Analyze the code below. Provide a case when the program will display "Unfortunately, you do not have enough balance to withdraw the amount." Specifically: 1. Provide values for accountNum and amount that will cause the program to display the error when the values are passed as arguments to the withdraw function. (You only need to provide one set of values). 2. Provide a step-by-step explanation of how the message gets displayed. Describe what functions are called, what lines of code are executed, or what are the results of an operation. 01 enum BankErrors : Error { 02 case InvalidAccount, InsufficientBalance 03 } 04 05 struct Bank { 06 var accounts: [Int : Double] = [:] 07 08 mutating func create(account: Int, amount: Double) { 09 accounts[account] = amount 10 } 11 12 mutating func withdraw(_ amount: Double, from: Int) throws { 13 guard let balance = accounts[from] else { 14 throw BankErrors.InvalidAccount 15 } 16 guard amount <= balance else { 17 throw BankErrors.InsufficientBalance 18 } 19 20 accounts[from] = balance - amount 21 } 22 } 23 24 var myBank = Bank(accounts:[1121: 500.0, 33412: 90.0, 77121: 1200.25]) 25 26 var accountNum = 1 27 var amount = 2.0 28 29 do { 30 try myBank.withdraw(amount, from: accountNum) 31 } catch (BankErrors.InvalidAccount) { 32 print("Account does not exist. Please open an account first.") 33 } catch (BankErrors.InsufficientBalance) { 34 print("Unfortunately, you do not have enough balance to withdraw the amount.") 35 } 36 print("Transaction complete.")
7.
Analyze the code below. Provide a case when the program will display "Unfortunately, you do not have enough balance to withdraw the amount." Specifically:
1. Provide values for accountNum and amount that will cause the program to display the error when the values are passed as arguments to the withdraw function. (You only need to provide one set of values).
2. Provide a step-by-step explanation of how the message gets displayed. Describe what functions are called, what lines of code are executed, or what are the results of an operation.
01 enum BankErrors : Error {
02 case InvalidAccount, InsufficientBalance
03 }
04
05 struct Bank {
06 var accounts: [Int : Double] = [:]
07
08 mutating func create(account: Int, amount: Double) {
09 accounts[account] = amount
10 }
11
12 mutating func withdraw(_ amount: Double, from: Int) throws {
13 guard let balance = accounts[from] else {
14 throw BankErrors.InvalidAccount
15 }
16 guard amount <= balance else {
17 throw BankErrors.InsufficientBalance
18 }
19
20 accounts[from] = balance - amount
21 }
22 }
23
24 var myBank = Bank(accounts:[1121: 500.0, 33412: 90.0, 77121: 1200.25])
25
26 var accountNum = 1
27 var amount = 2.0
28
29 do {
30 try myBank.withdraw(amount, from: accountNum)
31 } catch (BankErrors.InvalidAccount) {
32 print("Account does not exist. Please open an account first.")
33 } catch (BankErrors.InsufficientBalance) {
34 print("Unfortunately, you do not have enough balance to withdraw the amount.")
35 }
36 print("Transaction complete.")

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

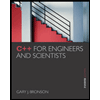
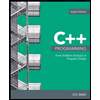
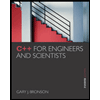
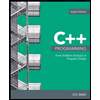