ABC Bank announced a new scheme of reward points for a transaction using an ATM card. Each transaction using the normal card will be provided by giving a reward percentage of the transaction amount as a reward point. If a transaction is made using a premium card and it is for fuel expenses, additional 10 points will be rewarded. Write a program to calculate the total reward points. Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified in the problem statement. A class VISACard has the following attributes. Access Specifiers Data type Variable protected string type protected double amount private int rewardPercentage Include the following method in VISACard class. Method Description public void ComputeRewardPoints() In this method, calculate and display the reward points based on rewardPercentage in amount Formula: Reward points = amount * (rewardPercentage / 100) A derived class HPVISACard derived from VISACard class and overrides the following method. Method Description public void ComputeRewardPoints() In this method, calculate the reward point. 3% of the amount will be reward points. If the transaction type is fuel, add 10 points to calculate reward points. Formula: Transaction type (Fuel): Reward points = (amount * (3 / 100))+ 10 Type other than fuel: Reward points = amount * (3 / 100) In the Main method, obtain input from the user. Create VISACard or HPVISACard objects and invoke display methods to display the details. Card type will be either ‘VISA card’ or ‘HPVISA card’. Otherwise, display ‘Invalid input’ Calculate the reward points corresponding to the card type and transaction type and print the reward points(upto two precision). Input and Output Format: Refer sample input and output for formatting specifications. All text in bold corresponds to the input and the rest corresponds to output. Sample Input and Output 1 : Menu 1.VISA Card 2.HPVISA Card Enter the choice 1 Enter the transaction type Shopping Enter the transaction amount 5000 Enter the reward points 2 Card details: Type: Shopping Amount: 5000 Reward point: 100.0 Sample Input and Output 2 : Menu 1.VISA Card 2.HPVISA Card Enter the choice 2 Enter the transaction type Fuel Enter the transaction amount 650 Card details: Type: Fuel Amount: 650 Reward point: 29.5 Sample Input and Output 3 : Menu 1.VISA Card 2.HPVISA Card Enter the choice 3 Invalid input
ABC Bank announced a new scheme of reward points for a transaction using an ATM card. Each transaction using the normal card will be provided by giving a reward percentage of the transaction amount as a reward point. If a transaction is made using a premium card and it is for fuel expenses, additional 10 points will be rewarded.
Write a program to calculate the total reward points.
Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified in the problem statement.
A class VISACard has the following attributes.
Access Specifiers | Data type | Variable |
protected | string | type |
protected | double | amount |
private | int | rewardPercentage |
Include the following method in VISACard class.
Method | Description |
public void ComputeRewardPoints() | In this method, calculate and display the reward points based on rewardPercentage in amount |
Formula:
Reward points = amount * (rewardPercentage / 100)
A derived class HPVISACard derived from VISACard class and overrides the following method.
Method | Description |
public void ComputeRewardPoints() | In this method, calculate the reward point. 3% of the amount will be reward points. If the transaction type is fuel, add 10 points to calculate reward points. |
Formula:
Transaction type (Fuel): Reward points = (amount * (3 / 100))+ 10
Type other than fuel: Reward points = amount * (3 / 100)
In the Main method, obtain input from the user.
Create VISACard or HPVISACard objects and invoke display methods to display the details.
Card type will be either ‘VISA card’ or ‘HPVISA card’. Otherwise, display ‘Invalid input’
Calculate the reward points corresponding to the card type and transaction type and print the reward points(upto two precision).
Input and Output Format:
Refer sample input and output for formatting specifications.
All text in bold corresponds to the input and the rest corresponds to output.
Sample Input and Output 1 :
Menu
1.VISA Card
2.HPVISA Card
Enter the choice
1
Enter the transaction type
Shopping
Enter the transaction amount
5000
Enter the reward points
2
Card details:
Type: Shopping
Amount: 5000
Reward point: 100.0
Sample Input and Output 2 :
Menu
1.VISA Card
2.HPVISA Card
Enter the choice
2
Enter the transaction type
Fuel
Enter the transaction amount
650
Card details:
Type: Fuel
Amount: 650
Reward point: 29.5
Sample Input and Output 3 :
Menu
1.VISA Card
2.HPVISA Card
Enter the choice
3
Invalid input

Trending now
This is a popular solution!
Step by step
Solved in 7 steps with 3 images

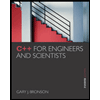
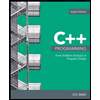
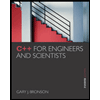
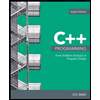