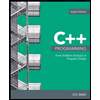
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN: 9781337102087
Author: D. S. Malik
Publisher: Cengage Learning
expand_more
expand_more
format_list_bulleted
Question
Fill out the starter code
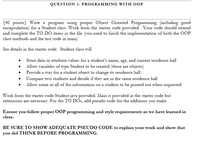
Transcribed Image Text:QUESTION 2: PROGRAMMING WITH OOP
{40 points} Write a program using proper Object Oriented Programming (including good
encapsulation) for a Student class. Work from the starter code provided. Your code should extend
and complete the TO DO items in the file (you need to finish the implementation of both the OOP
class methods and the test code in main).
See details in the starter code. Student class will
Store data or attribute values for a student's name, age, and current residence hall
Allow variables of type Student to be created (these are objects)
Provide a way for a student object to change its residence hall
Compare two students and decide if they are in the same residence hall
Allow some or all of the information on a student to be printed out when requested
Work from the starter code Student.java provided. Main is provided in the starter code but
extensions are necessary. For the TO DOS, add pseudo code for the additions you make.
Ensure you follow proper OOP programming and style requirements as we have learned in
class.
BE SURE TO SHOW ADEQUATE PSEUDO CODE to explain your work and show that
you did THINK BEFORE PROGRAMMING.
![public class Student {
/*
Starter code for your Student class and tests from main
See the TO DO comments for hints on how to proceed
Be sure your code compiles and runs when finished!
*/
private String name;
private int age;
private String residenceHall;
//constructor
public Student (String n, int a, String dorm) {
name = n;
age = a;
residenceHall = dorm;
}
/*
TO DOs to finish the Student class
Using good encapsulation, provide code that will allow you to extend the code in main (see those TO DOs
Consider:
TO DO 1: provide a mutator method that updates a Student's residenceHall
TO DO 2: provide some mechanism (several are possible) so that main can compare residenceHalls for two Students
TO DO 3: ensure main can print out Student's names after checking for matching residences (some kind of accessor method)
*/
//test code in main
public static void main(String[] args){
Student richard;
Student jane;
Student sue;
richard = new Student("Richard Smith",19,"San Francisco Hall");
jane = new Student ("Jane Dold", 21, "Fairfield Hall");
sue = new Student("Sue Alexander", 20, "Georgetown Hall");
/*TO DO 1: check to see if Richard and Sue are in the same residence hall;
if so, print out their names and say they are both in same dorm building; otherwise, do nothing
TO DO 2: change Sue's residence hall to Fairfield Hall
TO DO 3: check to see if Jane and Sue are in the same residence hall;
if so, print out their names and say they are both in same dorm building; otherwise, do nothing
YOUR CODE CANNOT RELY ON KNOWING THE VALUES FOR CURRENT RESIDENT HALL ASSIGNMENTS
USE PROPER OOP TO GET THESE VALUES
*/
}](https://content.bartleby.com/qna-images/question/bda60386-cf75-4b0b-a556-f8e365521ce5/be01bc3e-7188-4f7c-b324-bcb0b5f618e6/xwql99_thumbnail.png)
Transcribed Image Text:public class Student {
/*
Starter code for your Student class and tests from main
See the TO DO comments for hints on how to proceed
Be sure your code compiles and runs when finished!
*/
private String name;
private int age;
private String residenceHall;
//constructor
public Student (String n, int a, String dorm) {
name = n;
age = a;
residenceHall = dorm;
}
/*
TO DOs to finish the Student class
Using good encapsulation, provide code that will allow you to extend the code in main (see those TO DOs
Consider:
TO DO 1: provide a mutator method that updates a Student's residenceHall
TO DO 2: provide some mechanism (several are possible) so that main can compare residenceHalls for two Students
TO DO 3: ensure main can print out Student's names after checking for matching residences (some kind of accessor method)
*/
//test code in main
public static void main(String[] args){
Student richard;
Student jane;
Student sue;
richard = new Student("Richard Smith",19,"San Francisco Hall");
jane = new Student ("Jane Dold", 21, "Fairfield Hall");
sue = new Student("Sue Alexander", 20, "Georgetown Hall");
/*TO DO 1: check to see if Richard and Sue are in the same residence hall;
if so, print out their names and say they are both in same dorm building; otherwise, do nothing
TO DO 2: change Sue's residence hall to Fairfield Hall
TO DO 3: check to see if Jane and Sue are in the same residence hall;
if so, print out their names and say they are both in same dorm building; otherwise, do nothing
YOUR CODE CANNOT RELY ON KNOWING THE VALUES FOR CURRENT RESIDENT HALL ASSIGNMENTS
USE PROPER OOP TO GET THESE VALUES
*/
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Using classes, design an online address book to keep track of the names, addresses, phone numbers, and dates of birth of family members, close friends, and certain business associates. Your program should be able to handle a maximum of 500 entries. Define a class addressType that can store a street address, city, state, and ZIP code. Use the appropriate functions to print and store the address. Also, use constructors to automatically initialize the member variables. Define a class extPersonType using the class personType (as defined in Example 10-10, Chapter 10), the class dateType (as designed in this chapters Programming Exercise 2), and the class addressType. Add a member variable to this class to classify the person as a family member, friend, or business associate. Also, add a member variable to store the phone number. Add (or override) the functions to print and store the appropriate information. Use constructors to automatically initialize the member variables. Define the class addressBookType using the previously defined classes. An object of the type addressBookType should be able to process a maximum of 500 entries. The program should perform the following operations: Load the data into the address book from a disk. Sort the address book by last name. Search for a person by last name. Print the address, phone number, and date of birth (if it exists) of a given person. Print the names of the people whose birthdays are in a given month. Print the names of all the people between two last names. Depending on the users request, print the names of all family members, friends, or business associates.arrow_forwardIn previous chapters, you have created programs for the Greenville Idol competition. Now create a Contestant class with the following characteristics: The Contestant class contains public static arrays that hold talent codes and descriptions. Recall that the talent categories are Singing Dancing, Musical instrument, and Other. The class contains an auto-implemented property that holds a contestants name. The class contains fields for a talent code and description. The set accessor for the code assigns a code only if it is valid. Otherwise, it assigns I for Invalid. The talent description is a read-only property that is assigned a value when the code is set. Modify the GreenvilleRevenue program so that it uses the Contestant class and performs the following tasks: The program prompts the user for the number of contestants in this years competition; the number must be between 0 and 30. The program continues to prompt the user until a valid value is entered. The expected revenue is calculated and displayed. The revenue is $25 per contestant. The program prompts the user for names and talent codes for each contestant entered. Along with the prompt for a talent code, display a list of the valid categories. After data entry is complete, the program displays the valid talent categories and then continuously prompts the user for talent codes and displays the names of all contestants in the category. Appropriate messages are displayed if the entered code is not a character or a valid code.arrow_forwarda. Write a FractionDemo program that instantiates several Fraction objects and demonstrates that their methods work correctly. Create a Fraction class with fields that hold a whole number, a numerator, and a denominator. In addition: Create properties for each field. The set access or for the denominator should not allow a 0 value; the value defaults to 1. Add three constructors. One takes three parameters for a whole number, numerator, and denominator. Another accepts two parameters for the numerator and denominator; when this constructor is used, the whole number value is 0. The last constructor is parameterless; it sets the whole number and numerator to 0 and the denominator to 1. (After construction, Fractions do not have to be reduced to proper form. For example, even though 3/9 could be reduced to 1/3, your constructors do not have to perform this task.) Add a Reduce() method that reduces a Fraction if it is in improper form. For example, 2/4 should be reduced to 1/2. Add an operator+() method that adds two Fractions. To add two fractions, first eliminate any whole number part of the value. For example, 2 1/4 becomes 9/4 and 1 3/5 becomes 8/5. Find a common denominator and convert the fractions to it. For example, when adding 9/4 and 8/5, you can convert them to 45/20 and 32/20. Then you can add the numerators, giving 77/20. Finally, call the Reduce() method to reduce the result, restoring any whole number value so the fractional part of the number is less than 1. For example, 77/20 becomes 3 17/20. Include a function that returns a string that contains a Fraction in the usual display format—the whole number, a space, the numerator, a slash (D, and a denominator. When the whole number is 0, just the Fraction part of the value should be displayed (for example, 1/2 instead of 0 1/2). If the numerator is 0, just the whole number should be displayed (for example, 2 instead of 2 0/3). b. Add an operator*() method to the Fraction class created in Exercise 11a so that it correctly multiplies two Fractions. The result should be in proper, reduced format. Demonstrate that the method works correctly in a program named FractionDemo2. c. Write a program named FractionDem03 that includes an array of four Fractions. Prompt the user for values for each. Display every possible combination of addition results and every possible combination of multiplication results for each Fraction pair (that is, each type will have 16 results).arrow_forward
- At most, a class can contain ____________ method(S). 0 1 2 any number ofarrow_forwardIn Chapter 10, the class clockType was designed to implement the time of day in a program. Certain applications, in addition to hours, minutes, and seconds, might require you to store the time zone. Derive the class extclockType from the class clockType by adding a member variable to store the time zone. Add the necessary member functions and constructors to make the class functional. Also, write the definitions of the member functions and the constructors. Finally, write a test program to test your class.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
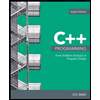
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
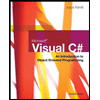
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
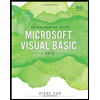
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
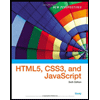
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
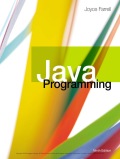
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT