Your tasks Implement the methods marked with ??? package percentiles: /** * Given a non-empty sequence of elements of type T, returns the smallest element e * in the sequence for which it holds that at least p percent of the elements in the sequence * are smaller or equal to e. * Note the special cases: * - when p == 0, the result should be the smallest element in the sequence * - when p == 100, the result should be the largest element in the sequence * If the sequence is empty, java.lang.IllegalArgumentException is thrown. * * For a sequence of length n, the method should make at most n*log(n) * [again, here log means base-2 logarithm] comparison operations between * the elements in the sequence. * Furthermore, assuming that co
Your tasks Implement the methods marked with ??? package percentiles: /** * Given a non-empty sequence of elements of type T, returns the smallest element e * in the sequence for which it holds that at least p percent of the elements in the sequence * are smaller or equal to e. * Note the special cases: * - when p == 0, the result should be the smallest element in the sequence * - when p == 100, the result should be the largest element in the sequence * If the sequence is empty, java.lang.IllegalArgumentException is thrown. * * For a sequence of length n, the method should make at most n*log(n) * [again, here log means base-2 logarithm] comparison operations between * the elements in the sequence. * Furthermore, assuming that co
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Your tasks
Implement the methods marked with ???
package percentiles:
/**
* Given a non-empty sequence of elements of type T, returns the smallest element e
* in the sequence for which it holds that at least p percent of the elements in the sequence
* are smaller or equal to e.
* Note the special cases:
* - when p == 0, the result should be the smallest element in the sequence
* - when p == 100, the result should be the largest element in the sequence
* If the sequence is empty, java.lang.IllegalArgumentException is thrown.
*
* For a sequence of length n, the method should make at most n*log(n)
* [again, here log means base-2 logarithm] comparison operations between
* the elements in the sequence.
* Furthermore, assuming that comparison is a constant-time operation,
* the whole method should run in O(n*log(n)) time.
* Hint: you can assume that the "sorted" method of Seq performs at most n*log(n) comparisons for
* sequences of length n (the real underlying implementation of "sorted" is currently
* http://docs.oracle.com/javase/7/docs/api/java/util/Arrays.html#sort%28T[],%20java.util.Comparator%29 )
*
* Note that there are alternative definitions for "percentile",
* see e.g. http://en.wikipedia.org/wiki/Percentile
*
* Advanced (non-obligatory) stuff: for the implicit keyword below, one can consult, for instance,
* http://www.artima.com/pins1ed/implicit-conversions-and-parameters.html
*/
def percentile[T](p: Int)(seq: Seq[T])(implicit ord: Ordering[T]): T =
require(0 <= p && p <= 100)
require(!seq.isEmpty, "cannot compute percentiles on an empty sequence")
???
end percentile
/**
* Calculate the 10th percentile.
*/
def percentile10[T](seq: Seq[T])(implicit ord: Ordering[T]): T = percentile(10)(seq)
/**
* Calculate the median.
*/
def median[T](seq: Seq[T])(implicit ord: Ordering[T]): T = percentile(50)(seq)
/**
* Calculate the 90th percentile.
*/
def percentile90[T](seq: Seq[T])(implicit ord: Ordering[T]): T = percentile(90)(seq)
/*
* The following functions are only provided for "additional reading"
* to illustrate the use of implicit keyword.
* You can safely ignore them if you wish.
*/
/** Average value of a sequence of Ints*/
def averageInt(seq: Seq[Int]): Double = if seq.isEmpty then 0.0 else seq.sum / seq.length
/** Average value of a sequence of Doubles */
def averageDouble(seq: Seq[Double]): Double = if seq.isEmpty then 0.0 else seq.sum / seq.length
/**
* With implicit conversions and the Numeric package, we can do a generic average
* value function that works for all types T that can be implicitly translated into Numeric[T].
* Performance-wise this is not as good as the specialized methods above!
*/
def average[T](seq: Seq[T])(implicit conv: Numeric[T]): Double =
if seq.isEmpty then 0.0
else
val sum = seq.foldLeft[T](conv.zero)((s, e) => conv.plus(s, e))
conv.toDouble(sum) / seq.length
end average
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
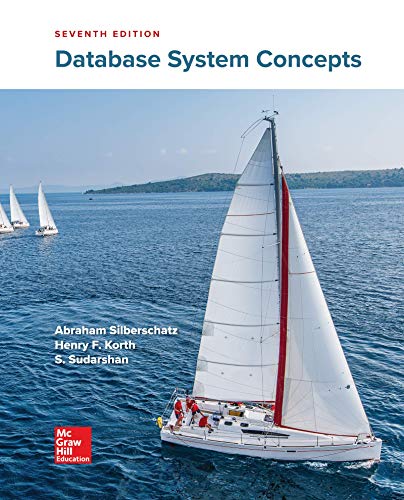
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
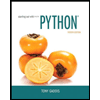
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
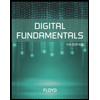
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
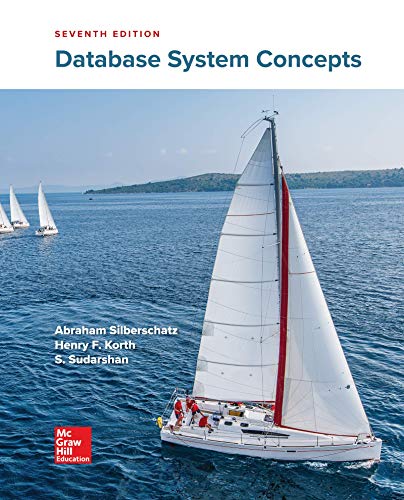
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
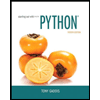
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
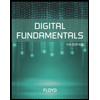
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
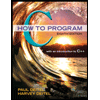
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
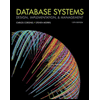
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
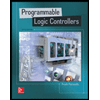
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education