1. The "search" method of the code returns the node that was found or "None" 2. The "Node" implementation has a "key" and "value" member
I am trying to implement a hash table of key-value pairs, where the key determines the location of the pair in the hash table, the key is what the hash function is used on. The hash table that I am trying to implement will use separate chaining (with unordered linked lists) for collision resolution.
I need help changing the code where:
1. The "search" method of the code returns the node that was found or "None"
2. The "Node" implementation has a "key" and "value" member
This is what I have so far
class Node:
def __init__(self,initdata):
self.data = initdata
self.next = None
def getData(self):
return self.data
def getNext(self):
return self.next
def setData(self,newdata):
self.data = newdata
def setNext(self,newnext):
self.next = newnext
class UnorderedList:
def __init__(self):
self.head = None
def isEmpty(self):
return self.head == None
def add(self,item):
temp = Node(item)
temp.setNext(self.head)
self.head = temp
def size(self):
current = self.head
count = 0
while current != None:
count = count + 1
current = current.getNext()
return count
def search(self,item):
current = self.head
found = False
while current != None and not found:
if current.getData() == item:
found = True
else:
current = current.getNext()
return found
def remove(self,item):
current = self.head
previous = None
found = False
while not found:
if current.getData() == item:
found = True
else:
previous = current
current = current.getNext()
if previous == None:
self.head = current.getNext()
else:
previous.setNext(current.getNext())
def printAll(self):
current = self.head
while current != None:
print(current.getData(), end=' ')
current = current.getNext()
print()
if __name__ == "__main__":
mylist = UnorderedList()
mylist.add(31)
mylist.add(77)
mylist.add(17)
mylist.add(93)
mylist.add(26)
mylist.add(54)
mylist.printAll()
print(mylist.size())
mylist.add(100)
mylist.printAll()
print(mylist.size())
mylist.remove(54)
mylist.printAll()
print(mylist.size())
mylist.remove(93)
mylist.printAll()
print(mylist.size())
mylist.remove(31)
mylist.printAll()
print(mylist.size())
*Subject: Python Programming

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

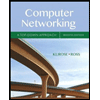
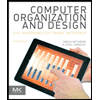
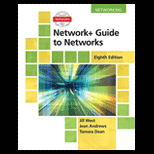
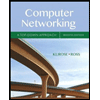
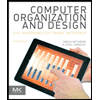
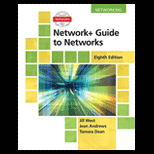
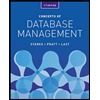
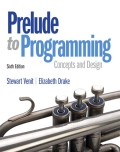
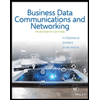