Chegg. Please actually solve this problem, or I'll just have to cancel my Bartleby subscription.
C++ Question
Hello, Please create the correct code based on the attached requirement picture. Please make sure the code is functional. Please do not use any Advanced C++ syntax. Please make sure to submit the codes for Transaction.cpp, Transaction.h, Source.cpp, Account.cpp, and Account.h Thank you.
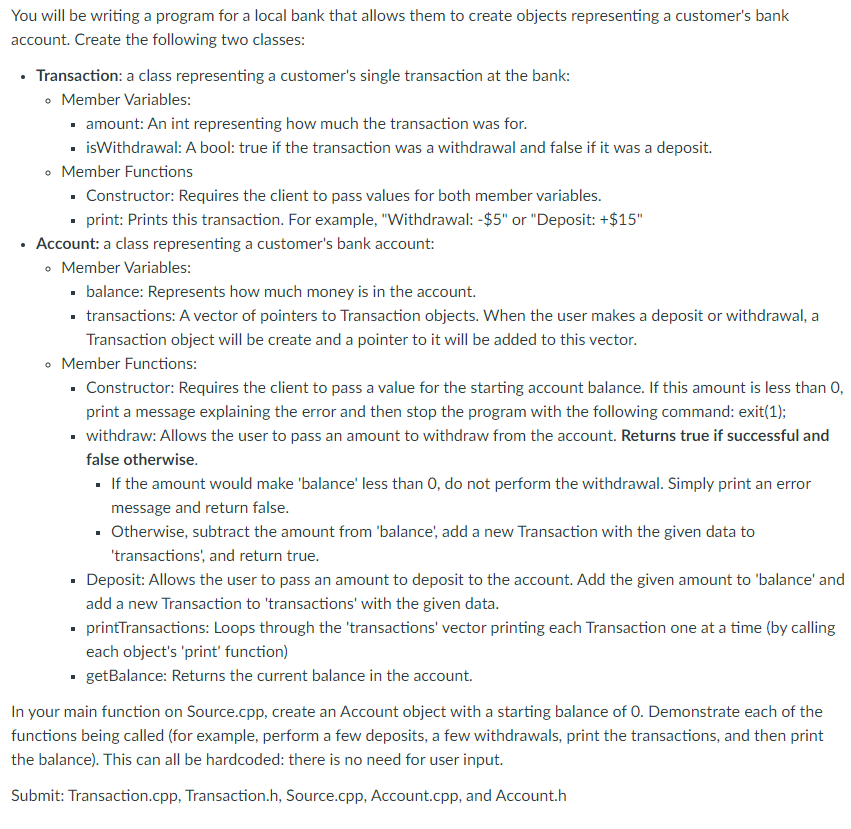

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

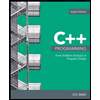
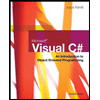
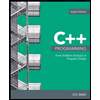
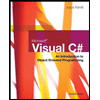