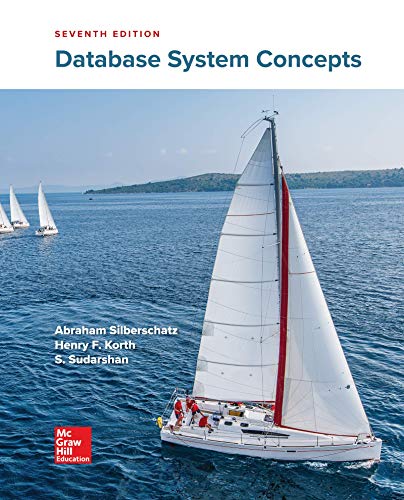
Concept explainers
import java.util.ArrayList;
import java.util.Arrays;
public class PS07A
{
/**
* Write the method named mesh.
*
* Start with two ArrayLists of String, A and B, each with
* its elements in alphabetical order and without any duplicates.
* Return a new list containing the first N elements from the two
* lists. The result list should be in alphabetical order and without
* duplicates. A and B will both have a size which is N or more.
* Your solution should make a single pass over A and B, taking
* advantage of the fact that they are in alphabetical order,
* copying elements directly to the new list.
*
* Remember, to see if one String is "greater than" or "less than"
* another, you need to use the compareTo() method, not the < or >
* operators.
*
* Examples:
* mesh(["a","c","z"], ["b","f","z"], 3) returns ["a","b","c"]
* mesh(["a","c","z"], ["c","f","z"], 3) returns ["a","c","f"]
* mesh(["f","g","z"], ["c","f","g"], 3) returns ["c","f","g"]
*
* @param a an ArrayList of String in alphabetical order.
* @param b an ArrayList of String in alphabetical order.
* @param n the size of the resulting list to return.
* @return a list of n elements in alphabetical order.
*/
// TODO - Write the mesh method here.
/**
* Write the method named examScore().
*
* The "key" list contains the correct answers
* to an exam, like ["a", "a", "b", "b"]. The "answers"
* list contains a student's answers, with "?" representing
* a question left blank. The two lists are not empty and
* are the same length. Return the score for this list
* of answers, giving +4 for each correct answer, -1 for
* each incorrect answer, and +0 for each blank answer.
*
* Examples:
* examScore(["a", "a", "b", "b"], ["a", "c", "b", "c"]) returns 6
* examScore(["a", "a", "b", "b"], ["a", "a", "b", "c"]) returns 11
* examScore(["a", "a", "b", "b"], ["a", "a", "b", "b"]) returns 16
*
* @param key a list of correct answers to an exam.
* @param answers the student's answers to the exam.
* @return the score as described above.
*/
// TODO - Write the examScore method here.
/**
* Write the method filterBySize().
*
* Given an ArrayList of String, return a new list where only
* strings of the given length are retained.
*
* Examples:
* filterBySize(["a", "bb", "b", "ccc"], 1) returns ["a", "b"]
* filterBySize(["a", "bb", "b", "ccc"], 3) returns ["ccc"]
* filterBySize(["a", "bb", "b", "ccc"], 4) returns []
*
* @param list the list of Strings to process.
* @param n the size of words to retain.
* @return a new list of words with the indicated size retained.
*/
// TODO - Write the filterBySize method here.
/**
* Write the method named nOdds().
*
* Given an ArrayList of positive Integers, return a new list
* of length "count" containing the first odd numbers from
* the original list. The original will contain at least
* "count" odd numbers.
*
* Examples:
* nOdds([3, 2, 4, 5, 8], 2) returns [3, 5]
* nOdds([3, 2, 4, 5, 8, 9], 3) returns [3, 5, 9]
* nOdds([3, 1, 2, 4, 5, 8], 3) returns [3, 1, 5]
*
* @param list the ArrayList of Integers to search.
* @param count the number of odd numbers to copy.
* @return the first "count" odd numbers from the list.
*/
// TODO - Write the nOdds method here.
// A utility method for testing
@SuppressWarnings("unchecked")
public static <T> ArrayList<T> al(T...o)
{
ArrayList<T> result = new ArrayList<T>();
for (T s : o) result.add(s);
return result;
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Java Programming! Please, write the method only, not an entire program. Thanks Write a method that is passed in an ArrayList of Strings and returns true of the list is sorted in non-descending order, false if not.arrow_forwardComplete the below function (using recursion)arrow_forwardHas to be done in Java. Import ArrayList.arrow_forward
- USING THE FOLLOWING METHOD SWAP CODE: import java.util.*; class ListIndexOutOfBoundsException extends IndexOutOfBoundsException { public ListIndexOutOfBoundsException(String s) { super(s); } } class ListUtil { public static int maxValue(List<Integer> aList) { if(aList == null || aList.size() == 0) { throw new IllegalArgumentException("List cannot be null or empty"); } int max = aList.get(0); for(int i = 1; i < aList.size(); i++) { if(aList.get(i) > max) { max = aList.get(i); } } return max; } public static void swap(List<Integer> aList, int i, int j) throws ListIndexOutOfBoundsException { if(aList == null) { throw new IllegalArgumentException("List cannot be null"); } if(i < 0 || i >= aList.size() || j < 0 || j >= aList.size()) { throw new ListIndexOutOfBoundsException("Index out…arrow_forwardstarter code //Provided imports, feel free to use these if neededimport java.util.Collections;import java.util.ArrayList; /** * TODO: add class header */public class Sorts { /** * this helper finds the appropriate number of buckets you should allocate * based on the range of the values in the input list * @param list the input list to bucket sort * @return number of buckets */ private static int assignNumBuckets(ArrayList<Integer> list) { Integer max = Collections.max(list); Integer min = Collections.min(list); return (int) Math.sqrt(max - min) + 1; } /** * this helper finds the appropriate bucket index that a data should be * placed in * @param data a particular data from the input list if you are using * loop iteration * @param numBuckets number of buckets * @param listMin the smallest element of the input list * @return the index of the bucket for which the particular data should…arrow_forwardJava language Write a method to insert an array of elements at index in a single linked list and then display this list. The method receives this array by parametersarrow_forward
- Lab 14.1 Beginning to build an Arrazlizt recursively In this sequence of problems we practice recursion by abandoning our reliance on iteration. We resolve to solve a sequence of problems without using while or for loops. Instead we will think recursively and look at the world through a different lens. Recursion is all about solving a large problem by using the solution to a similar smaller problem. The brilliant thing about recursion is that you can assume you already know how to solve the smaller problem. The goal is to demonstrate how the smaller solution relates to the larger problem at hand. For example, suppose you want to print all binary strings of length 3 and you already know how to print all binary strings of length 2. Here they are: 00 01 10 11 How can we solve the larger problem with a list of strings of length 2? Add a "0" or "1", right? So here is the solution to the larger problem: 00 + 0 = 000 01 + 0 = 010 10 + 0 = 100 11 +0 = 110 and 00 + 1 = 001 01 + 1 = 011 10 + 1 =…arrow_forwardHas to be done in Java, import ArrayListarrow_forwardPythong help!arrow_forward
- Complete the combinations function to generate all combinations of the characters in the string s with length k recursivelyarrow_forwardPYTHON LAB: Inserting an integer in descending order (doubly-linked list) Given main.py and an IntNode class, complete the IntList class (a linked list of IntNodes) by writing the insert_in_descending_order() method to insert new IntNodes into the IntList in descending order. Ex. If the input is: 3 4 2 5 1 6 7 9 8 the output is: 9 8 7 6 5 4 3 2 1 _____________________________________________________________________________________ Main.py: from IntNode import IntNode from IntList import IntList if __name__ == "__main__": int_list = IntList() input_line = input() input_strings = input_line.split(' ') for num_string in input_strings: # Convert from string to integer num = int(num_string) # Insert into linked list in descending order new_node = IntNode(num) int_list.insert_in_descending_order(new_node) int_list.print_int_list() IntNode.py class IntNode: def __init__(self, initial_data, next = None,…arrow_forwardjava In this assignment you will swap a position in an array list with another. swap() gets 3 arguments, an Arraylist, a position, and another position to swap with. Example swap(["one","two","three"],0,2) returns:["three","two","one"] public static ArrayList<String> swap(ArrayList<String> list,int pos1,int pos2) public static void main(String[] args) { Scanner in = new Scanner(System.in); int size = in.nextInt(); int pos1 = in.nextInt(); int pos2 = in.nextInt(); ArrayList<String> list = new ArrayList<>(); for(int i=0; i < size; i++) { list.add(in.next()); } System.out.println(swap(list, pos1, pos2)); } }arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
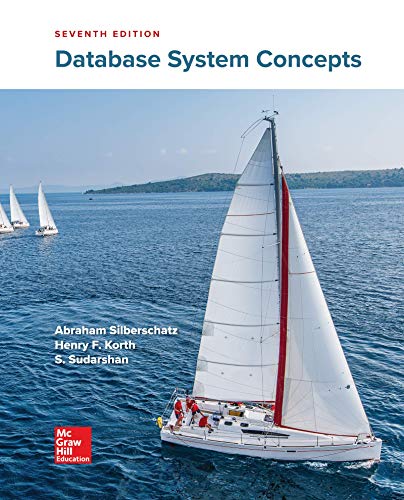
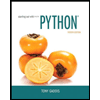
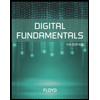
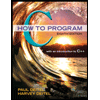
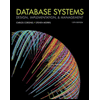
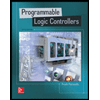