(1), (2), and (3) is accepted by the Scala compiler Question 26: Consider the following Scala code: class A { def f (println ("A") } class B extends A { override def f () System.out.println ("B") } class C extends B { override def f ()= System.out.println ("C") } val b:B= new C () val a: A - b a.f () What happens when we try to execute this code? A: The code fails at runtime at the line val a: A b because of a type error B: B is printed C: C is printed D: The code fails to compile at the line val a: A =b because of a type error E: A is printed Question 27: Consider the Scala code: val q (x: Int) => { var y: Int=0; y = y + x; y } = What will happen when q(10) and then q (20) are executed? A: Returns 10 and 20 in that order. B: Returns 10 and 30 in that order. C: Returns 0 and 30 in that order. D: Returns 30 and 30 in that order. E: Returns 0 and 0 in that order.
(1), (2), and (3) is accepted by the Scala compiler Question 26: Consider the following Scala code: class A { def f (println ("A") } class B extends A { override def f () System.out.println ("B") } class C extends B { override def f ()= System.out.println ("C") } val b:B= new C () val a: A - b a.f () What happens when we try to execute this code? A: The code fails at runtime at the line val a: A b because of a type error B: B is printed C: C is printed D: The code fails to compile at the line val a: A =b because of a type error E: A is printed Question 27: Consider the Scala code: val q (x: Int) => { var y: Int=0; y = y + x; y } = What will happen when q(10) and then q (20) are executed? A: Returns 10 and 20 in that order. B: Returns 10 and 30 in that order. C: Returns 0 and 30 in that order. D: Returns 30 and 30 in that order. E: Returns 0 and 0 in that order.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Scala programming
![Serial Number: 500
Name:
c2.update ()
c2.update ()
What will happen when c1.get()+c2.get() is executed?
A: Returns 40.
B: Returns 4.
C: Returns 22.
D: Returns 0.
E: Returns 44.
Question 24: Consider the following code in Scala:
val n = 3
def createList (init: Int): List [Int] = {
def aux (start: Int, count: Int): List [Int] = {
if (count == 0) {
Nil
} else {
start aux (start + 1, count - 1)
}
}
aux (init, n)
}
val xs List [Int] = {
val n = 5
createList (2)
}
What will the value of xs be?
A: List (2, 3, 4, 5, 6)
B: List (2, 3, 4)
C: List (2)
D: List (2, 3, 4, 5)
E: List (2, 3)
Question 25: Consider the following Scala code (where... indicates omitted code):
class Shape
{...}
class Triangle extends Shape {...}
val shapes List [Shape] List (new Triangle, new Triangle) // (1)
val triangles
List [Triangle] = shapes
// (2)
// (3)
val shapes2: List [Shape] = triangles
Which of the above lines of code are accepted by the Scala compiler when it typechecks the code? You should
answer for (2) based on the variable shapes having type List [Shape]. Similarly, you should answer for (3) based
on the variable triangles having type List [Triangle].](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F57e74000-fbe3-481d-ab8d-800d62a3b02c%2F1da2730f-3bbf-495a-8882-7ad16b0f4d57%2F3h1piuj_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Serial Number: 500
Name:
c2.update ()
c2.update ()
What will happen when c1.get()+c2.get() is executed?
A: Returns 40.
B: Returns 4.
C: Returns 22.
D: Returns 0.
E: Returns 44.
Question 24: Consider the following code in Scala:
val n = 3
def createList (init: Int): List [Int] = {
def aux (start: Int, count: Int): List [Int] = {
if (count == 0) {
Nil
} else {
start aux (start + 1, count - 1)
}
}
aux (init, n)
}
val xs List [Int] = {
val n = 5
createList (2)
}
What will the value of xs be?
A: List (2, 3, 4, 5, 6)
B: List (2, 3, 4)
C: List (2)
D: List (2, 3, 4, 5)
E: List (2, 3)
Question 25: Consider the following Scala code (where... indicates omitted code):
class Shape
{...}
class Triangle extends Shape {...}
val shapes List [Shape] List (new Triangle, new Triangle) // (1)
val triangles
List [Triangle] = shapes
// (2)
// (3)
val shapes2: List [Shape] = triangles
Which of the above lines of code are accepted by the Scala compiler when it typechecks the code? You should
answer for (2) based on the variable shapes having type List [Shape]. Similarly, you should answer for (3) based
on the variable triangles having type List [Triangle].
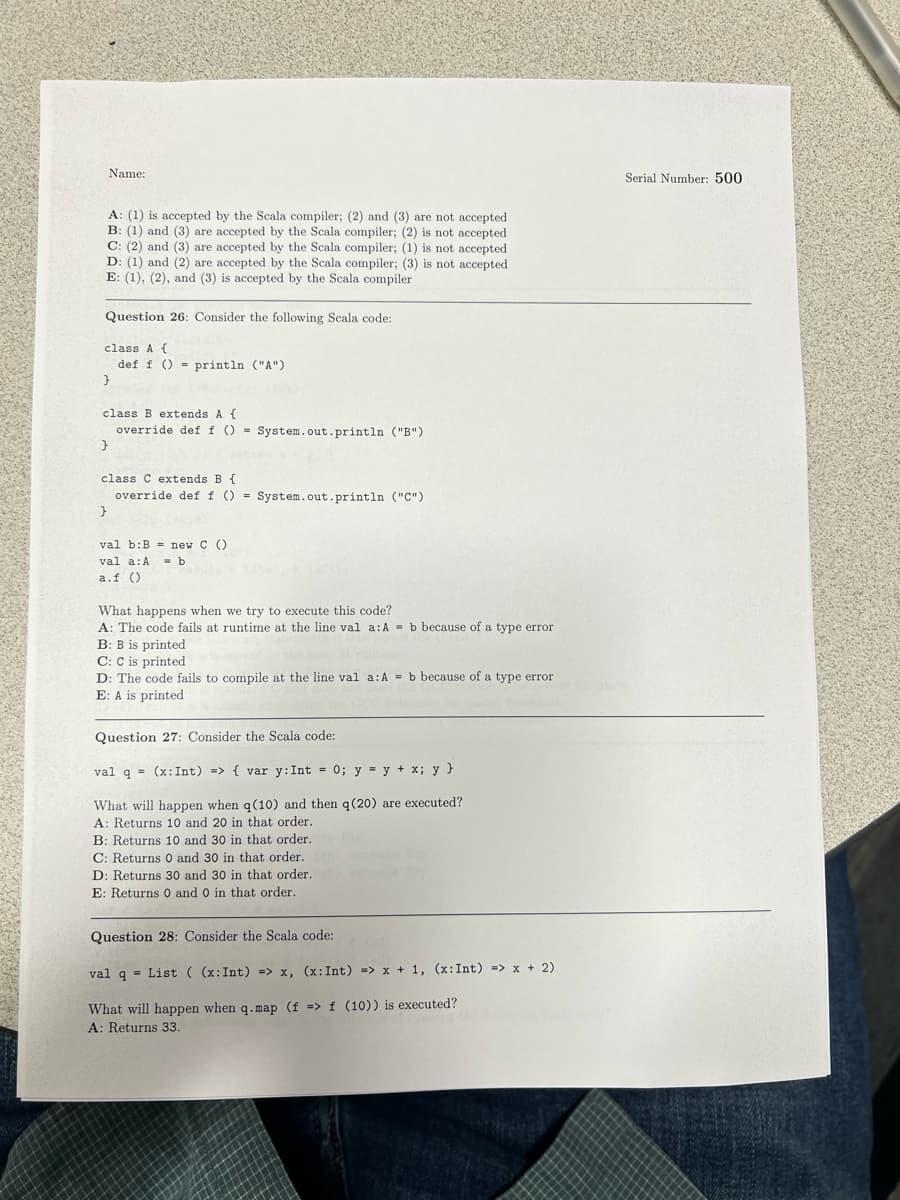
Transcribed Image Text:Name:
A: (1) is accepted by the Scala compiler; (2) and (3) are not accepted.
B: (1) and (3) are accepted by the Scala compiler; (2) is not accepted
C: (2) and (3) are accepted by the Scala compiler; (1) is not accepted
D: (1) and (2) are accepted by the Scala compiler; (3) is not accepted
E: (1), (2), and (3) is accepted by the Scala compiler
Question 26: Consider the following Scala code:
class A {
def f() println ("A")
}
class B extends A {
override def f () = System.out.println ("B")
class C extends B {
override def f () = System.out.println ("C").
}
val b: B = new C ()
val a: A
b
a.f ()
What happens when we try to execute this code?
A: The code fails at runtime at the line val a: A = b because of a type error
B: B is printed
C: C is printed
D: The code fails to compile at the line val a: A b because of a type error
E: A is printed
Question 27: Consider the Scala code:
val q (x: Int) => { var y: Int = 0; y = y + x; y }
What will happen when q(10) and then q (20) are executed?
A: Returns 10 and 20 in that order.
B: Returns 10 and 30 in that order.
C: Returns 0 and 30 in that order.
D: Returns 30 and 30 in that order.
E: Returns 0 and 0 in that order.
Question 28: Consider the Scala code:
val q = List ( (x: Int) => x, (x: Int) => x + 1, (x: Int) => x + 2)
What will happen when q.map (f => f (10)) is executed?
A: Returns 33.
Serial Number: 500
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
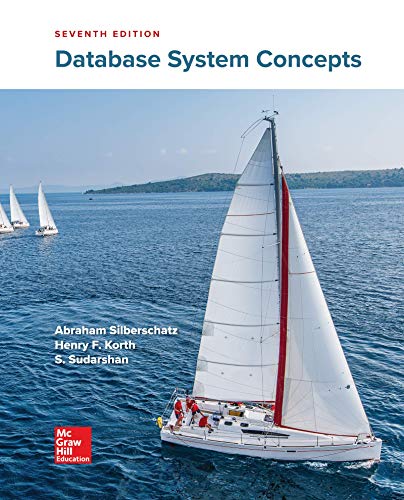
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
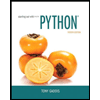
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
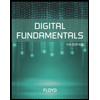
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
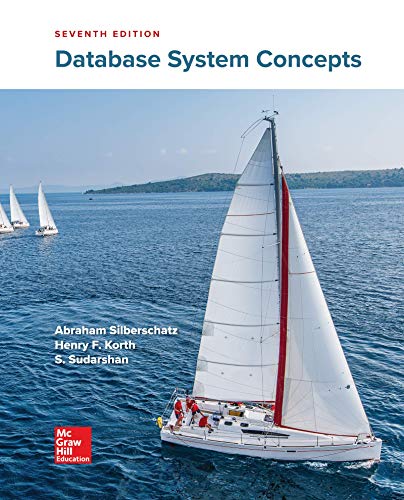
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
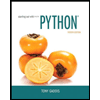
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
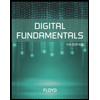
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
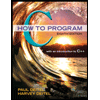
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
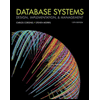
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
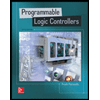
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education