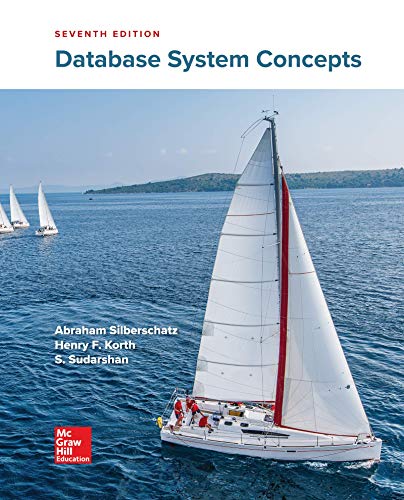
Please modify the C++ data structure program below to detect and display multiple candidates with the same number of votes: multiple winners, multiple candidates with the second highest number of votes, multiple candidates with the third highest number of votes, and so on.
Suppose the candidates are A, B, C, D, E, F and G and their votes are:
A 100
B 200
C 200
D 400
E 100
F 400
G 300
H 300
Then your program should display,
A Fourth Place
B Third Place
C Third Place
D First Place
E Fourth Place
F First Place
G Second Place
H Second Place
with the amount of votes they received, for example: A Fourth Place with 100 votes, B Third Place with 200 votes, C Third Place with 200 and etc. Text file names and program below. Thank you.
votes.txt |
A = Johnson |
B = Miller |
C = Robinson |
D = Duffy |
E = Asthon |
F = Sampson |
G = Adams |
H = Williams |
________________________________________________________________________________
#include <array>
#include <cassert>
#include <cmath>
#include <fstream>
#include <iomanip>
#include <iostream>
#include <string>
#include <vector>
using namespace std;
const string filename = "votes.txt";
const int maxCandidates = 8;
struct Candidate
{
string name = "";
size_t votes = 0;
double percent = 0.0;
};
int main()
{
ifstream inps(filename);
assert(inps);
array<Candidate, maxCandidates> candidates;
size_t nCandidates = 0;
while (inps.good() && nCandidates < maxCandidates)
{
string name;
inps >> name;
size_t votes;
inps >> votes;
candidates[nCandidates].name = name;
candidates[nCandidates].votes = votes;
nCandidates++;
}
if (nCandidates > 0)
{
size_t namefieldwidth = 12;
size_t maxvotes = 0;
size_t maxidx = 0;
size_t totalvotes = 0;
for (size_t idx = 0; idx < nCandidates; idx++)
{
totalvotes += candidates[idx].votes;
if (candidates[idx].votes > maxvotes)
{
maxvotes = candidates[idx].votes;
maxidx = idx;
}
}
size_t namefieldwith = 16;
size_t votesfieldwidth = 16;
size_t percentfieldwidth = 16;
if (totalvotes > 0)
{
for (size_t idx = 0; idx < nCandidates; idx++)
candidates[idx].percent = 100.0 * candidates[idx].votes / static_cast<double>(totalvotes);
}
cout << fixed << setprecision(2);
cout << left << setw(namefieldwidth) << "Candidate" << ' ' << right << setw(votesfieldwidth) << "Votes Received" << ' ' << right << setw(percentfieldwidth) << setfill(' ') << "% of Total Votes " << endl << endl;
for (size_t idx = 0; idx < nCandidates; ++idx)
cout << left << setw(namefieldwidth) << candidates[idx].name << ' ' << right << setw(votesfieldwidth) << candidates[idx].votes << ' ' << right << setw(percentfieldwidth) << setfill(' ') << candidates[idx].percent << endl;
size_t totalvotesfieldwidth = namefieldwidth + votesfieldwidth + 1;
cout << setw(totalvotesfieldwidth) << totalvotes << endl << endl;
cout << "The winner of the Election is " << candidates[maxidx].name << endl;
}
return 0;
}
/**
Implementation/Code change to display multiple winners
This way more than one candidate with same number of maximum votes can be displayed
**/
//Loops through every candidate
for (size_t idx = 0; idx < nCandidates; idx++)
{
//If the candidate has max votes
if (candidates[idx].votes == maxvotes)
{
//Display the candidate name
cout << candidates[idx].name << endl;
}
}
}
return 0;
}
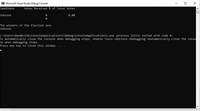

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- in C program For this assignment, you will need to build a program that does the following: The process of finding the largest value is used frequently in computer applications. For example, a program that determines the winner of a sales competition would input the number of units sold by each salesperson. The salesperson who sells the most units wins the contest. Write a program that has the user input a series of 10 integers and determine and print the largest integer. Your program should use at least the following three variables: counter: A counter to count to 10 (i.e., to keep track of how many numbers have been input and to determine when all 10 numbers have been processed). number: The integer most recently input by the user. largest: The largest number found so far. You must use a while loop as well as an if statement. Properly give the user instructions, take input and print the largest number before saying goodbye to the user. The program output will look something like…arrow_forwardIn C++, using the functions rand and srand write a complete main function that simulates a lottery and displays 6 random numbers in the range 1 to 60 horizontally with a space between them.(You do not need to test for duplicates).arrow_forwardC Programming Write a program that reads an integer from the user (keyboard), namely n. Yourprogram should print all positive numbers that are smaller than n and whosedigits sum up to a perfect square number.(An integer is a perfect square if it hasan integer square-root for example 25) You should print the numbers in ascendingorder and print their digits separated by “:“ symbol from the least significant digitto the most significant digit on the same line as the number. At the end of eachline you should also print the sum of the digits of the number. Example:If the user enters n : 30, then the output should be as follows:1 1 14 4 49 9 910 0:1 113 3:1 418 8:1 922 2:2 427 7:2 9arrow_forward
- Note: Please answer in C++ Programming language. During Eid, it is a tradition for every father to give his children money to spend on entertainment. Chef has N coins; denote the value of the coin i by vi. Since today is Eid, Chef gives one coin to each of his two children. He wants the absolute value of the difference between the values of the coins given to the two children to be as small as possible to be as fair as possible. Help the chef by telling him the minimum possible difference between the values of the coins given to the two children. Of course, the chef cannot give the same coin to both children. Possible input 1 3 142 Required Output 1arrow_forwardQn1: You are asked to write a simple C program that will accept an integer value in the range of 5-95 and as a multiple of 5 representing the number of cents to give to a customer in their change. The program should calculate how many coins of each denomination and display this to the user. Valid coin values are 50, 20, 10 and 5. Your solution (program and algorithm) should be modular in nature. This requires the submission of a high-level algorithm and suitable decompositions of each step. Note that for this problem the principle of code reuse is particularly important and a significant number of marks are allocated to this. You should attempt to design your solution such that it consists of a relatively small number of functions that are as general in design as possible and you should have one function in particular that can be reused (called repeatedly) in order to solve the majority of the problem. If you find that you have developed a large number of functions which each performs…arrow_forwardPlease modify the C++ data structure program below to detect and display multiple candidates with the same number of votes: multiple winners, multiple candidates with the second highest number of votes, multiple candidates with the third highest number of votes, and so on. Suppose the candidates are A, B, C, D, E, F and G and their votes are: A 100 B 200 C 200 D 400 E 100 F 400 G 300 H 300 Then your program should display, A Fourth Place B Third Place C Third Place D First Place E Fourth Place F First Place G Second Place H Second Place with the amount of votes they received, for example: A Fourth Place with 100 votes, B Third Place with 200 votes, C Third Place with 200 and etc. Text file names and program below. Thank you. votes.txt Johnson Miller Robinson Duffy Asthon Sampson Adams Williams ________________________________________________________________________________ #include <array> #include <cassert> #include <cmath> #include…arrow_forward
- in C++arrow_forwardNote: Please answer in C++ Programming language. During Eid, it is a tradition for every father to give his children money to spend on entertainment. Chef has N coins; denote the value of the coin i by vi. Since today is Eid, Chef gives one coin to each of his two children. He wants the absolute value of the difference between the values of the coins given to the two children to be as small as possible to be as fair as possible. Help the chef by telling him the minimum possible difference between the values of the coins given to the two children. Of course, the chef cannot give the same coin to both children. Possible input 1 3 142 Required Output 1arrow_forwardIn c++ you have 5 different countries, Each country contains two different integers. Example England(30, 10) in that format, how will you calculate and find the country with the smallest integers between those 5 countries and also calculate and display the total steps that country has compared to the other countries. Example…England has 50 steps.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
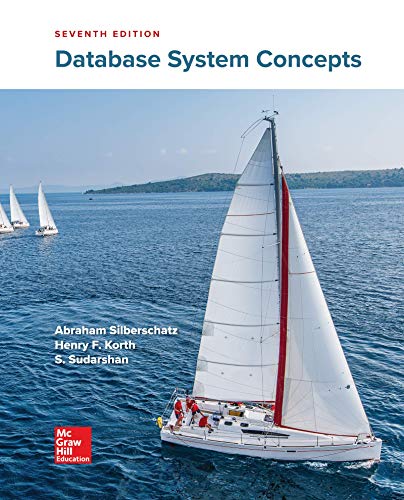
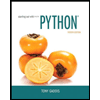
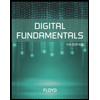
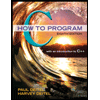
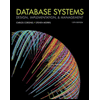
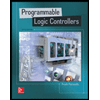