Learning Objectives • Open a file for writing Process information in a dictionary • Practice writing code needed for the final project Instructions A dictionary is a great way to store information in the real world and it is time for us to try it out on files! If you will continue to learn python or programming you will learn about the JSON encryption and "json" module in python, but for now, let's save the dictionary in CSV type that we already learned. If the format of the dictionary looks like this: { id: [name, prct, grades_list] } e.g. { 101: ["quiz", 12, [1,2,3,4]], 102: ["final", 53, [10, 10, 10, 10]] } The file contents should look like 101,quiz,12,1,2,3,4 102,final,53,10,10,10,10 Notice that the list became "unwrapped". It is ok since we know that the 3 first elements in line are if, name, and percentage (prct), and all the rest is the grades_list. 1. Create a function save_dict_to_csv(storage, filename) that will accept the dictionary and the string with future filename 1.0. Use with to open the file with the required filename. Please, use open exactly like this: with open(filename, newline=''). Please notice newline parameter here - it will prevent csv library to write extra empty lines in the file. Check the example in your IDE with and without newline="" and find the difference. 1.1. Use a loop to loop over all keys (and values if you need them) in the dictionary 1.2. Using knowledge about the structure of the dictionary prepare the line to be saved (key, first elem, second elem, third elem)
I have the following code but it is not running correctly!
import csv
lst = []
def save_dict_to_csv(storage, file_name):
with open(file_name, 'w', newline = '') as f:
f.write(file_name)
f.write('\n')
for key, value in storage.items():
lst1 = []
lst1.append(str(key))
for val in value :
if type(val) == list:
for v in val:
lst1.append(str(v))
else:
lst1.append(str(val))
for i in range(len(lst1)):
if i != (len(lst1) - 1):
f.writelines(lst1[i] + ',')
else:
f.writelines(lst1[i])
f.writelines('\n')
f = open(file_name)
print(f.read())
if __name__ == "__main__":
storage = { 101: ["quiz", 12, [1,2,3,4]],
102: ["final", 53, [10, 10, 10, 10]] }
res = save_dict_to_csv(storage, "storage_save.txt")
print(res)
![The file contents should look like
101,quiz,12,1,2,3,4
102,final,53,10,10,10, 10
Notice that the list became "unwrapped". It is ok since we know that the 3 first elements in line are if, name, and percentage (prct), and all
the rest is the grades_list.
1. Create a function save_dict_to_csv(storage, filename) that will accept the dictionary and the string with future filename
1.0. Use with to open the file with the required filename. Please, use open exactly like this: with open(filename, 'w',
newline=''). Please notice newline parameter here - it will prevent csv library to write extra empty lines in the file. Check the
example in your IDE with and without newline="" and find the difference.
1.1. Use a loop to loop over all keys (and values if you need them) in the dictionary
1.2. Using knowledge about the structure of the dictionary prepare the line to be saved (key, first elem, second elem, third elem)
1.3. Use csv module and writer in it to save all lines.
2. You do not have to have any if
main
statement, but you can use it and IDE on your computer to test your
name
program.
Examples of function work:
storage =
{ 101: ["quiz", 12, [1, 2,3,4]],
102: ["final", 53, [10, 10, 10, 10]] }
save_dict_to_csv(storage, "storage_save.txt")
storage save.txt
101,"quiz", 12,1,2,3,4
102,"final",53,10, 10, 10, 10](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa6da4736-f319-4fb1-b2df-b1ab9e1d0ca8%2F972f3d58-81b4-488c-a46e-bb8b350eb2b5%2Fm7hnqr2_processed.png&w=3840&q=75)
![Learning Objectives
Open a file for writing
Process information in a dictionary
Practice writing code needed for the final project
Instructions
A dictionary is a great way to store information in the real world and it is time for us to try it out on files! If you will continue to learn python
or programming you will learn about the JSON encryption and "json" module in python, but for now, let's save the dictionary in CSV type that
we already learned.
If the format of the dictionary looks like this: { id: [name, prct, grades_list] }e.g.
{
101: ["quiz", 12, [1,2,3,4]],
102: ["final", 53, [10, 10, 10, 10]]
}
The file contents should look like
101,quiz,12,1,2,3,4
102,final,53,10,10,10, 10
Notice that the list became "unwrapped". It is ok since we know that the 3 first elements in line are if, name, and percentage (prct), and all
the rest is the grades_list.
1. Create a function save_dict_to_csv(storage, filename) that will accept the dictionary and the string with future filename
1.0. Use with to open the file with the required filename. Please, use open exactly like this: with open(filename, 'w',
newline=''). Please notice newline parameter here - it will prevent csv library to write extra empty lines in the file. Check the
example in your IDE with and without newline="" and find the difference.
1.1. Use a loop to loop over all keys (and values if you need them) in the dictionary
1.2. Using knowledge about the structure of the dictionary prepare the line to be saved (key, first elem, second elem, third elem)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa6da4736-f319-4fb1-b2df-b1ab9e1d0ca8%2F972f3d58-81b4-488c-a46e-bb8b350eb2b5%2Fq1316w_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

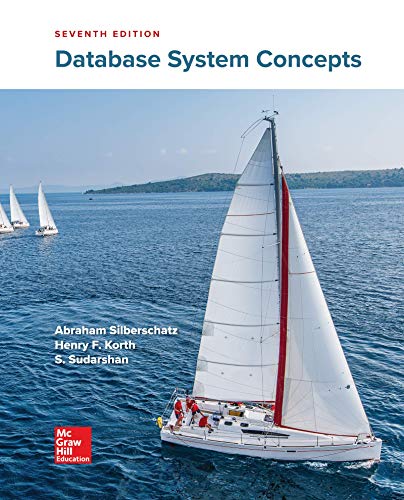
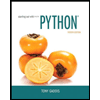
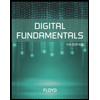
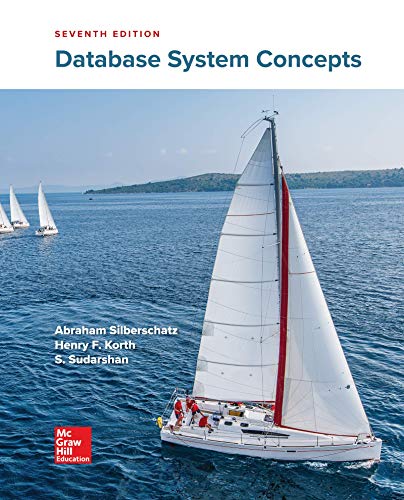
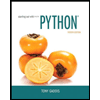
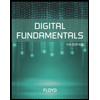
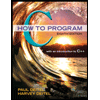
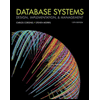
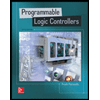