C++ I need to write a recursive function insertEnd that will call a recursive method insertEnd(const ItemType& newEntry, Node* node), to insert newEntry at the end of the linked list I completely stuck and can't figure out where the error is coming from. main #include #include "LinkedList.cpp" int main() {
C++ I need to write a recursive function insertEnd that will call a recursive method insertEnd(const ItemType& newEntry, Node* node), to insert newEntry at the end of the linked list I completely stuck and can't figure out where the error is coming from. main #include #include "LinkedList.cpp" int main() {
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter17: Linked Lists
Section: Chapter Questions
Problem 18SA
Related questions
Question
In C++ I need to write a recursive function insertEnd that will call a recursive method insertEnd(const ItemType& newEntry, Node<ItemType>* node), to insert newEntry at the end of the linked list
I completely stuck and can't figure out where the error is coming from.
main
#include <iostream>
#include "LinkedList.cpp"
int main() {
Node<int>* first = new Node(1);
Node<int>* second = new Node(2);
Node<int>* third = new Node(3);
first->next = second;
second->next = third;
cout<<first->data<<endl;
cout<<first->next->data<<endl;
cout<<first->next->next->data<<endl;
LinkedList<int> list; //an empty linked list
for(int i =0; i<10; i++){
list.inserEnd(i);
list.display();
}
return 0;
}
linkedlist.cpp
#include "LinkedList.h"
#include "iostream"
using namespace std;
template <class T>
LinkedList<T>::LinkedList(){
head = nullptr;
}
template <class T>
void LinkedList<T>::insertEnd(const T& newEntry) {
Node<T>* newNode = new Node(newEntry);
if(head == nullptr) {
return newNode(newEntry);
}else
head->next = insertEnd(head->next, newEntry);
return head;
}
template <class T>
void LinkedList<T>::display(){
Node<T>* temp = head;
while(temp!=nullptr){
cout<<temp->data<<"->"; //display the data of node that temp points to
temp = temp->next; // advance the temp pointer to next node
}
cout<<endl;
}
LinkedList.h
#ifndef LIST_H
#define LIST_H
#include "node.h"
template <class T>
class LinkedList {
private:
Node<T>* head;
// int newEntry;
public:
LinkedList();
void insertEnd(const T& newEntry);
void display();
};
#endif
Node.h
#ifndef NODE_H
#define NODE_H
template <class T>
class Node{
public:
T data;
Node<T>* next;
Node(T d){
data = d;
next = nullptr;
}
};
#endif
![> clang++-7 -pthread -std=C++17 -o main LinkedList.cpp main.cpp
In file included from main.cpp:2:
./LinkedList.cpp:17:11: error: called object type 'Node<int> *' is not a
function or function pointer
return newNode(newEntry);
main.cpp:22:8: note: in instantiation of member function
'LinkedList<int>::insertEnd' requested here
list.insertEnd(i);
In file included from main.cpp:2:
./LinkedList.cpp:20:3: error: void function 'insertEnd' should not return a
value [-Wreturn-type]
return head;
2 errors generated.
compiler exit status 1](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fbc17783e-7d96-40dd-a47a-5f95fbd7868b%2Ff735cbd6-a31f-44dd-8c2d-49599930460a%2Fv20lzcn_processed.png&w=3840&q=75)
Transcribed Image Text:> clang++-7 -pthread -std=C++17 -o main LinkedList.cpp main.cpp
In file included from main.cpp:2:
./LinkedList.cpp:17:11: error: called object type 'Node<int> *' is not a
function or function pointer
return newNode(newEntry);
main.cpp:22:8: note: in instantiation of member function
'LinkedList<int>::insertEnd' requested here
list.insertEnd(i);
In file included from main.cpp:2:
./LinkedList.cpp:20:3: error: void function 'insertEnd' should not return a
value [-Wreturn-type]
return head;
2 errors generated.
compiler exit status 1
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
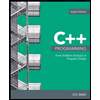
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
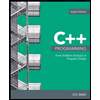
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning