6.use c code to Develop a code that gets two integers from the user and calculates and prints their least common multiplier or LCM. LCM is the smallest number that divides both given integers. Example. lcm (10, 15) = 30, lcm (5,7) = 35 and lcm (12, 24) = 24 Hint: we know that lcm(x,y) = xy/gcd(x,y) where gcd is the greatest common divisor (discussed in class). Develop a gcd function in your code, get the two numbers from the user, call your function to calculate their gcd and then use the formula above to calculate and display their lcm GOOD LUCK! Reference This is a list of function prototypes of the C library functions presented in class. You may use any of these functions in your solutions (unless the requirements explicitly indicate otherwise). As you have been provided the function prototypes, you are expected to use the functions correctly in your solutions. double atof(char *string); int atoi(char *string); long atol(char *string); int fclose(FILE *filePointer); char *fgets(char *string, int n, FILE *filePointer); FILE *fopen(char *fileName, char *access); int fprintf(FILE *filePointer, char *format, ...); int fputs(char *string, FILE *filePointer); int fscanf(FILE *filePointer, char *format, ...); char *gets(char *ptrString); int printf(char *format, ...); int puts(char *ptrString); int scanf(char *format, ...); char *strcat(char *stringTo, char *stringFrom); int strcmp(char *string1, char *string2); char *strcpy(char *stringTo, char *stringFrom); int strlen(char *string); char *strncat(char *stringTo, char *stringFrom, int size); int strncmp(char *string1, char *string2, int size); char *strncpy(char *stringTo, char *stringFrom, int size); int tolower(char oldchar); int toupper(char oldchar); Hint: the answer should need to link together with a c code and output ASCII Table 32 40 ( 48 0 56 8 64 @ 72 H 80 P 88 X 96 ` 104 h 112 p 120 x 33 ! 41 ) 49 1 57 9 65 A 73 I 81 Q 89 Y 97 a 105 i 113 q 121 y 34 " 42 * 50 2 58 : 66 B 74 J 82 R 90 Z 98 b 106 j 114 r 122 z 35 # 43 + 51 3 59 ; 67 C 75 K 83 S 91 [ 99 c 107 k 115 s 123 { 36 $ 44 , 52 4 60 < 68 D 76 L 84 T 92 \ 100 d 108 l 116 t 124 | 37 % 45 - 53 5 61 = 69 E 77 M 85 U 93 ] 101 e 109 m 117 u 125 } 38 & 46 . 54 6 62 > 70 F 78 N 86 V 94 ^ 102 f 110 n 118 v 126 ~ 39 ' 47 / 55 7 63 ? 71 G 79 O 87 W 95 _ 103 g 111 o 119 w 127
6.use c code to Develop a code that gets two integers from the user and calculates and prints their least common multiplier or LCM. LCM is the smallest number that divides both given integers.
Example.
lcm (10, 15) = 30, lcm (5,7) = 35 and lcm (12, 24) = 24
Hint: we know that lcm(x,y) = xy/gcd(x,y) where gcd is the greatest common divisor (discussed in class). Develop a gcd function in your code, get the two numbers from the user, call your function to calculate their gcd and then use the formula above to calculate and display their lcm
GOOD LUCK!
Reference
This is a list of function prototypes of the C library functions presented in class. You may use any of these functions in your solutions (unless the requirements explicitly indicate otherwise). As you have been provided the function prototypes, you are expected to use the functions correctly in your solutions.
double atof(char *string);
int atoi(char *string);
long atol(char *string);
int fclose(FILE *filePointer);
char *fgets(char *string, int n, FILE *filePointer);
FILE *fopen(char *fileName, char *access);
int fprintf(FILE *filePointer, char *format, ...);
int fputs(char *string, FILE *filePointer);
int fscanf(FILE *filePointer, char *format, ...);
char *gets(char *ptrString);
int printf(char *format, ...);
int puts(char *ptrString);
int scanf(char *format, ...);
char *strcat(char *stringTo, char *stringFrom);
int strcmp(char *string1, char *string2);
char *strcpy(char *stringTo, char *stringFrom);
int strlen(char *string);
char *strncat(char *stringTo, char *stringFrom, int size);
int strncmp(char *string1, char *string2, int size);
char *strncpy(char *stringTo, char *stringFrom, int size);
int tolower(char oldchar);
int toupper(char oldchar);
Hint: the answer should need to link together with a c code and output
ASCII Table
32 40 ( 48 0 56 8 64 @ 72 H 80 P 88 X 96 ` 104 h 112 p 120 x
33 ! 41 ) 49 1 57 9 65 A 73 I 81 Q 89 Y 97 a 105 i 113 q 121 y
34 " 42 * 50 2 58 : 66 B 74 J 82 R 90 Z 98 b 106 j 114 r 122 z
35 # 43 + 51 3 59 ; 67 C 75 K 83 S 91 [ 99 c 107 k 115 s 123 {
36 $ 44 , 52 4 60 < 68 D 76 L 84 T 92 \ 100 d 108 l 116 t 124 |
37 % 45 - 53 5 61 = 69 E 77 M 85 U 93 ] 101 e 109 m 117 u 125 }
38 & 46 . 54 6 62 > 70 F 78 N 86 V 94 ^ 102 f 110 n 118 v 126 ~
39 ' 47 / 55 7 63 ? 71 G 79 O 87 W 95 _ 103 g 111 o 119 w 127

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 3 images

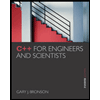
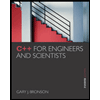