2. Define a new "findDegreesByYearAndSubject" function that accepts 4 parameters: o An array of "Degree" object pointers (not objects) o Array's length o Search year o Search subject It will return a vector of Degree object pointers containing only pointers to the matched Degree objects in the given array. It will return an empty vector if there is no match found. Show how this function is being called and returning proper values.
PLease! In c++
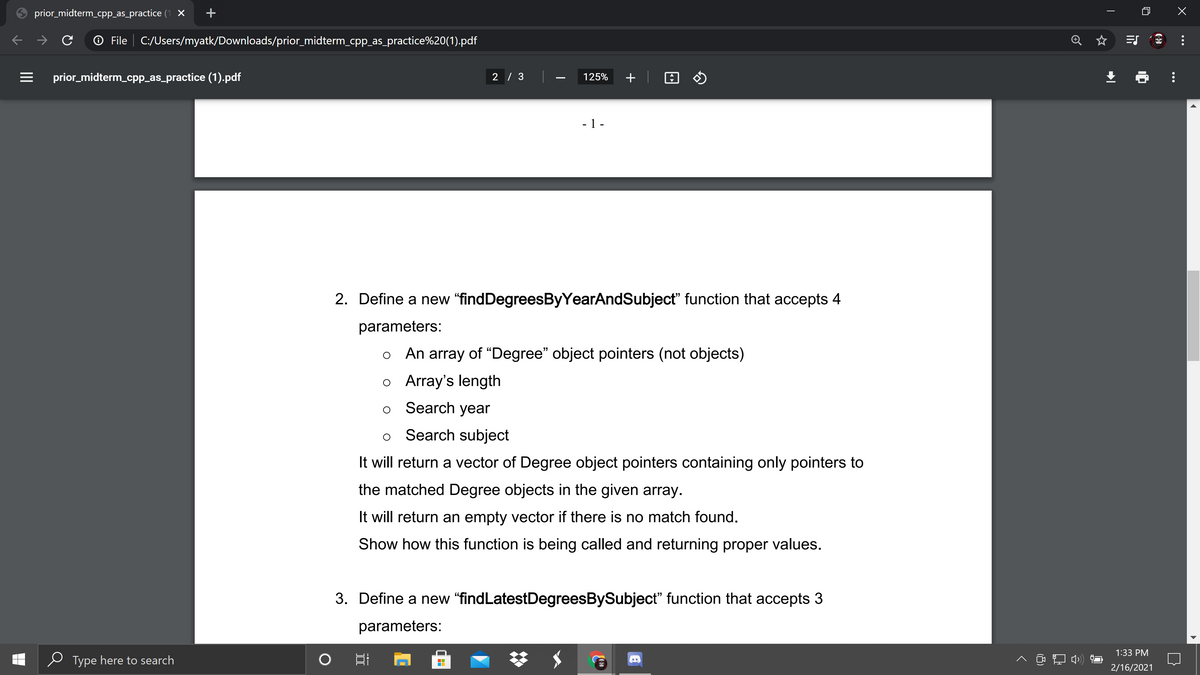

#include <iostream>
#include <vector>
using namespace std;
class Degree
{
int year;
string subject;
public:
Degree(int y, string s)
{
year = y;
subject = s;
}
int getYear()
{
return year;
}
string getSubject()
{
return subject;
}
void setYear(int y)
{
year = y;
}
void setSubject(string s)
{
subject = s;
}
void print()
{
cout << "\n\nYEAR: " << getYear();
cout << "\tSUBJECT: " << getSubject();
}
};
vector<Degree *> findDegreesByYearAndSubject(Degree *degrees, int length, int year, string subject)
{
vector<Degree *> return_degree;
for (int i = 0; i < length; i++)
{
if (degrees[i].getYear() == year && degrees[i].getSubject() == subject)
{
return_degree.push_back(°rees[i]);
}
}
return return_degree;
}
int main()
{
Degree *degrees;
int length;
cout << "\nEnter the size: ";
cin >> length;
degrees = (Degree *)malloc(length * sizeof(Degree));
int year;
string subject;
int i = 0;
while (i < length)
{
cout << "\nEnter the year: ";
cin >> year;
cout << "Enter the subject: ";
cin >> subject;
degrees[i].setYear(year);
degrees[i].setSubject(subject);
++i;
}
cout << "\nEnter year to be search: ";
cin >> year;
cout << "Enter subject to be search: ";
cin >> subject;
vector<Degree *> result = findDegreesByYearAndSubject(degrees, length, year, subject);
cout<<"\n\nRESULTS:";
for(int i = 0; i < result.size(); i++){
result[i]->print();
}
}
Step by step
Solved in 2 steps

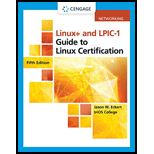
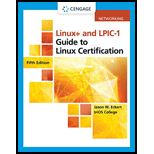