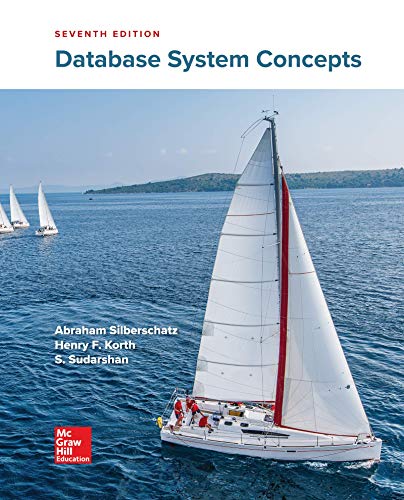
Modify the below program that the input values representing
fractions are stored with denominators that are positive integers. You cannot require the user to only enter a positive denominator value; the user should not be inconvenienced by such a restriction. For example, whilst values of 1 / -2 are acceptable inputs for a fraction, the output representation should be -1 / 2. Your solution should check the denominator input; if it is negative, swap the sign of both numerator and denominator instance variables.
//Import the essential package
import java.util.ArrayList;
import java.util.Scanner;
//Define the class Fraction
class Fraction
{
private int n, d;
public Fraction()
{
//Initialize the values
this.n = this.d = 0;
}
public Fraction(int n, int d)
{
//Initialize the variables
this.n = n;
this.d = d;
}
//Define the getter function getNum() that returns the numerator
public int getNum()
{
//Returns numerator
return n;
}
//Define the getter function getDen() that returns the denominator
public int getDen()
{
//Returns denominator
return d;
}
//Define the boolean function isZero() that returns 0 if numerator is 0 and denominator is not equals to zero
public boolean isZero()
{
return (getNum() == 0 && getDen() != 0);
}
//Define the function getSimplifiedFraction() that returns the simplified fraction
public String getSimplifiedFraction()
{
//Decalre the string variable result to store the result
String result = "";
//if the numerator and denominator both are zero
if(getNum() == 0 && getDen() == 0)
//result is zero
result = "0";
//if the numerator is zero and denominator is non-zero
else if(isZero())
//result is zero
result = "0";
//if the numerator is non-zero and denominator is zero
else if(getNum() != 0 && getDen() == 0)
//result is Undefined
result = "Undefined";
//for a defined and non-zero fraction
else
{
//if the remainder is zero
if(getNum() % getDen() == 0)
result = (getNum() / getDen()) + "";
//if the numerator and denominator both are greater than zero
else if(getNum() < 0 && getDen() < 0)
result = (getNum() * -1) + "/" + (getDen() * -1);
//if any of them is zero
else if(getNum() < 0 || getDen() < 0)
{
//if the numerator is greater than zero
if(getNum() < 0)
result = "- (" + (getNum() * -1) + "/" + getDen() + ")";
//if the denominator is zero
else
result = "- (" + getNum() + "/" + (getDen() * -1) + ")";
}
//both are non-zero
else
result = (getNum() + "/" + getDen());
}
//return the result
return result;
}
//Define the method display() that displays the resultant fraction
public void display()
{
//Call and display the fraction
System.out.println(getSimplifiedFraction());
}
}
//Define the class TestFraction
class TestFraction
{
//Define the main method
public static void main(String[] args)
{
//Declare the variables to store the numerator and denominator
int num, den;
//Declare an ArrayList to store the results
ArrayList<Fraction> fractions = new ArrayList<>();
//Create the object for scanner class
Scanner sc = new Scanner(System.in);
//use the do-while loop to iterate test the conditions
do
{
//Prompts the user to enter the numerator
System.out.print("Enter the num: ");
//Store the Integer part only
num = Integer.parseInt(sc.nextLine().trim());
//If the numerator is less than zero
if(num < 0)
//Break from loop
break;
//Prompts the user to enter the denominator
System.out.print("Enter the den: ");
//store the integer part only
den = Integer.parseInt(sc.nextLine().trim());
//Create the object of Fraction class
Fraction fraction = new Fraction(num, den);
//Add the resultant fractions into the ArrayList
fractions.add(fraction);
//Display the resultant fraction
System.out.println("Fraction added to list as: " + fraction.getSimplifiedFraction() + "\n");
}while(num >= 0);
//Display all fractions that are stored in the ArrayList
System.out.println("\nDISPLAYING ALL FRACTIONS:\n"
+ "-------------------------");
//Use the loop to display the values stored in the ArrayList
for(Fraction fr : fractions)
fr.display();
//to print the new line
System.out.println();
}
}

Step by stepSolved in 4 steps with 9 images

- When the robot makes a turn, it would be useful to have an operation to perform on d to represent this turn. This is because after making a turn, the new value of d will depend on the old value of d. Complete the table for the new values of d if the robot is turning left or right.Then determine an expression in terms of d that will give the new position if the robot turns left and another expression if the robot turns right. Type these expressions in the last row of the table. Attached is the table I completed. All rows are correct except the last one. I'm not sure what my error is.arrow_forwardPlease code in OOP C++, show example output to see if no errorsCrossword Puzzles An unlabeled crossword is given to you as an N by M grid (3 <= N <= 50, 3 <= M <= 50). Some cells will be clear (typically colored white) and some cells will be blocked (typically colored black). Given this layout, clue numbering is a simple process which follows two logical Steps: Step 1: We determine if a each cell begins a horizontal or vertical clue. If a cell begins a horizontal clue, it must be clear, its neighboring cell to the left must be blocked or outside the crossword grid, and the two cells on its right must be clear (that is, a horizontal clue can only represent a word of 3 or more characters). The rules for a cell beginning a vertical clue are analogous: the cell above must be blocked or outside the grid, and the two cells below must be clear. Step 2: We assign a number to each cell that begins a clue. Cells are assigned numbers sequentially starting with 1 in the same order that…arrow_forwardWord Statistics: The second requirement change is to allow replacement of all occurrences of a given word to a given replacemWrite the code only in python language with the opeartion exactly and necessary comments to be added.arrow_forward
- Code in Python Write a function, print_perfect_cubes(n), that takes an integer parameter n and prints the perfect cubes starting from 03 = 0 and ending with n3. When n is negative, the function prints nothing; but do not check for this condition with an "if" statement, it is unneccesary; use a for loop that will automatically print nothing when n < 0. Hint for choosing the right range for your for loop: In the general case, when n is not negative, your function will need to print n+1 different values. For example: Test Result print_perfect_cubes(2) 0 1 8 print_perfect_cubes(5) 0 1 8 27 64 125 print_perfect_cubes(-10)arrow_forwardPython Language] Using loops of any kind, lists, or is not allowed. At the Academy of Crime Fighting, each trainee is recognized by a unique 5-digit identifier. The identifier can have any of the following digits: 0, 1, 2, 3, 4, 5, 6, 7, 8, 9. The identifier can have leading 0s. Since Drew the owl has trouble typing numbers, the school makes things easier by ensuring that each successive digit in the identifier is either one digit greater or one digit less than the previous digit. For example, if the first digit is 3, then the successive digit would be 4 or 2. So, 32345 would be a valid trainee identifier, but 32435 would NOT be a valid identifier because the third digit 4 is two digits away from the previous digit 2. Your task is to write a program that validates an identifier. Input The input consists of five lines; the first line is the first digit of the trainee identifier, the second line is the second digit of the trainee identifier, and so on. Output If the trainee identifier is…arrow_forwardWrite a program that paints the 4 areas inside the mxm square as follow: Upper left: black. RGB (0, 0, 0) Upper right: white. RGB (255, 255, 255) Lower left: red. RGB (255, 0, 0) Lower right: random colors Random colors Hint: Define a function that paints one of the four squares. Call the function 4 times to complete the painting. (painting means setting the pixels)arrow_forward
- Description A mathmatician Goldbach's conjecture: any even number(larger than 2) can divide into two prime number’s sum.But some even numbers can divide into many pairs of two prime numbers’ sum. Example:10 =3+7, 10=5+5, 10 can divide into two pairs of two prime number. Input Input consist a positive even number n(4<=n<=32766). Output Print the value of how many pairs are this even number can be divided into. Sample Input 1 1234 Sample Output 1 25arrow_forwardWritten in Python as well as screenshotted accepting user input and computed valuesarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
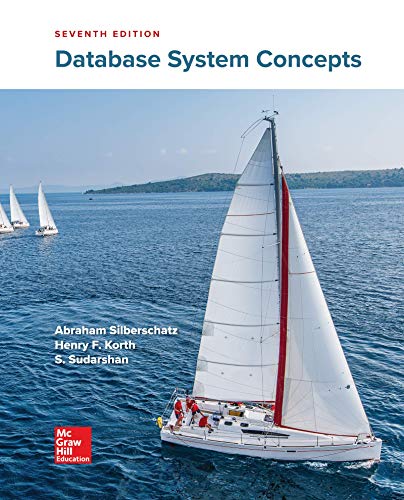
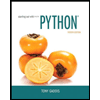
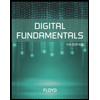
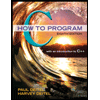
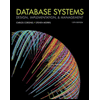
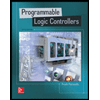