y: Queue Linked List Explain the flow of the code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow. SEE ATTACHED PHOTO FOR THE PROBLEM #include "queue.h" #include "linkedlist.h"
y: Queue Linked List Explain the flow of the code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow. SEE ATTACHED PHOTO FOR THE PROBLEM #include "queue.h" #include "linkedlist.h"
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 16PE:
The implementation of a queue in an array, as given in this chapter, uses the variable count to...
Related questions
Question
C++ Programming
Activity: Queue Linked List
Activity: Queue Linked List
Explain the flow of the code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow.
SEE ATTACHED PHOTO FOR THE PROBLEM
#include "queue.h"
#include "linkedlist.h"
class SLLQueue : public Queue {
LinkedList* list;
public:
SLLQueue() {
list = new LinkedList();
}
void enqueue(int e) {
list->addTail(e);
return;
}
int dequeue() {
int elem;
elem = list->removeHead();
return elem;
}
int first() {
int elem;
elem = list->get(1);
return elem;;
}
int size() {
return list->size();
}
bool isEmpty() {
return list->isEmpty();
}
int collect(int max) {
int sum = 0;
while(first() != 0) {
if(sum + first() <= max) {
sum += first();
dequeue();
} else {
break;
}
}
return sum;
}
};
![We have discussed how to implement our Queue using ArrayList in
our previous discussion. Now it is your turn to implement our Queue
using a Singly Linked List (SLL), but this time, store an integer int
instead of a character char.
Additionally, you are also going to implement a method in our Queue
implementation which will be: int collect(int max)
The method will continuously sum up and remove elements in our
queue and will only stop before the sum exceeds the value of max or
when the queue is exhausted. After that, we return the sum. If the
first value is already larger than the given max or when the queue is
empty, return 0.
In a given Queue with the elements (10, 80, 40, 5] where 10 is the
first and upon calling collect(100), it will sum all the values of the
queue starting from 10 and will remove it. It will only stop with 80 as
the current sum is 10+80 = 90 and if we try to add 40, it will exceed
the given max which is 100. Therefore, we return the value 90 and
the queue shall only contain the values [40, 5).
Restrictions for collect: You cannot perform enqueue nor any other
method calls from your list. You can only use the methods in your
Queue except for enqueue.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6a8dd343-05d5-4c47-959b-e09f7e32f213%2F0fdf1f82-d0f9-4ab9-ae50-e180dabb016a%2Fecxiukd_processed.png&w=3840&q=75)
Transcribed Image Text:We have discussed how to implement our Queue using ArrayList in
our previous discussion. Now it is your turn to implement our Queue
using a Singly Linked List (SLL), but this time, store an integer int
instead of a character char.
Additionally, you are also going to implement a method in our Queue
implementation which will be: int collect(int max)
The method will continuously sum up and remove elements in our
queue and will only stop before the sum exceeds the value of max or
when the queue is exhausted. After that, we return the sum. If the
first value is already larger than the given max or when the queue is
empty, return 0.
In a given Queue with the elements (10, 80, 40, 5] where 10 is the
first and upon calling collect(100), it will sum all the values of the
queue starting from 10 and will remove it. It will only stop with 80 as
the current sum is 10+80 = 90 and if we try to add 40, it will exceed
the given max which is 100. Therefore, we return the value 90 and
the queue shall only contain the values [40, 5).
Restrictions for collect: You cannot perform enqueue nor any other
method calls from your list. You can only use the methods in your
Queue except for enqueue.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
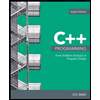
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
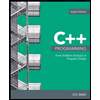
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning