1.12 Lab: Check Login Credentials (classes) Complete the Login class implementation. For the class method check credentials(), you will need to accept two arguments - login and passwd. Do not forget about the self argument. This class method will set two local variables simlogin = "Test' and simpass = "test1234". These variables will simulate the correct login and password for the credential check process. The method will receive the login and password from the user and check it with Test and test1234. If the user sent the correct credentials output: Successful login! If the user sent the correct login name but the wrong password output: Login name is correct, incorrect password! If the user sent an incorrect login name but the correct password output: Login name is incorrect, password accepted! If the user sent both incorrect login name and password output: Unsuccessful login attempt! The class method will return a boolean success variable as True if the login was successful. " Using if _name_ == "main_ to control the flow of your code, add a loop that will stop the process if the user attempts more than 5 login attempts. Ex: If the input is: Testit test And this is their first incorrect attempt. The output is: Unsuccessful login attempt! Try again - you have 4 attempts left! If the input is: Test test1234 The output is: Successful login!
1.12 Lab: Check Login Credentials (classes) Complete the Login class implementation. For the class method check credentials(), you will need to accept two arguments - login and passwd. Do not forget about the self argument. This class method will set two local variables simlogin = "Test' and simpass = "test1234". These variables will simulate the correct login and password for the credential check process. The method will receive the login and password from the user and check it with Test and test1234. If the user sent the correct credentials output: Successful login! If the user sent the correct login name but the wrong password output: Login name is correct, incorrect password! If the user sent an incorrect login name but the correct password output: Login name is incorrect, password accepted! If the user sent both incorrect login name and password output: Unsuccessful login attempt! The class method will return a boolean success variable as True if the login was successful. " Using if _name_ == "main_ to control the flow of your code, add a loop that will stop the process if the user attempts more than 5 login attempts. Ex: If the input is: Testit test And this is their first incorrect attempt. The output is: Unsuccessful login attempt! Try again - you have 4 attempts left! If the input is: Test test1234 The output is: Successful login!
Chapter14: Security, Troubleshooting, And Performance
Section: Chapter Questions
Problem 7HOP
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
Please revise this code so that the outputs are correct.
Current it’s flagging a syntax error. See screen shot. Also view the lab ( 2nd ) screen shot.
Please use both inputs to produce both out outputs.
1st input:
Testit
test
1st output:
Unsuccessful login attempt!
Try again – you have four times left!
2nd input:
Test
Test1234
Second output:
Successful login!
Current code needs amended:
 #class Login
class Login:
#__init__
def __init__(self):
#initializing login_name as none
self.login_name = 'none'
#initializing login_password as none
self.login_password = 'none'
#check_credentials()
def check_credentials(self, user_login, user_passwd):
#initializing simlogin as 'Test'
simlogin = 'Test'
#initializing simpass as 'Test'
simpass = 'test1234'
#if user sent the correct credentials
if user_login == simlogin and user_passwd == simpass:
#print "Successful login!"
print("Successful login!")
#returns True
return True
#if correct login name and incorrect password
elif user_login == simlogin and user_passwd != simpass:
#print "Login name is correct, incorrect password!"
print("Login name is correct, incorrect password!")
#returns False
return False
#if incorrect login name and correct password
elif user_login != simlogin and user_passwd == simpass:
#print "Login name incorrect, password accepted!"
print("Login name incorrect, password accepted!")
#returns False
return False
#if incorrect login name and incorrect password
elif user_login != simlogin and user_passwd != simpass:
#print "Unsuccessful login attempt!"
print("Unsuccessful login attempt!")
print("try again – you have four attempts left!")
#returns False
return False #main
if __name__ == "__main__":
#object of Login class
ob = Login()
#timeout variable used for login attempts
timeout = 5
#prompt for user name
login = input()
#prompt for password
password = input()
#calling check_credentials to check credentials are valid or not
valid_login = ob.check_credentials(login, password)
#loop
while True:
#if valid login
if valid_login:
#exits the loop
break
#if not valid login
else:
#decrements timeout
timeout = timeout - 1
#if timeout equals 0
if timeout == 0:
#prints "5 failed login attempts. No more login attempts."
print("5 failed login attempts. No more login attempts.")
#exits the loop
break
#prompt for user name
login = input()
#prompt for password
password = input()
#calling check_credentials to check credentials are valid or not
valid_login = ob.check_credentials(login, password)
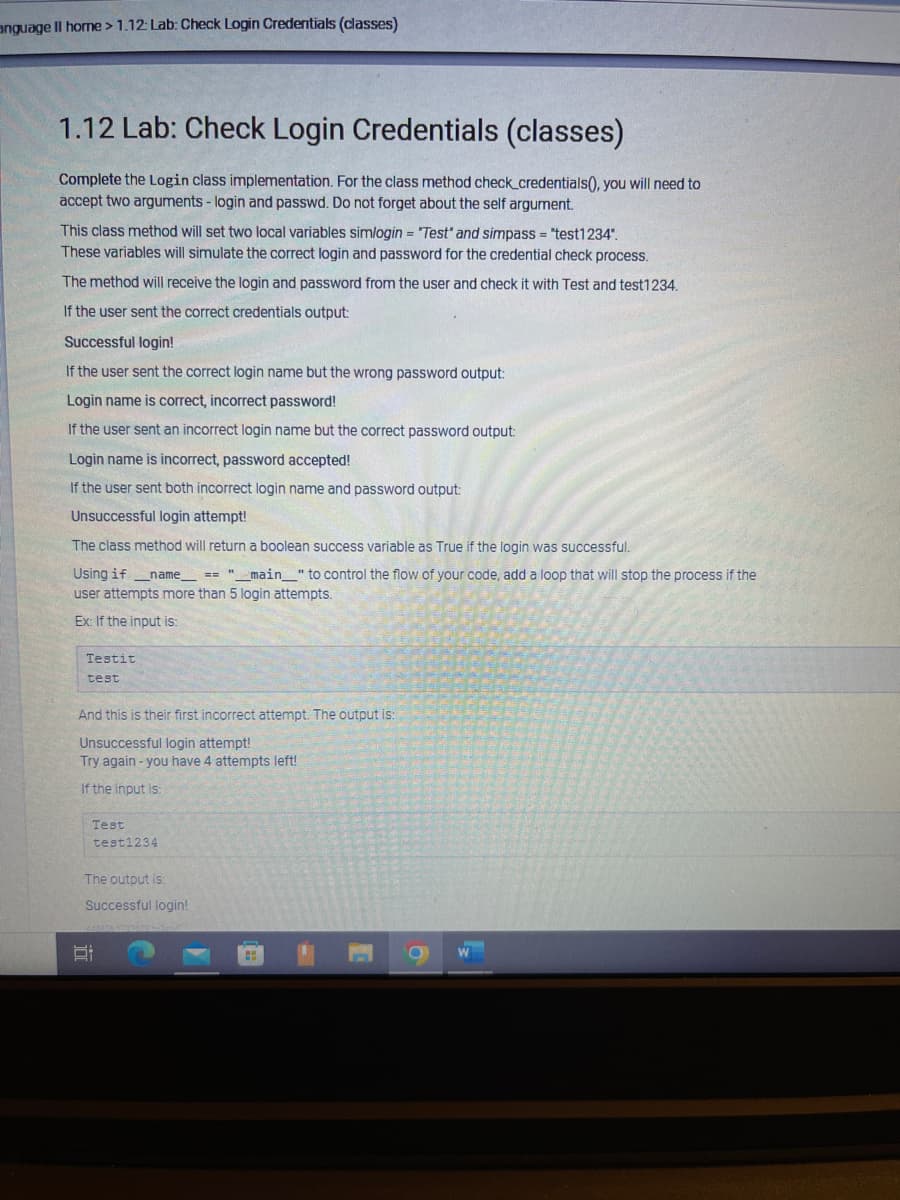
Transcribed Image Text:anguage Il home > 1.12: Lab: Check Login Credentials (classes)
1.12 Lab: Check Login Credentials (classes)
Complete the Login class implementation. For the class method check_credentials(), you will need to
accept two arguments - login and passwd. Do not forget about the self argument.
This class method will set two local variables simlogin = "Test' and simpass = "test1234".
These variables will simulate the correct login and password for the credential check process.
The method will receive the login and password from the user and check it with Test and test1234.
If the user sent the correct credentials output:
Successful login!
If the user sent the correct login name but the wrong password output:
Login name is correct, incorrect password!
If the user sent an incorrect login name but the correct password output:
Login name is incorrect, password accepted!
If the user sent both incorrect login name and password output:
Unsuccessful login attempt!
The class method will return a boolean success variable as True if the login was successful.
Using if _name__ == "__main_" to control the flow of your code, add a loop that will stop the process if the
user attempts more than 5 login attempts.
Ex: If the input is:
Testit
test
And this is their first incorrect attempt. The output is:
Unsuccessful login attempt!
Try again - you have 4 attempts left!
If the input is:
Test
test1234
The output is.
Successful login!
D
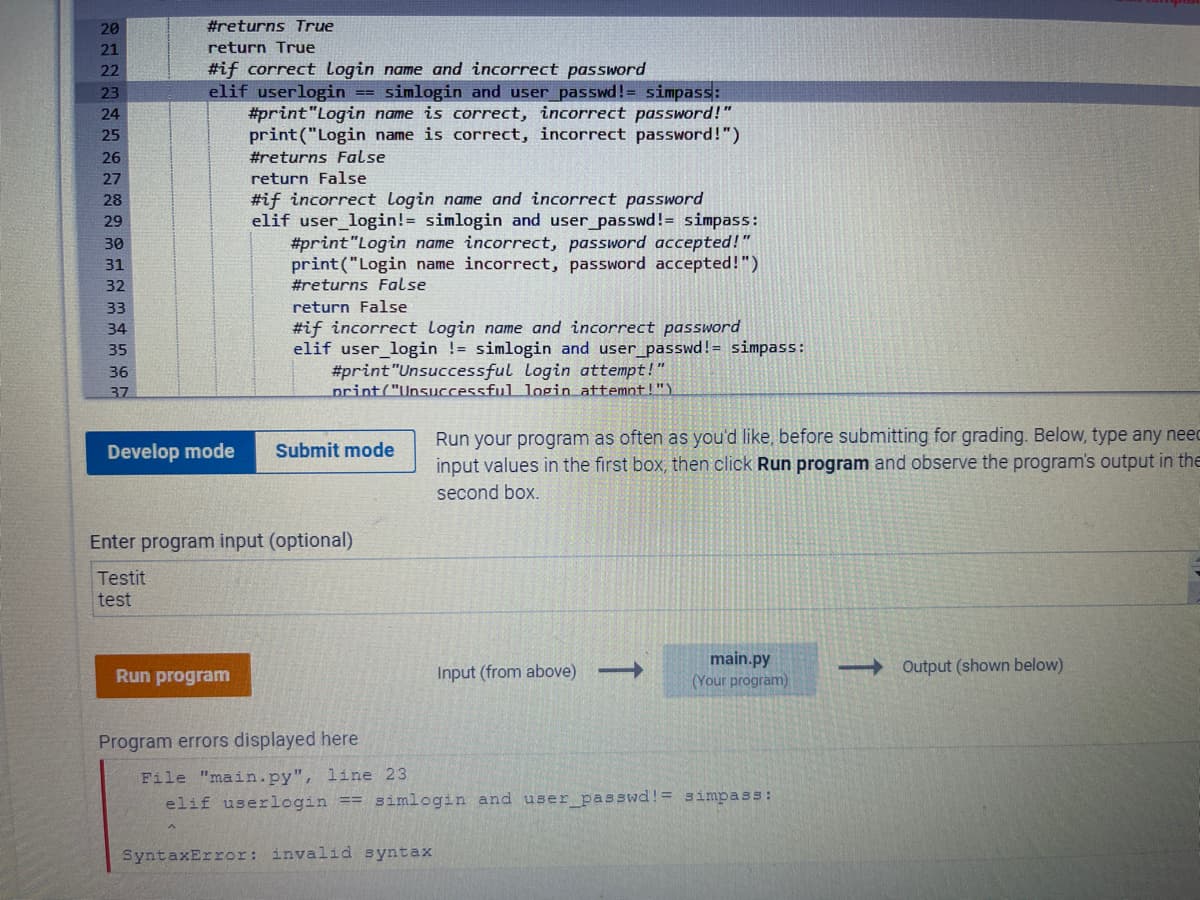
Transcribed Image Text:20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
#returns True
return True
#if correct Login name and incorrect password
elif userlogin == simlogin and user_passwd != simpass:
#print "Login name is correct, incorrect password!"
print("Login name is correct, incorrect password!")
#returns False
Develop mode
return False
#if incorrect Login name and incorrect password
elif user_login!= simlogin and user_passwd!= simpass:
#print "Login name incorrect, password accepted!"
print("Login name incorrect, password accepted!")
#returns False
Run program
return False
#if incorrect Login name and incorrect password
elif user_login != simlogin and user_passwd!= simpass:
#print "Unsuccessful Login attempt!"
print("Unsuccessful login attempt!")
Submit mode
Enter program input (optional)
Testit
test
Program errors displayed here
Run your program as often as you'd like, before submitting for grading. Below, type any neec
input values in the first box, then click Run program observe the progra
second box.
output in the
SyntaxError: invalid syntax
Input (from above) →→→→→
main.py
(Your program)
File "main.py", line 23
elif userlogin == simlogin and user_passwd!= simpass:
-Output (shown below)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
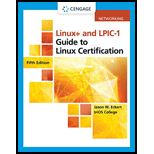
LINUX+ AND LPIC-1 GDE.TO LINUX CERTIF.
Computer Science
ISBN:
9781337569798
Author:
ECKERT
Publisher:
CENGAGE L
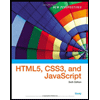
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:
9781305503922
Author:
Patrick M. Carey
Publisher:
Cengage Learning
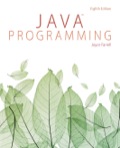
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
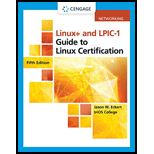
LINUX+ AND LPIC-1 GDE.TO LINUX CERTIF.
Computer Science
ISBN:
9781337569798
Author:
ECKERT
Publisher:
CENGAGE L
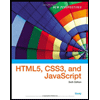
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:
9781305503922
Author:
Patrick M. Carey
Publisher:
Cengage Learning
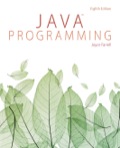
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT