Your activity: write a same java program that will accept 20 integer in a list and returns the index of the first occurrence of the smallest element in the array. Also, write a program to test your method. Write a Java method, smallestIndex, that takes as its parameters an int array and its size, and returns the index of the (first occurrence of the) smallest element in the array. public class Array_labExercise2 { public static void main(String[] args) { int[] list = {56, 34, 67, 54, 23, 87, 66, 92, 15, 32, 55, 15, 88, 22, 30}; System.out.print("List elements: "); printArray(list); System.out.println(); System.out.println("Index of the (first occurenc of the) " + "smallest element in list is: " + smallestIndex(list)); System.out.println("Smallest element in list is: " + list[smallestIndex(list)]); } public static void printArray(int[] list) { int counter; for (counter = 0; counter < list.length; counter++) System.out.print(list[counter] + " "); } public static int smallestIndex(int[] list) { int counter; int minIndex = 0; //Assume first element is the largest for (counter = 1; counter < list.length; counter++) if (list[minIndex] > list[counter]) minIndex = counter; return minIndex; } } Output:
Your activity: write a same java program that will accept 20 integer in a list and returns the index of the first occurrence of the smallest element in the array. Also, write a program to test your method.
Write a Java method, smallestIndex, that takes as its parameters an int array and its size, and returns the index of the (first occurrence of the) smallest element in the array.
public class Array_labExercise2
{
public static void main(String[] args)
{
int[] list = {56, 34, 67, 54, 23, 87, 66, 92, 15, 32, 55, 15, 88, 22, 30};
System.out.print("List elements: ");
printArray(list);
System.out.println();
System.out.println("Index of the (first occurenc of the) " + "smallest element in list is: " + smallestIndex(list));
System.out.println("Smallest element in list is: " + list[smallestIndex(list)]);
}
public static void printArray(int[] list)
{
int counter;
for (counter = 0; counter < list.length; counter++)
System.out.print(list[counter] + " ");
}
public static int smallestIndex(int[] list)
{
int counter;
int minIndex = 0; //Assume first element is the largest
for (counter = 1; counter < list.length; counter++)
if (list[minIndex] > list[counter])
minIndex = counter;
return minIndex;
}
}
Output:
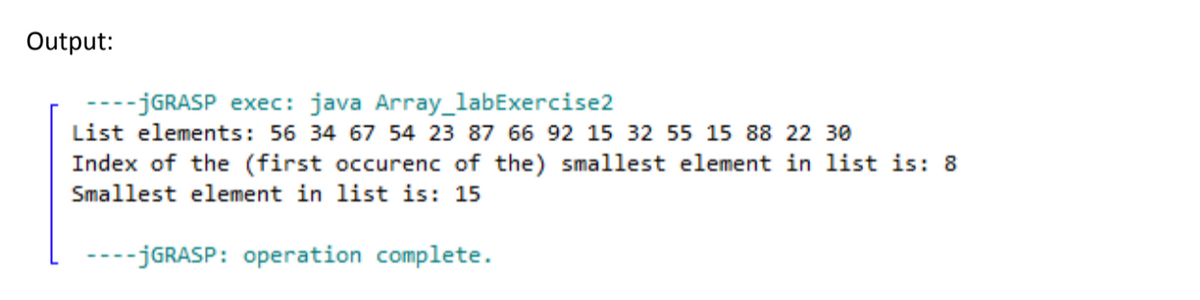

Step by step
Solved in 3 steps with 1 images

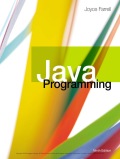
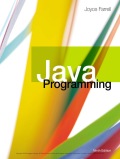