Your task is to modify the selection sort algorithm so that you find the largest element and swap it to the last position, then continue with the next-largest element and so on. SelectionSorter.java /** 1 2 3 4 */ 5 public class SelectionSorter 6 { 7 8 9 10 11 12 13 METRARHAMME: 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 This class sorts an array, using a modified selection sort algorithm 40 /** */ public SelectionSorter (int[] anArray) { } Constructs a selection sorter. @param anArray the array to sort } /** Sorts the array managed by this selection sorter. */ public void sort() { /** a = anArray; // TODO: add loop bounds for (int i = ...; i > ...; i--) { } /** } int maxPos maximumPosition (i); swap (maxPos, i); Finds the largest element in a subrange of the array. @param to the last position in a to compare @return the position of the largest element in the range a[0]. . . a[to] */ private int maximumPosition (int to) { // your work here
Your task is to modify the selection sort algorithm so that you find the largest element and swap it to the last position, then continue with the next-largest element and so on. SelectionSorter.java /** 1 2 3 4 */ 5 public class SelectionSorter 6 { 7 8 9 10 11 12 13 METRARHAMME: 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 This class sorts an array, using a modified selection sort algorithm 40 /** */ public SelectionSorter (int[] anArray) { } Constructs a selection sorter. @param anArray the array to sort } /** Sorts the array managed by this selection sorter. */ public void sort() { /** a = anArray; // TODO: add loop bounds for (int i = ...; i > ...; i--) { } /** } int maxPos maximumPosition (i); swap (maxPos, i); Finds the largest element in a subrange of the array. @param to the last position in a to compare @return the position of the largest element in the range a[0]. . . a[to] */ private int maximumPosition (int to) { // your work here
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
In JAVA
![Your task is to modify the selection sort algorithm so that you find the largest element and
swap it to the last position, then continue with the next-largest element and so on.
SelectionSorter.java
/**
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
36 37 38 39 40 412344 45 46 7 48 49 50 152 3 4 55 56 望 58 59 60 1
47
53
54
57
This class sorts an array, using a modified selection sort
algorithm
*/
public class SelectionSorter
{
/**
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
*/
35 private int maximumPosition (int to).
// your work here
61 }
*/
public SelectionSorter (int[] anArray)
{
}
/**
Sorts the array managed by this selection sorter.
*/
public void sort()
{
}
{
}
*/
Constructs a selection sorter.
@param anArray the array to sort
{
a = anArray;
/**
}
// TODO: add loop bounds
for (int i = ...; i > ...; i--)
{
{
private void swap(int i, int j)
}
int maxPos maximumPosition (i);
swap (maxPos, i);
Finds the largest element in a subrange of the array.
@param to the last position in a to compare
@return the position of the largest element in the
range a [0] ... a[to]
Swaps two entries of the array.
@param i the first position to swap
@param j the second position to swap
private
int[] a;
// this method is used to check your work
public static int[] check(int[] values)
int temp = a[i];
a[i] = a[j];
a[j] = temp;
SelectionSorter sorter = new Selection Sorter (values);
sorter.sort();
return values;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1580620b-ec3d-48da-8d5f-e0e1d6dd1757%2F5bc1f149-935e-45f0-a9bf-811c9acfb29d%2Fkb6pozq_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Your task is to modify the selection sort algorithm so that you find the largest element and
swap it to the last position, then continue with the next-largest element and so on.
SelectionSorter.java
/**
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
36 37 38 39 40 412344 45 46 7 48 49 50 152 3 4 55 56 望 58 59 60 1
47
53
54
57
This class sorts an array, using a modified selection sort
algorithm
*/
public class SelectionSorter
{
/**
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
*/
35 private int maximumPosition (int to).
// your work here
61 }
*/
public SelectionSorter (int[] anArray)
{
}
/**
Sorts the array managed by this selection sorter.
*/
public void sort()
{
}
{
}
*/
Constructs a selection sorter.
@param anArray the array to sort
{
a = anArray;
/**
}
// TODO: add loop bounds
for (int i = ...; i > ...; i--)
{
{
private void swap(int i, int j)
}
int maxPos maximumPosition (i);
swap (maxPos, i);
Finds the largest element in a subrange of the array.
@param to the last position in a to compare
@return the position of the largest element in the
range a [0] ... a[to]
Swaps two entries of the array.
@param i the first position to swap
@param j the second position to swap
private
int[] a;
// this method is used to check your work
public static int[] check(int[] values)
int temp = a[i];
a[i] = a[j];
a[j] = temp;
SelectionSorter sorter = new Selection Sorter (values);
sorter.sort();
return values;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
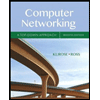
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
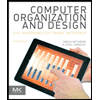
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
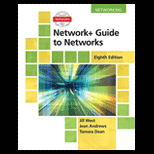
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
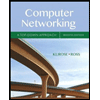
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
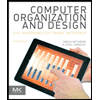
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
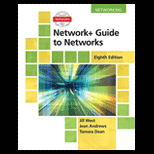
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
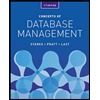
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
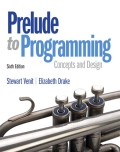
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
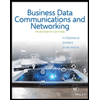
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY