You will complete a class called Socks. It simulates loading a series of socks into a sock drawer.. The class will have the following methods: • newsocks( ) : this method will receive a series of socks. Your code will ask the user haw many pairs of socks are being added to drawer (because, after all, socks should ALWAYS come in paris). Then use a loop to obtain the sock color. Users can enter blue, brown, black, or white for sock color. For any other color, they will receive a message that the color is not allowed and to enter a proper color. o How many pairs of socks? (Answer: 3) o For sock #1, what color? (Answer: white) o For sock #2, what color? (Answer: blue) o For sock #3, what color? (Answer: green) o That is not a valid color. Try again. For sock #3, what color? (Answer: black This method will update the attribute to maintain the current and accurate count of socks. • list( ) : this method will print the number of sock pairs for each color, one color per line. For instance, the result might look like this: o There are 12 pairs of blue socks o There are 5 pairs of brown socks o There are 6 pairs of black socks o There are 11 pairs of white socks
You will complete a class called Socks. It simulates loading a series of socks into a sock drawer..
The class will have the following methods:
• newsocks( ) : this method will receive a series of socks. Your code will ask the user
haw many pairs of socks are being added to drawer (because, after all, socks should
ALWAYS come in paris). Then use a loop to obtain the sock color. Users can enter
blue, brown, black, or white for sock color. For any other color, they will receive a
message that the color is not allowed and to enter a proper color.
o How many pairs of socks? (Answer: 3)
o For sock #1, what color? (Answer: white)
o For sock #2, what color? (Answer: blue)
o For sock #3, what color? (Answer: green)
o That is not a valid color. Try again. For sock #3, what color? (Answer: black
This method will update the attribute to maintain the current and accurate count of
socks.
• list( ) : this method will print the number of sock pairs for each color, one color per line.
For instance, the result might look like this:
o There are 12 pairs of blue socks
o There are 5 pairs of brown socks
o There are 6 pairs of black socks
o There are 11 pairs of white socks
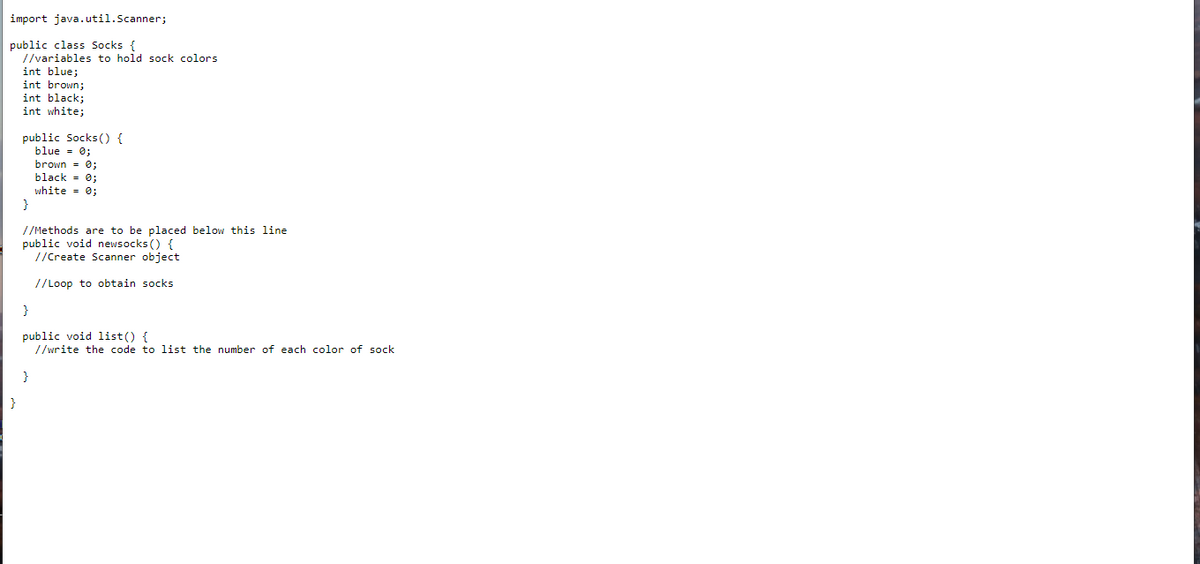
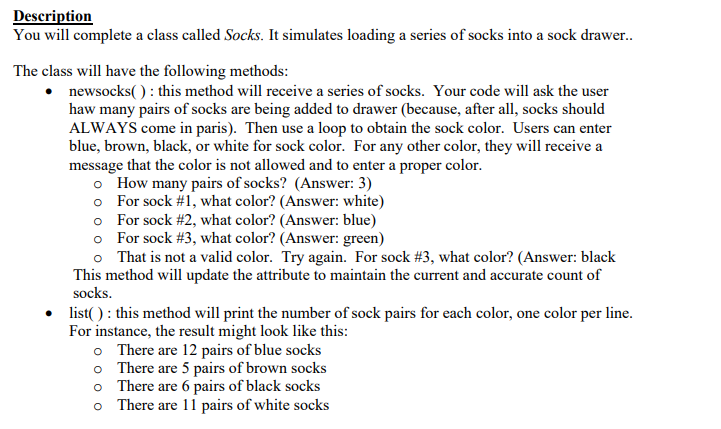

In this question we will write a java code for the socks class where we can add new socks and list them.
Let's code and hope this helps, if you find any query on solution, utilize threaded question feature.
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

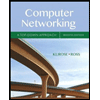
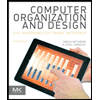
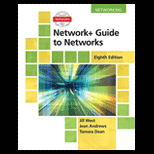
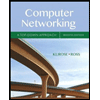
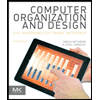
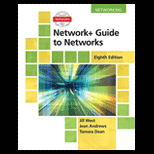
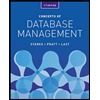
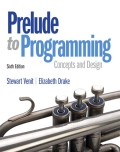
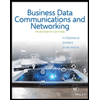