Your task for this assignment is to implement a stack data structure in C++. This may be accomplished by utilizing the C++ standard template library (STL) or by utilizing a user-defined class. 1. Implement a transaction-based stack data structure using C++. The program will be interactive. Data transactions will be entered at the command line and results will be displayed on the console. 2. Each input transaction will contain an arithmetic expression in post-fix format. Assume that each operand and operation (+, -, *, /, %, ^) is a single character in each arithmetic expression without spacing. You may assume that each arithmetic expression is correctly formatted. However, your program should not attempt the following: a. Divide by zero with / or %. Instead, your program should display an error message and then begin evaluating a new input transaction. b. Perform % with non-integer operands . Instead, your program should display an error message and then begin evaluating a new input transaction. c. Perform ^ with a negative base . Instead, your program should display an error message and then begin evaluating a new input transaction. Note that the intermediate or final results of an expression may be negative or non- integer.
Your task for this assignment is to implement a stack data structure in C++. This may be accomplished by utilizing the C++ standard template library (STL) or by utilizing a user-defined class. 1. Implement a transaction-based stack data structure using C++. The program will be interactive. Data transactions will be entered at the command line and results will be displayed on the console. 2. Each input transaction will contain an arithmetic expression in post-fix format. Assume that each operand and operation (+, -, *, /, %, ^) is a single character in each arithmetic expression without spacing. You may assume that each arithmetic expression is correctly formatted. However, your program should not attempt the following: a. Divide by zero with / or %. Instead, your program should display an error message and then begin evaluating a new input transaction. b. Perform % with non-integer operands . Instead, your program should display an error message and then begin evaluating a new input transaction. c. Perform ^ with a negative base . Instead, your program should display an error message and then begin evaluating a new input transaction. Note that the intermediate or final results of an expression may be negative or non- integer.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question

Transcribed Image Text:Home
CSC331 Program 3 specifications
Your task for this assignment is to implement a stack data structure in C++. This may be
accomplished by utilizing the C++ standard template library (STL) or by utilizing a user-defined
class.
1.
Implement a transaction-based stack data structure using C++. The program will be
interactive. Data transactions will be entered at the command line and results will be
displayed on the console.
Each input transaction will contain an arithmetic expression in post-fix format. Assume
that each operand and operation (+, -, *, /, %, ^) is a single character in each arithmetic
expression without spacing. You may assume that each arithmetic expression is correctly
formatted. However, your program should not attempt the following:
a. Divide by zero with / or % . Instead, your program should display an error message
and then begin evaluating a new input transaction.
b. Perform % with non-integer operands . Instead, your program should display an
error message and then begin evaluating a new input transaction.
c. Perform ^ with a negative base . Instead, your program should display an error
message and then begin evaluating a new input transaction.
Note that the intermediate or final results of an expression may be negative or non-
integer.
2.
Sample input transactions and results:
а. 23+
(result = 5)

Transcribed Image Text:Home
CSC331 Program 3 specifications
and then begin evaluating a new input transaction.
b. Perform % with non-integer operands . Instead, your program should display an
error message and then begin evaluating a new input transaction.
c. Perform ^ with a negative base . Instead, your program should display an error
message and then begin evaluating a new input transaction.
Note that the intermediate or final results of an expression may be negative or non-
integer.
Sample input transactions and results:
а. 23+
b. 34*5/
(result = 5)
(result = 2.4)
(result = "error: division by zero")
c. 832*6-/
d. 48*62*42^3+-% (result = 4)
e. 92/73/5%4/+
(result = "error: non-integer operand for %")
3.
An input transaction containing "end-of-file" indicates there are no more transactions to
be processed. Implement a stack to evaluate each expression and display the result. Use
the C++ built-in class or a user-defined class to implement stack functions.
The program will be run at the command prompt by navigating to the directory
containing the executable version of the program after the program is compiled. The
program should display a prompt requesting input, such as "Please enter an expression in
post-fix notation:".
4.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 12 images

Recommended textbooks for you
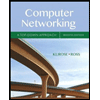
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
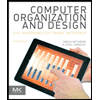
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
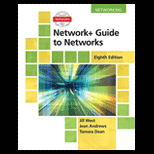
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
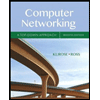
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
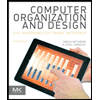
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
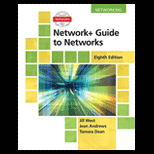
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
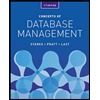
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
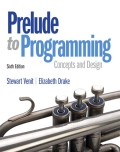
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
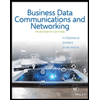
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY