1) Create a class called Course. It will not have a main method; it is a business class. 2) Put in your documentation comments at the top. Be sure to include your name. 3) Course must have the following instance variables named as shown in the table: Variable name crn subject number sectionld creditHours instructor isAvailable Description A unique number given each semester to a section (stands for Course Registration Number) - int A 4-letter abbreviation for the course (e.g., ITEC, PHED, CHEM) A 4-digit number associated with the course (e.g., 1001, 0099) - int A 2-digit number associated with a particular section of a course (e.g., 01, 13, 47) -int Number of credit hours the course is worth. Valid values are 0, 1, 2, 3, or 4. The default value is 3. -int First and last name of the instructor teaching the course. If the instructor is not known, this should be listed as "TBA" - String Value is either true or false based upon whether the course is available for registration. Default is false. - boolean 4) Create a constructor that has the crn, subject, number, and sectionld as String. Initialize the other instance variables based upon the default values in the table above. Call the appropriate setters from #7 to validate data. 5) Add a 7-argument constructor that uses all parameter variables as Strings to set the instance variables. Make sure your constructor calls the setters from #7, to ensure all data is validated. (All arguments will be of type String.) 6) Generate all the getters and setters 7) Changes to make to generated getters and setters to make sure data is valid: • public void setCrn(String input) - if input consists of all digits, convert input to integer. Use this integer to set the CRN. Otherwise, set the CRN to 9001 and display an error message that "Invalid value typed for CRN. Defaulting to 9001." public void setNumber(String input) - if input consists of all digits and is in the range of 1 - 9999, convert input to integer. Use this integer to set the course number. Otherwise, set the course number to 1001 and display an error message that "Invalid value typed for course number. Defaulting to 1001." • public void setSectionId(String input) -if input consists of all digits and is in the range of 1-80, convert input to integer. Use this integer to set the section Id. Otherwise, set the section ID to 1 and display an error message that "Invalid value typed for section ID. Defaulting to 1." • public void setCreditHours(String input) -if input consists of all digits and is in the range of 0 - 4, convert input to integer. Use this integer to set the number of credit hours. Otherwise, set the credit hours to 3 and display an error message that "Invalid value typed for credit hours. Defaulting to 3." • public void setlsAvailable(String input) - if input starts with 't', 'T', 'y', or "Y", set Available to true. Otherwise, set is Available to false. 8) Create the toString method
1) Create a class called Course. It will not have a main method; it is a business class. 2) Put in your documentation comments at the top. Be sure to include your name. 3) Course must have the following instance variables named as shown in the table: Variable name crn subject number sectionld creditHours instructor isAvailable Description A unique number given each semester to a section (stands for Course Registration Number) - int A 4-letter abbreviation for the course (e.g., ITEC, PHED, CHEM) A 4-digit number associated with the course (e.g., 1001, 0099) - int A 2-digit number associated with a particular section of a course (e.g., 01, 13, 47) -int Number of credit hours the course is worth. Valid values are 0, 1, 2, 3, or 4. The default value is 3. -int First and last name of the instructor teaching the course. If the instructor is not known, this should be listed as "TBA" - String Value is either true or false based upon whether the course is available for registration. Default is false. - boolean 4) Create a constructor that has the crn, subject, number, and sectionld as String. Initialize the other instance variables based upon the default values in the table above. Call the appropriate setters from #7 to validate data. 5) Add a 7-argument constructor that uses all parameter variables as Strings to set the instance variables. Make sure your constructor calls the setters from #7, to ensure all data is validated. (All arguments will be of type String.) 6) Generate all the getters and setters 7) Changes to make to generated getters and setters to make sure data is valid: • public void setCrn(String input) - if input consists of all digits, convert input to integer. Use this integer to set the CRN. Otherwise, set the CRN to 9001 and display an error message that "Invalid value typed for CRN. Defaulting to 9001." public void setNumber(String input) - if input consists of all digits and is in the range of 1 - 9999, convert input to integer. Use this integer to set the course number. Otherwise, set the course number to 1001 and display an error message that "Invalid value typed for course number. Defaulting to 1001." • public void setSectionId(String input) -if input consists of all digits and is in the range of 1-80, convert input to integer. Use this integer to set the section Id. Otherwise, set the section ID to 1 and display an error message that "Invalid value typed for section ID. Defaulting to 1." • public void setCreditHours(String input) -if input consists of all digits and is in the range of 0 - 4, convert input to integer. Use this integer to set the number of credit hours. Otherwise, set the credit hours to 3 and display an error message that "Invalid value typed for credit hours. Defaulting to 3." • public void setlsAvailable(String input) - if input starts with 't', 'T', 'y', or "Y", set Available to true. Otherwise, set is Available to false. 8) Create the toString method
Chapter3: Using Methods, Classes, And Objects
Section: Chapter Questions
Problem 13PE
Related questions
Question
USE BEGINNER LEVEL JAVA!!!! BASIC JAVA!!!
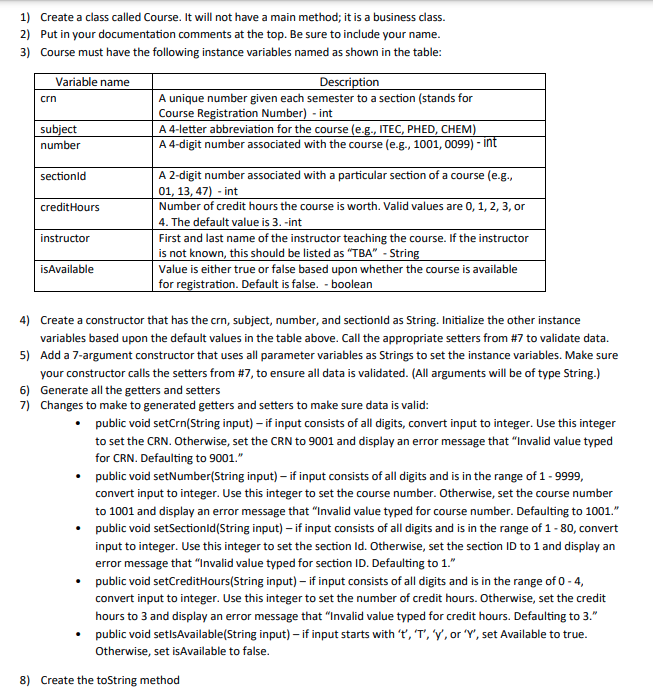
Transcribed Image Text:1) Create a class called Course. It will not have a main method; it is a business class.
2) Put in your documentation comments at the top. Be sure to include your name.
3) Course must have the following instance variables named as shown in the table:
Variable name
crn
subject
number
sectionld
creditHours
instructor
isAvailable
Description
A unique number given each semester to a section (stands for
Course Registration Number) - int
A 4-letter abbreviation for the course (e.g., ITEC, PHED, CHEM)
A 4-digit number associated with the course (e.g., 1001, 0099) - int
A 2-digit number associated with a particular section of a course (e.g.,
01, 13, 47) -int
Number of credit hours the course is worth. Valid values are 0, 1, 2, 3, or
4. The default value is 3. -int
First and last name of the instructor teaching the course. If the instructor
is not known, this should be listed as "TBA" - String
Value is either true or false based upon whether the course is available
for registration. Default is false. - boolean
4) Create a constructor that has the crn, subject, number, and sectionld as String. Initialize the other instance
variables based upon the default values in the table above. Call the appropriate setters from # 7 to validate data.
5) Add a 7-argument constructor that uses all parameter variables as Strings to set the instance variables. Make sure
your constructor calls the setters from #7, to ensure all data is validated. (All arguments will be of type String.)
6) Generate all the getters and setters
7) Changes to make to generated getters and setters to make sure data is valid:
•
public void setCrn(String input) - if input consists of all digits, convert input to integer. Use this integer
to set the CRN. Otherwise, set the CRN to 9001 and display an error message that "Invalid value typed
for CRN. Defaulting to 9001."
• public void setNumber(String input) -if input consists of all digits and is in the range of 1- 9999,
convert input to integer. Use this integer to set the course number. Otherwise, set the course number
to 1001 and display an error message that "Invalid value typed for course number. Defaulting to 1001."
• public void setSectionId(String input) - if input consists of all digits and is in the range of 1 - 80, convert
input to integer. Use this integer to set the section Id. Otherwise, set the section ID to 1 and display an
error message that "Invalid value typed for section ID. Defaulting to 1."
• public void setCreditHours (String input) - if input consists of all digits and is in the range of 0 - 4,
convert input to integer. Use this integer to set the number of credit hours. Otherwise, set the credit
hours to 3 and display an error message that "Invalid value typed for credit hours. Defaulting to 3."
• public void setlsAvailable(String input) - if input starts with 't', 'T', 'y', or 'Y', set Available to true.
Otherwise, set isAvailable to false.
8) Create the toString method
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 8 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
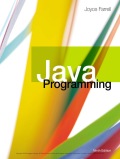
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
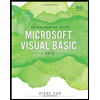
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
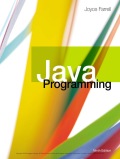
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
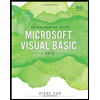
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning