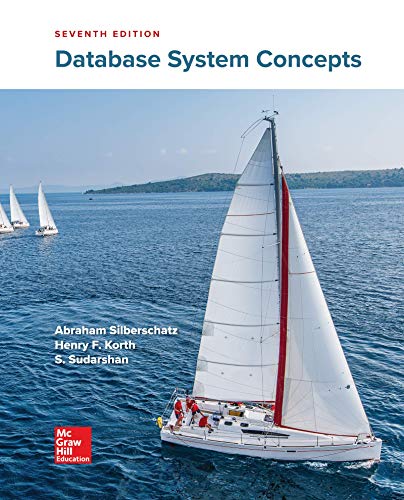
Concept explainers
how can i remove the spaces at the end of each line in my code ?
code:
def load_course(course_name):
try:
file = open(course_name+'.txt')
return file.readlines()
except FileNotFoundError as e:
return None
def word_wrap_text(text,lineWidth):
words = list(text.split())
linelen = 0
lines = []
line = ''
for word in words:
if linelen + len(word) > lineWidth:
lines.append(line)
line = ''
line += word + ' '
linelen = len(line)
if line !='':
lines.append(line)
return '\n'.join(lines)
def enhanced_word_wrap_text(text,lineWidth):
words = list(text.split())
linelen = 0
lines = []
line = ''
for word in words:
if linelen + len(word) + 1 > lineWidth:
lines.append(line + word[:lineWidth-linelen])
line = word[lineWidth-linelen:] + ' '
else:
line += word + ' '
linelen = len(line)
if line != '':
lines.append(line)
return '\n'.join(lines)
lineWidth = int(input(""))
while True:
course = input("")
if course == 'q':
break
lines = load_course(course)
if lines == None:
print('Unable to load course information for course',course)
else:
for line in lines:
print(word_wrap_text(line,lineWidth),'\n')
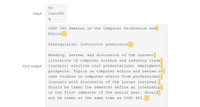

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- You may have found it somewhat tedious and unpleasant to use the debugger and visualizer to verify the correctness of your addFirst and addLast methods. There is also the problem that such manual verification becomes stale as soon as you change your code. Imagine that you made some minor but uncertain change to addLast]. To verify that you didn't break anything you'd have to go back and do that whole process again. Yuck. What we really want are some automated tests. But unfortunately there's no easy way to verify correctness of addFirst and addLast] if those are the only two methods we've implemented. That is, there's currently no way to iterate over our list and get bad its values and see that they are correct. That's where the toList method comes in. When called, this method returns a List representation of the Deque. For example, if the Deque has had addLast (5) addLast (9) addLast (10), then addFirst (3) called on it, then the result of toList() should be a List with 3 at the…arrow_forwardFor any element in keysList with a value smaller than 40, print the corresponding value in itemsList, followed by a comma (no spaces). Ex: If keysList = {32, 105, 101, 35} and itemsList = {10, 20, 30, 40}, print: 10,40, #include <iostream>#include <string.h>using namespace std; int main() { const int SIZE_LIST = 4; int keysList[SIZE_LIST]; int itemsList[SIZE_LIST]; int i; cin >> keysList[0]; cin >> keysList[1]; cin >> keysList[2]; cin >> keysList[3]; cin >> itemsList[0]; cin >> itemsList[1]; cin >> itemsList[2]; cin >> itemsList[3]; /* Your code goes here */ cout << endl; return 0;}arrow_forwardImplement the following: Given: names = ['ann', 'bill', 'calvin', 'david']ids = [12, 34, 56, 78] 1) Create a dictionary, stuInfo by using the two given lists above.2) Use a loop to print all keys and values in the dictionary stulfno. 3) Print the dictionary, stuInfo. Example Output12 ANN34 BILL 56 CALVIN78 DAVID{'ann': 12, 'bill': 34, 'calvin': 56, 'david': 78} NEED HELP WITH PYTHONarrow_forward
- Here is the code for number 1. def reverse_list(old_list): new_list = [ele for ele in reversed(old_list)] return new_list if __name__ == "__main__": str = input("Enter the elements of the list: ").split() elements = list(map(int, str)) print(reverse_list(elements))arrow_forward19. Given the following linked list: LinkedList list = new LinkedList(); And this code: 1. list.add(1.5); 2. list.addFirst(11.3); 3. list.add(6.2); 4. list.add(3.4); 5. list.addLast(4.8); 6. list.add(7.4); 7. list.remove(1.5); 8. list.remove(3.4); 9. list.removeFirst); 10. System.out.println(list.getFirst(); Answer the following questions regarding the code: a) What is the list after line 6? b) What is the list after line 9? c) Line 10 gives what value?arrow_forwardI need help with the last two functions in pythonarrow_forward
- int size; double[] list = new double[size]; Your answerarrow_forwardint [] myArray = {2,3,4,5}: What is the length of myArray? type your answer.arrow_forwardWrite a program that will take this dictionary: gradePoints = {"A":4,"B":3,"C":2,"D":1,"F":0} and this list: courseList = ["CST 161","Mat 144","ENG 201","PSY 101","HIS 101"] and randomly use elements from each of these lists : gradeList = ["A","B","C","D","F"] creditList = [3,4] and produce a grade point average. Academic average is determined by dividing the total number of qualitypoints earned by the total number of credits taken, whether passed orfailed. The following table is an illustration of this computation:GRADE & QUALITY NUMERICAL POINTSCourse . . . Credits Value Per CourseHIS 101. . . . . .3 C (2) 6PSY 101. . . . . 3 B (3) 9MAT 144. . . . 4 D (1) 4ENG 201. . . . 3 B+ (3.5) 10.5CST 161. . . . . 3 F (0) 0Total credits taken: 16Total quality points earned 29.529.5 divided by 16 results in a 1.8 gradepoint average. Here the gradeList element will be embedded as the key in referencing the grade points. (e.g. gPoints = gradePoints[random gradeList element])…arrow_forward
- 1. How can values in a pandas Series method be accessed as a Python list? using the tolist) method using the getlist() method using the list constructor any of these 2. For a DataFrame df, how can its first row be accessed? df[O] df.loc[0] df.iloc[0] any of thesearrow_forwardmyStrList = ["this", "is", "my", "str", "list", 0] for s in myStrList: print(s)arrow_forwardWhich element is stored at index 1 after the following sequence? ArrayList<String>groceryList;groceryList=newArrayList<String>();groceryList.add("Bread");groceryList.add("Apples");groceryList.add("Grape Jelly");groceryList.add(1,"Peanut Butter");groceryList.set(1,"Frozen Pizza");groceryList.remove(1); Bread Peanut Butter Apples Frozen Pizza For the given code, when is numType.setText() called? publicclassOddEvenextendsJFrameimplementsChangeListener{privateJSpinnernumSpinner;privateJTextFieldnumType;...numSpinner.addChangeListener(this);...@OverridepublicvoidstateChanged(ChangeEventevent){IntegernumValue;numValue=(Integer)numSpinner.getValue();if(numValue%2==0){numType.setText("Even");}else{numType.setText("Odd");}}...} Every time numSpinner's value changes Only the first time numSpinner's value changes Every time numSpinner's…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
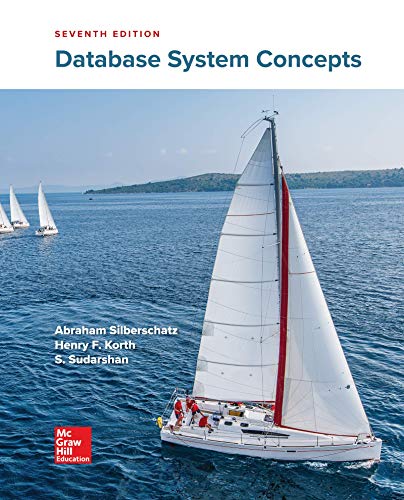
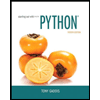
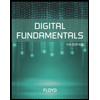
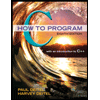
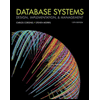
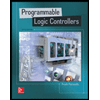