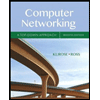
Java Programming
Please do not change anything in Student class or Course class
class John_Smith extends Student{
public John_Smith() {
setFirstName("John");
setLastName("Smith");
setEmail("jsmith@jaguar.tamu.edu");
setGender("Male");
setPhoneNumber("(200)00-0000");
setJNumber("J0101459");
}
class MyCourse extends Course {
public MyCourse {
class Course {
private String courseNumber;
private String courseName;
private int creditHrs;
public Course (String number, String name, int creditHrs){
this.courseNumber = number;
this.courseName = name;
this.creditHrs = creditHrs;
}
public String getNumber() {
return courseNumber;
}
public String getName() {
return courseName;
}
public int getCreditHrs() {
return creditHrs;
}
public void setCourseNumber(String courseNumber) {
this.courseNumber = courseNumber;
}
public void setCourseName(String courseName) {
this.courseName = courseName;
}
public void setCreditHrs(int creditHrs) {
this.creditHrs = creditHrs;
}
}
class Student {
private String firstName;
private String lastName;
private String gender;
private String phoneNumber;
private String email;
private String jNumber;
protected ArrayList<MyCourse> courseList;
public String getFullName() {
return firstName + " " + lastName;
}
public void setFirstName(String fName) {
firstName = fName;
}
public void setLastName(String lName) {
lastName = lName;
}
public String getGender() {
return gender;
}
public void setGender(String gen) {
gender = gen;
}
public String getPhoneNumber() {
return phoneNumber;
}
public void setPhoneNumber(String pNumber) {
phoneNumber = pNumber;
}
public String getEmail() {
return email;
}
public void setEmail(String e_mail) {
email = e_mail;
}
public String getJNumber() {
return jNumber;
}
public void setJNumber(String jNum) {
jNumber = jNum;
}
/* OVERRIDE!
Print student's basic information following the format in the assignment's description*/
public void printBasicInfo() {
}
/* OVERRIDE!
Print all courses following the format in the assignment's description */
public void printCourseList() {
}
/* OVERRIDE!
Enroll a new course by adding it into the courseList
Do NOT add a course if it is already in the courseList */
public void addCourse(MyCourse course) {
}
/* OVERRIDE!
If the course exists, remove it from the courseList
throw CourseNotFoundException if it doesn't exist*/
public void dropCourse(MyCourse course) {
}
/* OVERRIDE!
Return true if the course is currently enrolled, otherwise, false */
public boolean isEnrolled(MyCourse course) {
return false;
}
/* OVERRIDE!
Return total credit hours for all enrolled courses */
public int getTotalCredits() {
return 0;
}
}
![[1] Description: You're asked to create a basic Student's Enrollment System with the provided
Student and Course classes. Please download the source code files from Blackboard. Create two
new classes: (i) FirstName_LastName (replace by your name) that extends the Student class and
(i) MyCourse that extends the Course class.
12] Requirements:
a. You're NOT allowed to change or add ANYTHING in the provided Student and Course
classes.
b. Provide a no-arg constructor for your FirstName_LastName class. The no-arg
constructor should initialize all the data fields in Student class based on your
information and your current semester's enrolled courses.
c. Override 6 methods in Student class, as marked in the source code comments. Please
read the comment sections carefully for descriptions.
d. Override the equals() method in MyCourse class. Two courses are equal if they have the
same course number, course name, and credit hours. You should use this method for all
courses comparisons.
e. Create a customized exception class CourseNotFoundException, throw this exception in
the dropCourse() method if the dropping course doesn't exist in the courseList. The
thrown exception object should contain a meaningful message, including course number,
course name, and credit hours for the not found course. (hint: I demo the
InvalidSideException in Feb 24th lecture, please review for reference)](https://content.bartleby.com/qna-images/question/0e20848f-885a-4953-aa62-49de56df484f/96c656fc-debd-492c-8715-653626700df9/j1nzogf_thumbnail.jpeg)
![|3] Sample outputs for printBasicInfo() and printCourseList() methods:
John_Smith js = new John_Smith();
js.printBasicInfo();
Full Name: John Smith
Gender: Male
Phone Number: (210)000-0000
Email: jsmith@jaguar.tamu.edu
JNumber: JO00000
js.printCourseList();
John Smith's Course List
CSCI 1437 Programming Fundamentals II
4 hrs
CISA 2306 Computer Networks
3 hrs
CISA 2356 Systems Analysis and Design
3 hrs
Total
10 hrs](https://content.bartleby.com/qna-images/question/0e20848f-885a-4953-aa62-49de56df484f/96c656fc-debd-492c-8715-653626700df9/7lrpvn8_thumbnail.jpeg)

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Focus on classes, objects, methods and good programming style Your task is to create a BankAccount class(See the pic attached) The bank account will be protected by a 4-digit pin number (i.e. between 1000 and 9999). The pin should be generated randomly when the account object is created. The initial balance should be 0. get_pin()should return the pin. check_pin(pin) should check the argument against the saved pin and return True if it matches, False if it does not. deposit(amount) should receive the amount as the argument, add the amount to the account and return the new balance. withraw(amount) should check if the amount can be withdrawn (not more than is in the account), If so, remove the argument amount from the account and return the new balance if the transaction was successful. Return False if it was not. get_balance() should return the current balance. Finally, write a main() to demo your bank account class. Present a menu offering a few actions and perform the action the user…arrow_forwardCourse Title: Modern Programming Language Please Java Language Code Question : Create a class called with your 18Arid2891, as Invoice18Arid2891, that a hardware store might use to represent an invoice for an item sold at the store. An Invoice should include four pieces of information as instance variables a part number (type String), a part description (type String),a quantity of the item being purchased (type int) and a price per item (double). Your class should have a constructor that initializes the four instance variables. Provide a set and a get method for each instance variable. In addition, provide a method named getInvoice Amount that calculates the invoice amount (i.e., multiplies the quantity by the price per item), then returns the amount as a double value. If the quantity is not positive, it should be set to 0. If the price per item is not positive, it should be set to 0.0. Write a test application named InvoiceTestMJibranAkram that demonstrates class Invoice’s…arrow_forwardAssignment:The BankAccount class models an account of a customer. A BankAccount has the followinginstance variables: A unique account id sequentially assigned when the Bank Account is created. A balance which represents the amount of money in the account A date created which is the date on which the account is created.The following methods are defined in the BankAccount class: Withdraw – subtract money from the balance Deposit – add money to the balance Inquiry on:o Balanceo Account ido Date createdarrow_forward
- public class ItemToPurchase { private String itemName; private int itemPrice; private int itemQuantity; // Default constructor public ItemToPurchase() { itemName = "none"; itemPrice = 0; itemQuantity = 0; } // Mutator for itemName public void setName(String itemName) { this.itemName = itemName; } // Accessor for itemName public String getName() { return itemName; } // Mutator for itemPrice public void setPrice(int itemPrice) { this.itemPrice = itemPrice; } // Accessor for itemPrice public int getPrice() { return itemPrice; } // Mutator for itemQuantity public void setQuantity(int itemQuantity) { this.itemQuantity = itemQuantity; } // Accessor for itemQuantity public int getQuantity() { return itemQuantity; }} import java.util.Scanner; public class ShoppingCartPrinter { public static void main(String[] args) { Scanner scnr = new…arrow_forwardJava prgm basedarrow_forwardclass Artist{StringsArtist(const std::string& name="",int age=0):name_(name),age_(age){}std::string name()const {return name_;}void set_name(const std::string& name){name_=name;}int age()const {return age_;}void set_age(int age){age_=age;}virtual std::string perform(){return std::string("");}private:int age_;std::string name_;};class Singer : public Artist{public:Singer():Artist(){};Singer(const std::string& name,int age,int hits);int hits() const{return hits_;}void set_hits(int hit);std::string perform();int operator+(const Singer& rhs);int changeme(std::string name,int age,int hits);Singer combine(const Singer& rhs);private:int hits_=0;};int FindOldestandMostSuccess(std::vector<Singer> list); Task: Implement the function int Singer::changeme(std::string name,int age,int hits) : This function should check if the values passed by name, age and hits are different than those stored. And if this is the case it should change the values. This should be done by…arrow_forward
- public class Plant { protected String plantName; protected String plantCost; public void setPlantName(String userPlantName) { plantName = userPlantName; } public String getPlantName() { return plantName; } public void setPlantCost(String userPlantCost) { plantCost = userPlantCost; } public String getPlantCost() { return plantCost; } public void printInfo() { System.out.println(" Plant name: " + plantName); System.out.println(" Cost: " + plantCost); }} public class Flower extends Plant { private boolean isAnnual; private String colorOfFlowers; public void setPlantType(boolean userIsAnnual) { isAnnual = userIsAnnual; } public boolean getPlantType(){ return isAnnual; } public void setColorOfFlowers(String userColorOfFlowers) { colorOfFlowers = userColorOfFlowers; } public String getColorOfFlowers(){ return colorOfFlowers; } @Override public void printInfo(){…arrow_forwardclass Artist{StringsArtist(const std::string& name="",int age=0):name_(name),age_(age){}std::string name()const {return name_;}void set_name(const std::string& name){name_=name;}int age()const {return age_;}void set_age(int age){age_=age;}virtual std::string perform(){return std::string("");}private:int age_;std::string name_;};class Singer : public Artist{public:Singer():Artist(){};Singer(const std::string& name,int age,int hits);int hits() const{return hits_;}void set_hits(int hit);std::string perform();int operator+(const Singer& rhs);int changeme(std::string name,int age,int hits);Singer combine(const Singer& rhs);private:int hits_=0;};int FindOldestandMostSuccess(std::vector<Singer> list); Implement the function Singer Singer::combine(const Singer& rhs):It should create a new Singer object, by calling the constructor with the following values: For name it should pass a combination of be the name of the calling object followed by a '+' and then…arrow_forwardinterface StudentsADT{void admissions();void discharge();void transfers(); }public class Course{String cname;int cno;int credits;public Course(){System.out.println("\nDEFAULT constructor called");}public Course(String c){System.out.println("\noverloaded constructor called");cname=c;}public Course(Course ch){System.out.println("\nCopy constructor called");cname=ch;}void setCourseName(String ch){cname=ch;System.out.println("\n"+cname);}void setSelectionNumber(int cno1){cno=cno1;System.out.println("\n"+cno);}void setNumberOfCredits(int cdit){credits=cdit;System.out.println("\n"+credits);}void setLink(){System.out.println("\nset link");}String getCourseName(){System.out.println("\n"+cname);}int getSelectionNumber(){System.out.println("\n"+cno);}int getNumberOfCredits(){System.out.println("\n"+credits); }void getLink(){System.out.println("\ninside get link");}} public class Students{String sname;int cno;int credits;int maxno;public Students(){System.out.println("\nDEFAULT constructor…arrow_forward
- PYTHON # TODO: Define BankAccount class # TODO: Define constructor with parameters to initialize instance attributes # TODO: Define deposit_checking() # TODO: Define deposit_savings() # TODO: Define withdraw_checking() # TODO: Define withdraw_savings() # TODO: Define transfer_to_savings() if __name__ == "__main__": account = BankAccount("Mickey", 500.00, 1000.00) account.checking_balance = 500 account.savings_balance = 500 account.withdraw_savings(100) account.withdraw_checking(100) account.transfer_to_savings(300) print(account.name) print('${:.2f}'.format(account.checking_balance)) print('${:.2f}'.format(account.savings_balance))arrow_forwardclass Student: def __init__(self, id, fn, ln, dob, m='undefined'): self.id = id self.firstName = fn self.lastName = ln self.dateOfBirth = dob self.Major = m def set_id(self, newid): #This is known as setter self.id = newid def get_id(self): #This is known as a getter return self.id def set_fn(self, newfirstName): self.fn = newfirstName def get_fn(self): return self.fn def set_ln(self, newlastName): self.ln = newlastName def get_ln(self): return self.ln def set_dob(self, newdob): self.dob = newdob def get_dob(self): return self.dob def set_m(self, newMajor): self.m = newMajor def get_m(self): return self.m def print_student_info(self): print(f'{self.id} {self.firstName} {self.lastName} {self.dateOfBirth} {self.Major}')all_students = []id=100user_input = int(input("How many students: "))for x in range(user_input): firstName = input('Enter…arrow_forwardTrace through the following program and show the output. Show your work for partial credit. public class Employee { private static int empID = 1111l; private String name , position; double salary; public Employee(String name) { empID ++; this.name 3 пате; } public Employee(Employee obj) { empID = obj.empĪD; пате %3D оbj.naте; position = obj.position; %3D public void empPosition(String empPosition) {position = empPosition;} public void empSalary(double empSalary) { salary = empSalary;} public String toString() { return name + " "+empID + " "+ position +" $"+salary; public void setName(String empName){ name = empName;} public static void main(String args[]) { Employee empOne = new Employee("James Smith"), empTwo; %3D empOne.empPosition("Senior Software Engineer"); етрOпе.етpSalary(1000); System.out.println(empOne); еmpTwo empTwo.empPosition("CEO"); System.out.println(empOne); System.out.println(empTwo); %3D етpОпе empOne ;arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
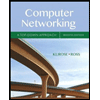
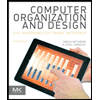
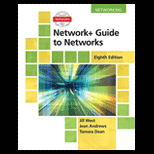
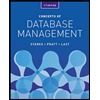
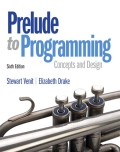
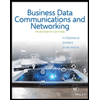