Hello. I'm creating a game of rock, paper, scissors in my python code. I'm confused about how to make the computer get a random number. Instructions: You may not use any global variables A limited number of global constants are permitted (Hint: use for ROCK, PAPER, and SCISSORS) Where applicable, values must be passed as arguments to functions Where applicable, functions must return values As a minimum, you must have the following functions: main – calls the introduction function to get the player’s name and print the game instructions. The game should be played in a loop, until the user inputs a sentinel value to end play. Inside the loop the following must happen: get the user and computer plays determine the winner keep track of wins and losses Call the print_statistics function at the end of the game (after exiting the loop) introduction – prints welcome message, gets the player’s name, prints game instru
Hello. I'm creating a game of rock, paper, scissors in my python code. I'm confused about how to make the computer get a random number. Instructions: You may not use any global variables A limited number of global constants are permitted (Hint: use for ROCK, PAPER, and SCISSORS) Where applicable, values must be passed as arguments to functions Where applicable, functions must return values As a minimum, you must have the following functions: main – calls the introduction function to get the player’s name and print the game instructions. The game should be played in a loop, until the user inputs a sentinel value to end play. Inside the loop the following must happen: get the user and computer plays determine the winner keep track of wins and losses Call the print_statistics function at the end of the game (after exiting the loop) introduction – prints welcome message, gets the player’s name, prints game instru
Chapter9: Advanced Array Concepts
Section: Chapter Questions
Problem 2GZ
Related questions
Question
Hello. I'm creating a game of rock, paper, scissors in my python code. I'm confused about how to make the computer get a random number.
Instructions:
- You may not use any global variables
- A limited number of global constants are permitted (Hint: use for ROCK, PAPER, and SCISSORS)
- Where applicable, values must be passed as arguments to functions
- Where applicable, functions must return values
- As a minimum, you must have the following functions:
- main – calls the introduction function to get the player’s name and print the game instructions. The game should be played in a loop, until the user inputs a sentinel value to end play. Inside the loop the following must happen:
- get the user and computer plays
- determine the winner
- keep track of wins and losses
- Call the print_statistics function at the end of the game (after exiting the loop)
- introduction – prints welcome message, gets the player’s name, prints game instructions, returns the player’s name
- get_user_play – gets the user’s play selection from the keyboard, and returns it
- get_computer_play – Uses a random number to get the computer’s chosen play selection, and returns it
- print_statistics – Outputs the number of games played, and the total won by the player and the computer, as well as the number of ties. Requires data be passed in as parameters.
- main – calls the introduction function to get the player’s name and print the game instructions. The game should be played in a loop, until the user inputs a sentinel value to end play. Inside the loop the following must happen:
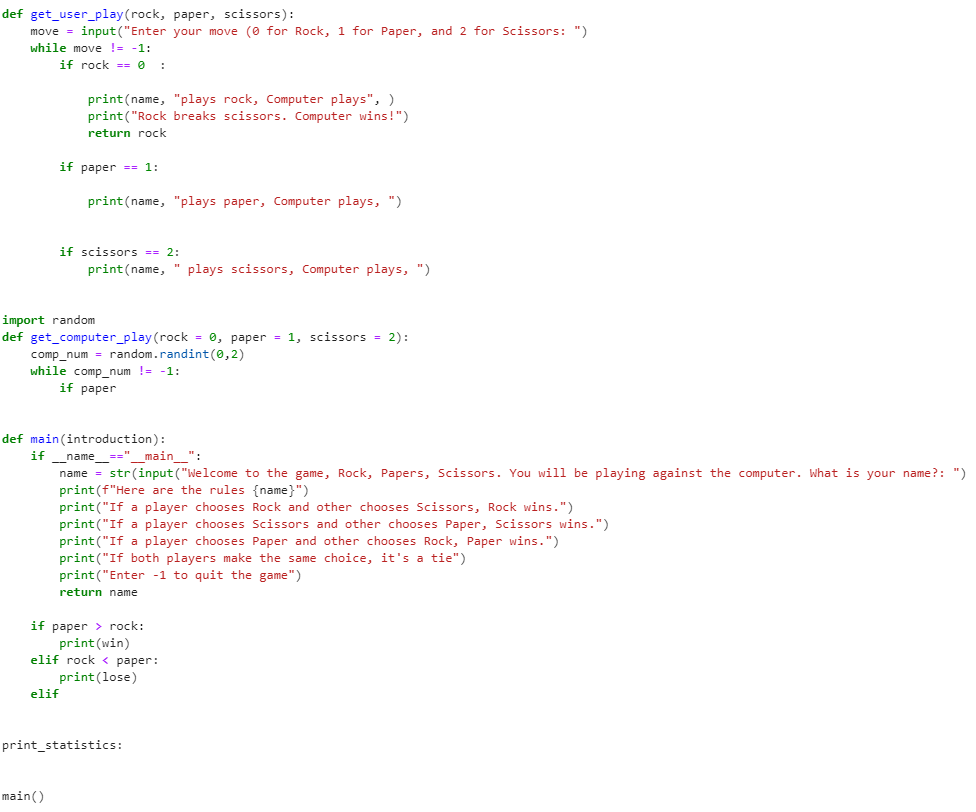
Transcribed Image Text:def get_user_play (rock, paper, scissors):
move = input ("Enter your move (0 for Rock, 1 for Paper, and 2 for Scissors: ")
while move != -1:
if rock == 0 :
print (name, "plays rock, Computer plays", )
print("Rock breaks scissors. Computer wins!")
return rock
if paper == 1:
print (name, "plays paper, Computer plays, ")
if scissors == 2:
print(name, "plays scissors, Computer plays, ")
import random
def get_computer_play (rock = 0, paper = 1, scissors = 2):
comp_num= random.randint(0,2)
while comp_num != -1:
if paper
def main(introduction):
if __name__ == "____main__":
name = str(input ("Welcome to the game, Rock, Papers, Scissors. You will be playing against the computer. What is your name?: ")
print (f"Here are the rules {name}")
main ()
print("If a player chooses Rock and other chooses Scissors, Rock wins.")
print("If a player chooses Scissors and other chooses Paper, Scissors wins.")
print("If a player chooses Paper and other chooses Rock, Paper wins.")
print("If both players make the same choice, it's a tie")
print("Enter -1 to quit the game")
return name
if paper > rock:
print (win)
elif rock paper:
print (lose)
elif
print_statistics:
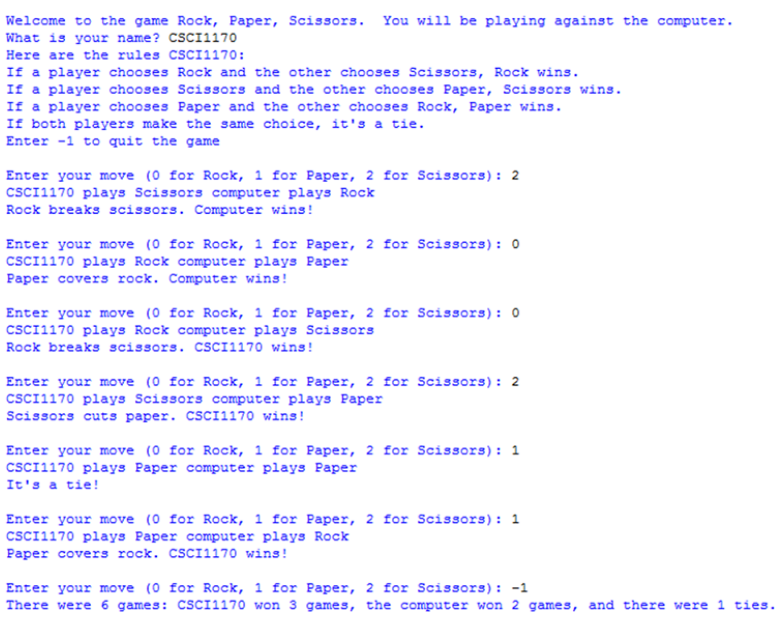
Transcribed Image Text:Welcome to the game Rock, Paper, Scissors. You will be playing against the computer.
What is your name? CSCI1170
Here are the rules CSCI1170:
If a player chooses Rock and the other chooses Scissors, Rock wins.
If a player chooses Scissors and the other chooses Paper, Scissors wins.
If a player chooses Paper and the other chooses Rock, Paper wins.
If both players make the same choice, it's a tie.
Enter 1 to quit the game
Enter your move (0 for Rock, 1 for Paper, 2 for Scissors): 2
CSCI1170 plays Scissors computer plays Rock
Rock breaks scissors. Computer wins!
Enter your move (0 for Rock, 1 for Paper, 2 for Scissors): 0
CSCI1170 plays Rock computer plays Paper
Paper covers rock. Computer wins!
Enter your move (0 for Rock, 1 for Paper, 2 for Scissors): 0
CSCI1170 plays Rock computer plays Scissors
Rock breaks scissors. CSCI1170 wins!
Enter your move (0 for Rock, 1 for Paper, 2 for Scissors): 2
CSCI1170 plays Scissors computer plays Paper
Scissors cuts paper. CSCI1170 wins!
Enter your move (0 for Rock, 1 for Paper, 2 for Scissors): 1
CSCI1170 plays Paper computer plays Paper
It's a tie!
Enter your move (0 for Rock, 1 for Paper, 2 for Scissors): 1
CSCI1170 plays Paper computer plays Rock
Paper covers rock. CSCI1170 wins!
Enter your move (0 for Rock, 1 for Paper, 2 for Scissors): -1
There were 6 games: CSCI1170 won 3 games, the computer won 2 games, and there were 1 ties.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 10 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
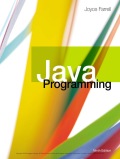
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
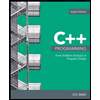
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
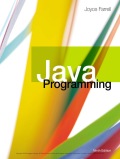
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
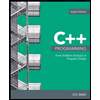
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning